diff --git a/.github/workflows/docker-cd.yml b/.github/workflows/docker-cd.yml
new file mode 100644
index 00000000..e1ed4155
--- /dev/null
+++ b/.github/workflows/docker-cd.yml
@@ -0,0 +1,41 @@
+name: Docker CD
+
+on:
+ push:
+ branches:
+ - master
+ tags:
+ - '*'
+ paths-ignore:
+ - '**.md'
+
+jobs:
+ build:
+
+ runs-on: ubuntu-latest
+
+ steps:
+
+ - name: checkout code
+ uses: actions/checkout@v2
+
+ - name: Log in to ghcr
+ uses: docker/login-action@v1
+ with:
+ registry: ghcr.io
+ username: ${{ github.actor }}
+ password: ${{ secrets.GITHUB_TOKEN }}
+
+ - if: startsWith(github.ref, 'refs/heads/master')
+ run: echo "TAG=latest" >> $GITHUB_ENV
+
+ - if: startsWith(github.ref, 'refs/tags')
+ run: echo "TAG=$(git describe --tags)" >> $GITHUB_ENV
+
+ - name: Build & Push
+ uses: docker/build-push-action@v2
+ with:
+ context: ./build
+ file: ./build/Dockerfile
+ push: true
+ tags: ghcr.io/${{ github.repository }}:${{ env.TAG }}
\ No newline at end of file
diff --git a/.github/workflows/main.yml b/.github/workflows/main.yml
new file mode 100644
index 00000000..1072bdeb
--- /dev/null
+++ b/.github/workflows/main.yml
@@ -0,0 +1,62 @@
+name: CI
+
+on: [push, pull_request]
+
+jobs:
+ build-linux:
+ runs-on: ubuntu-latest
+
+ steps:
+ - uses: actions/checkout@v3
+ - name: Install .Net Core
+ uses: actions/setup-dotnet@v3
+ with:
+ dotnet-version: |
+ 8.0.x
+ 9.0.x
+ include-prerelease: true
+ - name: Install dotnet-script
+ run: dotnet tool install dotnet-script --global
+
+ - name: Run build script
+ run: dotnet-script build/Build.csx
+
+ build-mac:
+ runs-on: macos-13
+
+ steps:
+ - uses: actions/checkout@v3
+ - name: Install .Net Core
+ uses: actions/setup-dotnet@v3
+ with:
+ dotnet-version: |
+ 8.0.x
+ 9.0.x
+ include-prerelease: true
+ - name: Install dotnet-script
+ run: dotnet tool install dotnet-script --global
+
+ - name: Run build script
+ run: dotnet-script build/Build.csx
+
+ build-windows:
+ runs-on: windows-latest
+
+ steps:
+ - uses: actions/checkout@v3
+ - name: Install .Net Core
+ uses: actions/setup-dotnet@v3
+ with:
+ dotnet-version: |
+ 8.0.x
+ 9.0.x
+ include-prerelease: true
+ - name: Install dotnet-script
+ run: dotnet tool install dotnet-script --global
+
+ - name: Run build script
+ run: dotnet-script build/Build.csx
+ env: # Or as an environment variable
+ GITHUB_REPO_TOKEN: ${{ secrets.GITHUB_TOKEN }}
+ IS_SECURE_BUILDENVIRONMENT: ${{ secrets.IS_SECURE_BUILDENVIRONMENT }}
+ NUGET_APIKEY: ${{ secrets.NUGET_APIKEY }}
diff --git a/.gitignore b/.gitignore
index e9ec90a2..62f574c6 100644
--- a/.gitignore
+++ b/.gitignore
@@ -265,3 +265,5 @@ project.json
/build/dotnet-script
/dotnet-script
/.vscode
+/src/Dotnet.Script/Properties/launchSettings.json
+.DS_Store
diff --git a/README.md b/README.md
index 5d621cd0..3482c176 100644
--- a/README.md
+++ b/README.md
@@ -8,23 +8,26 @@ Run C# scripts from the .NET CLI, define NuGet packages inline and edit/debug th
## NuGet Packages
-| Name | Version | Framework(s) |
-| ------------------------------------- | ------------------------------------------------------------ | -------------------------------- |
-| `dotnet-script` | [](https://www.nuget.org/packages/dotnet-script/) | `netcoreapp2.1`, `netcoreapp3.1` |
-| `Dotnet.Script` | [](https://www.nuget.org/packages/dotnet.script/) | `netcoreapp2.1`, `netcoreapp3.1` |
-| `Dotnet.Script.Core` | [](https://www.nuget.org/packages/Dotnet.Script.Core/) | `netstandard2.0` |
-| `Dotnet.Script.DependencyModel` | [](https://www.nuget.org/packages/Dotnet.Script.DependencyModel/) | `netstandard2.0` |
-| `Dotnet.Script.DependencyModel.Nuget` | [](https://www.nuget.org/packages/Dotnet.Script.DependencyModel.Nuget/) | `netstandard2.0` |
+| Name | Version | Framework(s) |
+| ------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------- | ---------------------------------- |
+| `dotnet-script` (global tool) | [](https://www.nuget.org/packages/dotnet-script/) | `net8.0`,`net9.0` |
+| `Dotnet.Script` (CLI as Nuget) | [](https://www.nuget.org/packages/dotnet.script/) | `net8.0`,`net9.0` |
+| `Dotnet.Script.Core` | [](https://www.nuget.org/packages/Dotnet.Script.Core/) | `net8.0`,`net9.0`,`netstandard2.0` |
+| `Dotnet.Script.DependencyModel` | [](https://www.nuget.org/packages/Dotnet.Script.DependencyModel/) | `netstandard2.0` |
+| `Dotnet.Script.DependencyModel.Nuget` | [](https://www.nuget.org/packages/Dotnet.Script.DependencyModel.Nuget/) | `netstandard2.0` |
## Installing
### Prerequisites
-The only thing we need to install is [.NET Core 2.1+ SDK](https://www.microsoft.com/net/download/core). In order to use C# 8.0 features, [.NET Core 3.1+ SDK](https://www.microsoft.com/net/download/core) must be installed.
