|
| 1 | +# 1420. Build Array Where You Can Find The Maximum Exactly K Comparisons |
| 2 | + |
| 3 | +- Difficulty: Hard. |
| 4 | +- Related Topics: Dynamic Programming, Prefix Sum. |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +You are given three integers `n`, `m` and `k`. Consider the following algorithm to find the maximum element of an array of positive integers: |
| 10 | + |
| 11 | +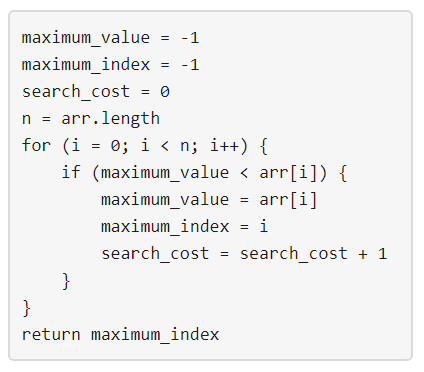 |
| 12 | + |
| 13 | +You should build the array arr which has the following properties: |
| 14 | + |
| 15 | + |
| 16 | + |
| 17 | +- `arr` has exactly `n` integers. |
| 18 | + |
| 19 | +- `1 <= arr[i] <= m` where `(0 <= i < n)`. |
| 20 | + |
| 21 | +- After applying the mentioned algorithm to `arr`, the value `search_cost` is equal to `k`. |
| 22 | + |
| 23 | + |
| 24 | +Return **the number of ways** to build the array `arr` under the mentioned conditions. As the answer may grow large, the answer **must be** computed modulo `109 + 7`. |
| 25 | + |
| 26 | + |
| 27 | +Example 1: |
| 28 | + |
| 29 | +``` |
| 30 | +Input: n = 2, m = 3, k = 1 |
| 31 | +Output: 6 |
| 32 | +Explanation: The possible arrays are [1, 1], [2, 1], [2, 2], [3, 1], [3, 2] [3, 3] |
| 33 | +``` |
| 34 | + |
| 35 | +Example 2: |
| 36 | + |
| 37 | +``` |
| 38 | +Input: n = 5, m = 2, k = 3 |
| 39 | +Output: 0 |
| 40 | +Explanation: There are no possible arrays that satisify the mentioned conditions. |
| 41 | +``` |
| 42 | + |
| 43 | +Example 3: |
| 44 | + |
| 45 | +``` |
| 46 | +Input: n = 9, m = 1, k = 1 |
| 47 | +Output: 1 |
| 48 | +Explanation: The only possible array is [1, 1, 1, 1, 1, 1, 1, 1, 1] |
| 49 | +``` |
| 50 | + |
| 51 | + |
| 52 | +**Constraints:** |
| 53 | + |
| 54 | + |
| 55 | + |
| 56 | +- `1 <= n <= 50` |
| 57 | + |
| 58 | +- `1 <= m <= 100` |
| 59 | + |
| 60 | +- `0 <= k <= n` |
| 61 | + |
| 62 | + |
| 63 | + |
| 64 | +## Solution |
| 65 | + |
| 66 | +```javascript |
| 67 | +/** |
| 68 | + * @param {number} n |
| 69 | + * @param {number} m |
| 70 | + * @param {number} k |
| 71 | + * @return {number} |
| 72 | + */ |
| 73 | +var numOfArrays = function(n, m, k) { |
| 74 | + return helper(n, m, k, 0, {}); |
| 75 | +}; |
| 76 | + |
| 77 | +var helper = function (n, m, k, maxSoFar, dp) { |
| 78 | + if (n === 0 && k === 0) return 1; |
| 79 | + if (n === 0) return 0; |
| 80 | + if (maxSoFar === m && k > 0) return 0; |
| 81 | + var key = `${n}-${k}-${maxSoFar}`; |
| 82 | + if (dp[key] !== undefined) { |
| 83 | + return dp[key]; |
| 84 | + } |
| 85 | + var mod = Math.pow(10, 9) + 7; |
| 86 | + var ans = 0; |
| 87 | + // choose num less than the current max value |
| 88 | + for (var i = 1; i <= maxSoFar; i++) { |
| 89 | + ans = (ans + helper(n - 1, m, k, maxSoFar, dp)) % mod; |
| 90 | + } |
| 91 | + // choose num bigger than the current max value |
| 92 | + for (var j = maxSoFar + 1; j <= m; j++) { |
| 93 | + ans = (ans + helper(n - 1, m, k - 1, j, dp)) % mod; |
| 94 | + } |
| 95 | + dp[key] = ans; |
| 96 | + return dp[key]; |
| 97 | +}; |
| 98 | +``` |
| 99 | + |
| 100 | +**Explain:** |
| 101 | + |
| 102 | +nope. |
| 103 | + |
| 104 | +**Complexity:** |
| 105 | + |
| 106 | +* Time complexity : O(n * m ^ 2 * k). |
| 107 | +* Space complexity : O(n * m * k). |
0 commit comments