+The only thing we need to install is [.NET 8.0 or .NET 9.0 SDK](https://dotnet.microsoft.com/en-us/download/dotnet).
+
+[Note](https://learn.microsoft.com/en-us/dotnet/core/install/linux-scripted-manual#manual-install):
+> If you install the .NET SDK to a non-default location, you need to set the environment variable `DOTNET_ROOT` to the directory that contains the dotnet executable
### .NET Core Global Tool
-.NET Core 2.1 introduces the concept of global tools meaning that you can install `dotnet-script` using nothing but the .NET CLI.
+.NET Core 2.1 introduced the concept of global tools meaning that you can install `dotnet-script` using nothing but the .NET CLI.
```shell
dotnet tool install -g dotnet-script
@@ -34,9 +37,6 @@ Tool 'dotnet-script' (version '0.22.0') was successfully installed.
```
The advantage of this approach is that you can use the same command for installation across all platforms.
-
-> ⚠️ In order to use the global tool you need [.NET Core SDK 2.1.300](https://www.microsoft.com/net/download/dotnet-core/sdk-2.1.300) or higher. The earlier previews and release candidates of .NET Core 2.1 are not supported.
-
.NET Core SDK also supports viewing a list of installed tools and their uninstallation.
```shell
@@ -55,26 +55,22 @@ Tool 'dotnet-script' (version '0.22.0') was successfully uninstalled.
### Windows
-```powershell
-choco install dotnet.script
-```
-
-We also provide a PowerShell script for installation.
+PowerShell script for installation.
```powershell
-(new-object Net.WebClient).DownloadString("https://raw.githubusercontent.com/filipw/dotnet-script/master/install/install.ps1") | iex
+(new-object Net.WebClient).DownloadString("https://raw.githubusercontent.com/dotnet-script/dotnet-script/master/install/install.ps1") | iex
```
### Linux and Mac
```shell
-curl -s https://raw.githubusercontent.com/filipw/dotnet-script/master/install/install.sh | bash
+curl -s https://raw.githubusercontent.com/dotnet-script/dotnet-script/master/install/install.sh | bash
```
If permission is denied we can try with `sudo`
```shell
-curl -s https://raw.githubusercontent.com/filipw/dotnet-script/master/install/install.sh | sudo bash
+curl -s https://raw.githubusercontent.com/dotnet-script/dotnet-script/master/install/install.sh | sudo bash
```
### Docker
@@ -94,7 +90,7 @@ docker run -it dotnet-script --version
### Github
-You can manually download all the releases in `zip` format from the [GitHub releases page](https://github.com/filipw/dotnet-script/releases).
+You can manually download all the releases in `zip` format from the [GitHub releases page](https://github.com/dotnet-script/dotnet-script/releases).
## Usage
@@ -110,6 +106,19 @@ That is all it takes and we can execute the script. Args are accessible via the
dotnet script helloworld.csx
```
+The following namespaces are available in the script implicitly and do not need to be imported explicitly:
+
+ - System
+ - System.IO
+ - System.Collections.Generic
+ - System.Console
+ - System.Diagnostics
+ - System.Dynamic
+ - System.Linq
+ - System.Linq.Expressions
+ - System.Text
+ - System.Threading.Tasks
+
### Scaffolding
Simply create a folder somewhere on your system and issue the following command.
@@ -148,7 +157,14 @@ Instead of `main.csx` which is the default, we now have a file named `custom.csx
### Running scripts
-Scripts can be executed directly from the shell as if they were executables.
+You can execute your script using **dotnet script** or **dotnet-script**.
+
+```bash
+dotnet script foo.csx
+dotnet-script foo.csx
+```
+
+On OSX/Linux, scripts can be executed directly from a shell as if they were executables.
```bash
foo.csx arg1 arg2 arg3
@@ -167,7 +183,9 @@ The OSX/Linux shebang directive should be **#!/usr/bin/env dotnet-script**
Console.WriteLine("Hello world");
```
-You can execute your script using **dotnet script** or **dotnet-script**, which allows you to pass arguments to control your script execution more.
+On Windows, you can run `dotnet script register` to achieve a similar behaviour. This registers dotnet-script in the Windows registry as the tool to process .csx files.
+
+You can pass arguments to control your script execution more.
```bash
foo.csx arg1 arg2 arg3
@@ -316,7 +334,6 @@ To consume a script package all we need to do specify the NuGet package in the `
The following example loads the [simple-targets](https://www.nuget.org/packages/simple-targets-csx) package that contains script files to be included in our script.
```C#
-#! "netcoreapp2.1"
#load "nuget:simple-targets-csx, 6.0.0"
using static SimpleTargets;
@@ -466,7 +483,6 @@ The following example shows how we can pipe data in and out of a script.
The `UpperCase.csx` script simply converts the standard input to upper case and writes it back out to standard output.
```csharp
-#! "netcoreapp2.1"
using (var streamReader = new StreamReader(Console.OpenStandardInput()))
{
Write(streamReader.ReadToEnd().ToUpper());
@@ -565,6 +581,24 @@ We will also see this when working with scripts in VS Code under the problems pa
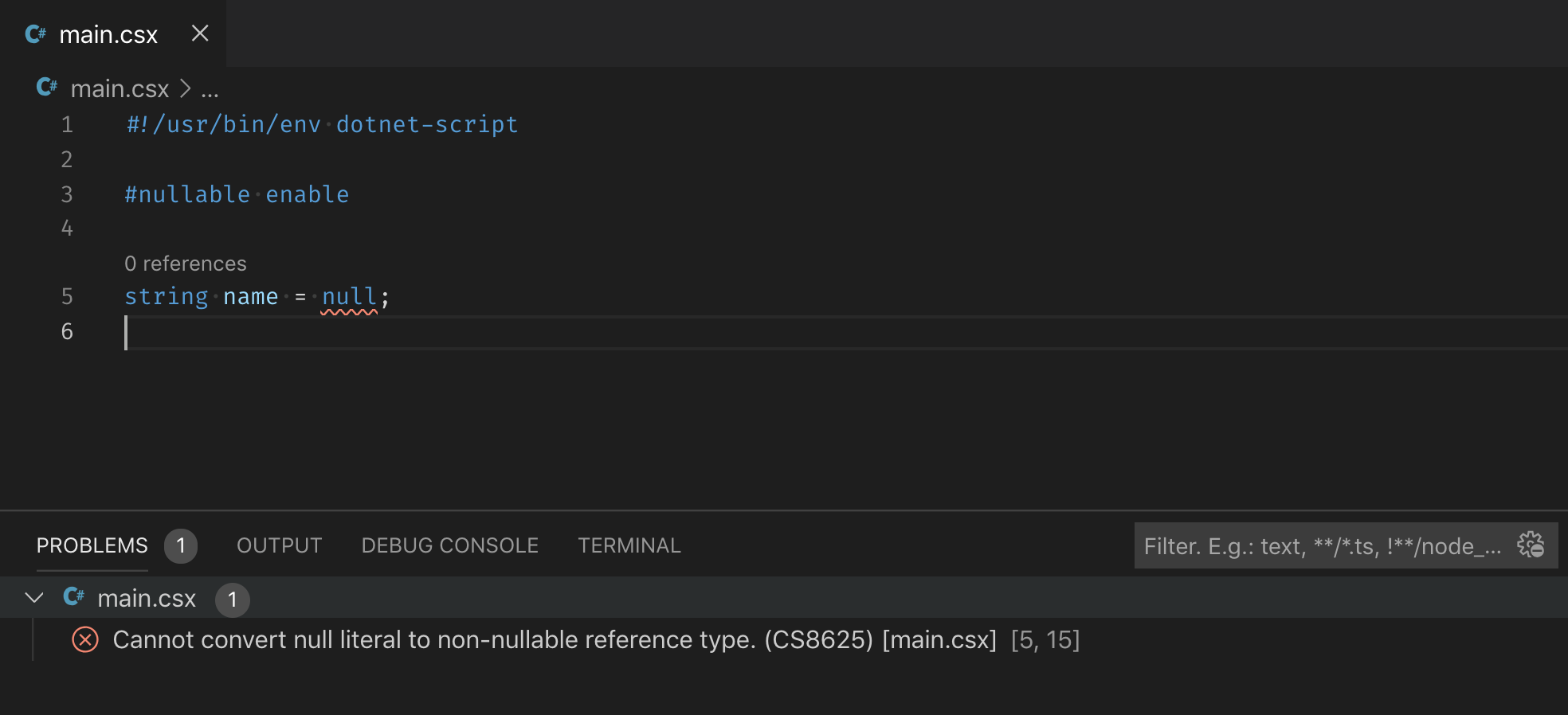
+## Specifying an SDK
+
+Starting with `dotnet-script` 1.4.0 we can now specify the SDK to be used for a script.
+
+For instance, creating a web server in a script is now as simple as the following.
+
+```csharp
+#r "sdk:Microsoft.NET.Sdk.Web"
+
+using Microsoft.AspNetCore.Builder;
+
+var a = WebApplication.Create();
+a.MapGet("/", () => "Hello world");
+a.Run();
+```
+
+> Please note the the only SDK currently supported is `Microsoft.NET.Sdk.Web`
+
## Team
- [Bernhard Richter](https://github.com/seesharper) ([@bernhardrichter](https://twitter.com/bernhardrichter))
@@ -572,4 +606,5 @@ We will also see this when working with scripts in VS Code under the problems pa
## License
-[MIT License](https://github.com/filipw/dotnet-script/blob/master/LICENSE)
+[MIT License](https://github.com/dotnet-script/dotnet-script/blob/master/LICENSE)
+ -
diff --git a/azure-pipelines.yml b/azure-pipelines.yml
deleted file mode 100644
index 6e47aa6e..00000000
--- a/azure-pipelines.yml
+++ /dev/null
@@ -1,122 +0,0 @@
-resources:
- - repo: self
-
-trigger:
- tags:
- include:
- - refs/tags/*
- branches:
- include:
- - "*"
-
-variables:
- - group: dotnet-script-api-keys
-
-jobs:
- - job: Job_3
- displayName: Ubuntu Agent
- condition: succeeded()
- pool:
- name: Hosted Ubuntu 1604
- steps:
- - bash: "curl -sSL https://dot.net/v1/dotnet-install.sh | bash /dev/stdin -version 3.1.102"
- displayName: "Install 3.0.100"
-
- - bash: "curl -sSL https://dot.net/v1/dotnet-install.sh | bash /dev/stdin -version 2.1.402"
- displayName: "Install 2.1.402"
-
- - bash: "curl -sSL https://dot.net/v1/dotnet-install.sh | bash /dev/stdin -version 5.0.100"
- displayName: " 5.0.100"
-
- - bash: |
- export PATH=/home/vsts/.dotnet:$PATH
- curl -L https://github.com/filipw/dotnet-script/releases/download/0.28.0/dotnet-script.0.28.0.zip > dotnet-script.zip
- unzip -o dotnet-script.zip -d ./
- displayName: "Install dotnet-script"
-
- - bash: |
- export PATH=/home/vsts/.dotnet:$PATH
- dotnet dotnet-script/dotnet-script.dll build/Build.csx
- displayName: "Run build.csx"
-
- - job: Job_1
- displayName: Mac Agent
- condition: succeeded()
- pool:
- name: Hosted macOS
- steps:
- - bash: |
- curl -sSL https://dot.net/v1/dotnet-install.sh | bash /dev/stdin -version 3.1.102
- displayName: "Install 3.0.100"
-
- - bash: |
- curl -sSL https://dot.net/v1/dotnet-install.sh | bash /dev/stdin -version 5.0.100
- displayName: "Install 5.0.100"
-
- - bash: |
- curl -L https://github.com/filipw/dotnet-script/releases/download/0.28.0/dotnet-script.0.28.0.zip > dotnet-script.zip
- unzip -o dotnet-script.zip -d ./
- displayName: "Install dotnet-script"
-
- - bash: "dotnet dotnet-script/dotnet-script.dll build/build.csx"
- displayName: "Run build.csx"
-
- - job: Job_2
- displayName: Windows Agent
- condition: succeeded()
- pool:
- name: Hosted Windows 2019 with VS2019
- steps:
- - powershell: |
- iwr https://raw.githubusercontent.com/dotnet/cli/release/2.1.3xx/scripts/obtain/dotnet-install.ps1 -outfile dotnet-install.ps1
- .\dotnet-install.ps1 -Version 3.1.102
-
- displayName: "Install 3.0.100"
-
- - powershell: |
- iwr https://raw.githubusercontent.com/dotnet/cli/release/2.1.3xx/scripts/obtain/dotnet-install.ps1 -outfile dotnet-install.ps1
- .\dotnet-install.ps1 -Version 2.1.402
-
- displayName: "Install 2.1.402 SDK"
-
- - powershell: |
- iwr https://raw.githubusercontent.com/dotnet/cli/release/2.1.3xx/scripts/obtain/dotnet-install.ps1 -outfile dotnet-install.ps1
- .\dotnet-install.ps1 -Version 5.0.100
-
- displayName: "Install 5.0.100"
-
- # NuGet Tool Installer
- # Acquires a specific version of NuGet from the internet or the tools cache and adds it to the PATH. Use this task to change the version of NuGet used in the NuGet tasks.
- - task: NuGetToolInstaller@0
- inputs:
- versionSpec: "4.8.2"
-
- #checkLatest: false # Optional
- - bash: |
- export PATH=/c/Users/VssAdministrator/AppData/Local/Microsoft/dotnet:$PATH
- dotnet --info
-
- displayName: "Show installed sdk"
-
- - bash: |
- export PATH=/c/Users/VssAdministrator/AppData/Local/Microsoft/dotnet:$PATH
- cd build
- curl -L https://github.com/filipw/dotnet-script/releases/download/0.28.0/dotnet-script.0.28.0.zip > dotnet-script.zip
- unzip -o dotnet-script.zip -d ./
- displayName: "Install dotnet-script"
-
- - bash: |
- export PATH=/c/Users/VssAdministrator/AppData/Local/Microsoft/dotnet:$PATH
- cd build
- dotnet dotnet-script/dotnet-script.dll build.csx
- displayName: "Run build.csx"
- env:
- IS_SECURE_BUILDENVIRONMENT: $(IS_SECURE_BUILDENVIRONMENT)
- GITHUB_REPO_TOKEN: $(GITHUB_REPO_TOKEN)
- NUGET_APIKEY: $(NUGET_APIKEY)
- CHOCOLATEY_APIKEY: $(CHOCOLATEY_APIKEY)
-
- - task: PublishPipelineArtifact@0
- displayName: "Publish Pipeline Artifact"
- inputs:
- targetPath: build/Artifacts
diff --git a/build.sh b/build.sh
index 8c3298d2..8af51e4a 100755
--- a/build.sh
+++ b/build.sh
@@ -3,9 +3,9 @@
SCRIPT_DIR=$( cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd )
DOTNET_SCRIPT="$SCRIPT_DIR/build/dotnet-script"
if [ ! -d "$DOTNET_SCRIPT" ]; then
- currentVersion=$(curl https://api.github.com/repos/filipw/dotnet-script/releases/latest?access_token=3a5de576bd32ddfccb52662d2d08d33a7edc318b | grep -Eo "\"tag_name\":\s*\"(.*)\"" | cut -d'"' -f4)
+ currentVersion=$(curl https://api.github.com/repos/dotnet-script/dotnet-script/releases/latest?access_token=3a5de576bd32ddfccb52662d2d08d33a7edc318b | grep -Eo "\"tag_name\":\s*\"(.*)\"" | cut -d'"' -f4)
echo "Downloading dotnet-script version $currentVersion..."
- curl -L https://github.com/filipw/dotnet-script/releases/download/$currentVersion/dotnet-script.$currentVersion.zip > "$SCRIPT_DIR/build/dotnet-script.zip"
+ curl -L https://github.com/dotnet-script/dotnet-script/releases/download/$currentVersion/dotnet-script.$currentVersion.zip > "$SCRIPT_DIR/build/dotnet-script.zip"
unzip -o "$SCRIPT_DIR/build/dotnet-script.zip" -d "$SCRIPT_DIR/build/"
if [ $? -ne 0 ]; then
echo "An error occured while downloading dotnet-script"
diff --git a/build/Build.csx b/build/Build.csx
index 02ee632c..3aa8a709 100644
--- a/build/Build.csx
+++ b/build/Build.csx
@@ -1,13 +1,12 @@
-#load "nuget:Dotnet.Build, 0.7.1"
+#load "nuget:Dotnet.Build, 0.23.0"
#load "nuget:dotnet-steps, 0.0.1"
#load "nuget:github-changelog, 0.1.5"
-#load "Choco.csx"
#load "BuildContext.csx"
-using static ReleaseManagement;
+using System.Xml.Linq;
using static ChangeLog;
using static FileUtils;
-using System.Xml.Linq;
+using static ReleaseManagement;
[StepDescription("Runs all tests.")]
Step test = () => RunTests();
@@ -17,7 +16,6 @@ Step pack = () =>
{
CreateGitHubReleaseAsset();
CreateNuGetPackages();
- CreateChocoPackage();
CreateGlobalToolPackage();
};
@@ -35,33 +33,18 @@ await StepRunner.Execute(Args);
private void CreateGitHubReleaseAsset()
{
- DotNet.Publish(dotnetScriptProjectFolder, publishArtifactsFolder, "netcoreapp2.1");
+ DotNet.Publish(dotnetScriptProjectFolder, publishArtifactsFolder, "net8.0");
Zip(publishArchiveFolder, pathToGitHubReleaseAsset);
}
-
-private void CreateChocoPackage()
-{
- if (BuildEnvironment.IsWindows)
- {
- Choco.Pack(dotnetScriptProjectFolder, publishArtifactsFolder, chocolateyArtifactsFolder);
- }
- else
- {
- Logger.Log("The choco package is only built on Windows");
- }
-}
-
private void CreateGlobalToolPackage()
{
- using (var globalToolBuildFolder = new DisposableFolder())
- {
- Copy(solutionFolder, globalToolBuildFolder.Path);
- PatchPackAsTool(globalToolBuildFolder.Path);
- PatchPackageId(globalToolBuildFolder.Path, GlobalToolPackageId);
- PatchContent(globalToolBuildFolder.Path);
- Command.Execute("dotnet", $"pack --configuration release --output {nuGetArtifactsFolder}", Path.Combine(globalToolBuildFolder.Path, "Dotnet.Script"));
- }
+ using var globalToolBuildFolder = new DisposableFolder();
+ Copy(solutionFolder, globalToolBuildFolder.Path);
+ PatchPackAsTool(globalToolBuildFolder.Path);
+ PatchPackageId(globalToolBuildFolder.Path, GlobalToolPackageId);
+ PatchContent(globalToolBuildFolder.Path);
+ Command.Execute("dotnet", $"pack --configuration release --output {nuGetArtifactsFolder}", Path.Combine(globalToolBuildFolder.Path, "Dotnet.Script"));
}
private void CreateNuGetPackages()
@@ -75,7 +58,8 @@ private void CreateNuGetPackages()
private void RunTests()
{
- DotNet.Test(testProjectFolder);
+ Command.Execute("dotnet", "test -c Release -f net8.0", testProjectFolder);
+ Command.Execute("dotnet", "test -c Release -f net9.0", testProjectFolder);
if (BuildEnvironment.IsWindows)
{
DotNet.Test(testDesktopProjectFolder);
@@ -103,8 +87,8 @@ private async Task PublishRelease()
Git.Default.RequireCleanWorkingTree();
await ReleaseManagerFor(owner, projectName, BuildEnvironment.GitHubAccessToken)
.CreateRelease(Git.Default.GetLatestTag(), pathToReleaseNotes, new[] { new ZipReleaseAsset(pathToGitHubReleaseAsset) });
- NuGet.TryPush(nuGetArtifactsFolder);
- Choco.TryPush(chocolateyArtifactsFolder, BuildEnvironment.ChocolateyApiKey);
+
+ DotNet.TryPush(nuGetArtifactsFolder);
}
}
diff --git a/build/BuildContext.csx b/build/BuildContext.csx
index 6cf4505b..1cfeeb07 100644
--- a/build/BuildContext.csx
+++ b/build/BuildContext.csx
@@ -1,4 +1,4 @@
-#load "nuget:Dotnet.Build, 0.7.1"
+#load "nuget:Dotnet.Build, 0.23.0"
using static FileUtils;
using System.Xml.Linq;
@@ -7,7 +7,7 @@ const string GlobalToolPackageId = "dotnet-script";
var owner = "filipw";
var projectName = "dotnet-script";
var root = FileUtils.GetScriptFolder();
-var solutionFolder = Path.Combine(root,"..","src");
+var solutionFolder = Path.Combine(root, "..", "src");
var dotnetScriptProjectFolder = Path.Combine(root, "..", "src", "Dotnet.Script");
var dotnetScriptCoreProjectFolder = Path.Combine(root, "..", "src", "Dotnet.Script.Core");
var dotnetScriptDependencyModelProjectFolder = Path.Combine(root, "..", "src", "Dotnet.Script.DependencyModel");
diff --git a/build/Choco.csx b/build/Choco.csx
deleted file mode 100644
index 9cfcb665..00000000
--- a/build/Choco.csx
+++ /dev/null
@@ -1,96 +0,0 @@
-#load "nuget:Dotnet.Build, 0.7.1"
-
-using System.Xml.Linq;
-
-public static class Choco
-{
- ///
- /// Creates a Chocolatey package based on a "csproj" project file.
- ///
- /// The path to the project folder.
- /// The path to the output folder (*.nupkg)
- public static void Pack(string pathToProjectFolder, string pathToBinaries, string outputFolder)
- {
- string pathToProjectFile = Directory.GetFiles(pathToProjectFolder, "*.csproj").Single();
- CreateSpecificationFromProject(pathToProjectFile, pathToBinaries);
- Command.Execute("choco.exe", $@"pack Chocolatey\chocolatey.nuspec --outputdirectory {outputFolder}");
- }
-
- public static void Push(string packagesFolder, string apiKey, string source = "https://push.chocolatey.org/")
- {
- var packageFiles = Directory.GetFiles(packagesFolder, "*.nupkg");
- foreach (var packageFile in packageFiles)
- {
- Command.Execute("choco.exe", $"push {packageFile} --source {source} --key {apiKey}");
- }
- }
-
- public static void TryPush(string packagesFolder, string apiKey, string source = "https://push.chocolatey.org/")
- {
- var packageFiles = Directory.GetFiles(packagesFolder, "*.nupkg");
- foreach (var packageFile in packageFiles)
- {
- Command.Execute("choco.exe", $"push {packageFile} --source {source} --key {apiKey}");
- }
- }
-
- private static void CreateSpecificationFromProject(string pathToProjectFile, string pathToBinaries)
- {
- var projectFile = XDocument.Load(pathToProjectFile);
- var authors = projectFile.Descendants("Authors").SingleOrDefault()?.Value;
- var packageId = projectFile.Descendants("PackageId").SingleOrDefault()?.Value;
- var description = projectFile.Descendants("Description").SingleOrDefault()?.Value;
- var versionPrefix = projectFile.Descendants("VersionPrefix").SingleOrDefault()?.Value;
- var versionSuffix = projectFile.Descendants("VersionSuffix").SingleOrDefault()?.Value;
-
- string version;
- if (versionSuffix != null)
- {
- version = $"{versionPrefix}-{versionSuffix}";
- }
- else
- {
- version = versionPrefix;
- }
- var tags = projectFile.Descendants("PackageTags").SingleOrDefault()?.Value;
- var iconUrl = projectFile.Descendants("PackageIconUrl").SingleOrDefault()?.Value;
- var projectUrl = projectFile.Descendants("PackageProjectUrl").SingleOrDefault()?.Value;
- var repositoryUrl = projectFile.Descendants("RepositoryUrl").SingleOrDefault()?.Value;
-
- var packageElement = new XElement("package");
- var metadataElement = new XElement("metadata");
- packageElement.Add(metadataElement);
-
- // Package id should be lower case
- // https://chocolatey.org/docs/create-packages#naming-your-package
- metadataElement.Add(new XElement("id", packageId.ToLower()));
- metadataElement.Add(new XElement("version", version));
- metadataElement.Add(new XElement("authors", authors));
- metadataElement.Add(new XElement("licenseUrl", "https://licenses.nuget.org/MIT"));
- metadataElement.Add(new XElement("projectUrl", projectUrl));
- metadataElement.Add(new XElement("iconUrl", iconUrl));
- metadataElement.Add(new XElement("description", description));
- metadataElement.Add(new XElement("tags", repositoryUrl));
-
- var filesElement = new XElement("files");
- packageElement.Add(filesElement);
-
- // Add the tools folder that contains "ChocolateyInstall.ps1"
- filesElement.Add(CreateFileElement(@"tools\*.*", $@"{packageId}\tools"));
- var srcGlobPattern = $@"{pathToBinaries}\**\*";
- filesElement.Add(CreateFileElement(srcGlobPattern, packageId));
-
- using (var fileStream = new FileStream("Chocolatey/chocolatey.nuspec", FileMode.Create))
- {
- new XDocument(packageElement).Save(fileStream);
- }
- }
-
- private static XElement CreateFileElement(string src, string target)
- {
- var srcAttribute = new XAttribute("src", src);
- var targetAttribute = new XAttribute("target", target);
- return new XElement("file", srcAttribute, targetAttribute);
- }
-
-}
\ No newline at end of file
diff --git a/build/Chocolatey/tools/ChocolateyInstall.ps1 b/build/Chocolatey/tools/ChocolateyInstall.ps1
deleted file mode 100644
index 23b027f6..00000000
--- a/build/Chocolatey/tools/ChocolateyInstall.ps1
+++ /dev/null
@@ -1,3 +0,0 @@
-# Add the binary folder to the users PATH environment variable.
-$pathToBinaries = Join-Path -Path $($env:ChocolateyPackageFolder) -ChildPath $($env:ChocolateyPackageName )
-Install-ChocolateyPath -PathToInstall "$pathToBinaries" -PathType 'Machine'
\ No newline at end of file
diff --git a/build/Chocolatey/tools/LICENSE.TXT b/build/Chocolatey/tools/LICENSE.TXT
deleted file mode 100644
index f8a5c9f2..00000000
--- a/build/Chocolatey/tools/LICENSE.TXT
+++ /dev/null
@@ -1,19 +0,0 @@
-Copyright (c) 2019 dotnet-script
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
\ No newline at end of file
diff --git a/build/Chocolatey/tools/VERIFICATION.TXT b/build/Chocolatey/tools/VERIFICATION.TXT
deleted file mode 100644
index 1936dea3..00000000
--- a/build/Chocolatey/tools/VERIFICATION.TXT
+++ /dev/null
@@ -1,8 +0,0 @@
-VERIFICATION
-Verification is intended to assist the Chocolatey moderators and community
-in verifying that this package's contents are trustworthy.
-
-dotnet-script.dll : 3BF42E83BE931AC98D02306DBC6FB319
-
-Check against the corresponding file in this release.
-https://github.com/filipw/dotnet-script/releases/download/0.13.0/dotnet-script.0.13.0.zip
\ No newline at end of file
diff --git a/build/Dockerfile b/build/Dockerfile
index 44e5014e..876d8d79 100644
--- a/build/Dockerfile
+++ b/build/Dockerfile
@@ -1,4 +1,4 @@
-FROM mcr.microsoft.com/dotnet/core/sdk:3.1
+FROM mcr.microsoft.com/dotnet/sdk:7.0
# https://www.nuget.org/packages/dotnet-script/
RUN dotnet tool install dotnet-script --tool-path /usr/bin
diff --git a/build/install-dotnet-script.ps1 b/build/install-dotnet-script.ps1
index 2e0def4c..2b155019 100644
--- a/build/install-dotnet-script.ps1
+++ b/build/install-dotnet-script.ps1
@@ -2,7 +2,7 @@
$scriptRoot = Split-Path $MyInvocation.MyCommand.Path -Parent
$client = New-Object "System.Net.WebClient"
-$url = "https://github.com/filipw/dotnet-script/releases/download/0.18.0/dotnet-script.0.18.0.zip"
+$url = "https://github.com/dotnet-script/dotnet-script/releases/download/0.18.0/dotnet-script.0.18.0.zip"
$file = "$scriptRoot/dotnet-script.zip"
$client.DownloadFile($url,$file)
Expand-Archive $file -DestinationPath $scriptRoot -Force
\ No newline at end of file
diff --git a/build/install-dotnet-script.sh b/build/install-dotnet-script.sh
index 9242df21..4b3727c9 100755
--- a/build/install-dotnet-script.sh
+++ b/build/install-dotnet-script.sh
@@ -1,4 +1,4 @@
#!/bin/bash
-curl -L https://github.com/filipw/dotnet-script/releases/download/0.18.0/dotnet-script.0.18.0.zip > dotnet-script.zip
+curl -L https://github.com/dotnet-script/dotnet-script/releases/download/0.18.0/dotnet-script.0.18.0.zip > dotnet-script.zip
unzip -o dotnet-script.zip -d ./
diff --git a/build/omnisharp.json b/build/omnisharp.json
index 5c14541a..945a3c21 100644
--- a/build/omnisharp.json
+++ b/build/omnisharp.json
@@ -1,6 +1,6 @@
{
"script": {
"enableScriptNuGetReferences": true,
- "defaultTargetFramework": "netcoreapp2.1"
+ "defaultTargetFramework": "net8.0"
}
}
\ No newline at end of file
diff --git a/build/prerestore.Dockerfile b/build/prerestore.Dockerfile
new file mode 100644
index 00000000..48894424
--- /dev/null
+++ b/build/prerestore.Dockerfile
@@ -0,0 +1,13 @@
+FROM mcr.microsoft.com/dotnet/sdk:7.0
+
+# https://www.nuget.org/packages/dotnet-script/
+RUN dotnet tool install dotnet-script --tool-path /usr/bin
+
+# Create a simple script, execute it and cleanup after
+# to create the script project dir, which requires
+# 'dotnet restore' to be run.
+# This is necessary if you want to run this in a networkless
+# docker container.
+RUN dotnet script eval "Console.WriteLine(\"☑️ Prepared env for offline usage\")"
+
+ENTRYPOINT [ "dotnet", "script" ]
diff --git a/global.json b/global.json
index 94eb6594..97614a3d 100644
--- a/global.json
+++ b/global.json
@@ -1,6 +1,6 @@
{
"sdk": {
- "version": "5.0.100",
+ "version": "9.0.100",
"rollForward": "latestFeature"
}
}
diff --git a/install/install.ps1 b/install/install.ps1
index 2e8093ab..a0680936 100644
--- a/install/install.ps1
+++ b/install/install.ps1
@@ -5,7 +5,7 @@ $tempFolder = Join-Path $env:TEMP "dotnet-script"
New-Item $tempFolder -ItemType Directory -Force
# Get the latest release
-$latestRelease = Invoke-WebRequest "https://api.github.com/repos/filipw/dotnet-script/releases/latest" |
+$latestRelease = Invoke-WebRequest "https://api.github.com/repos/dotnet-script/dotnet-script/releases/latest" |
ConvertFrom-Json |
Select-Object tag_name
$tag_name = $latestRelease.tag_name
@@ -13,7 +13,7 @@ $tag_name = $latestRelease.tag_name
# Download the zip
Write-Host "Downloading latest version ($tag_name)"
$client = New-Object "System.Net.WebClient"
-$url = "https://github.com/filipw/dotnet-script/releases/download/$tag_name/dotnet-script.$tag_name.zip"
+$url = "https://github.com/dotnet-script/dotnet-script/releases/download/$tag_name/dotnet-script.$tag_name.zip"
$zipFile = Join-Path $tempFolder "dotnet-script.zip"
$client.DownloadFile($url,$zipFile)
diff --git a/install/install.sh b/install/install.sh
index 3a464fc7..3cdf9ce5 100755
--- a/install/install.sh
+++ b/install/install.sh
@@ -1,7 +1,7 @@
#!/bin/bash
mkdir /tmp/dotnet-script
if [[ -z $1 ]]; then
- version=$(curl https://api.github.com/repos/filipw/dotnet-script/releases/latest | grep -Eo "\"tag_name\":\s*\"(.*)\"" | cut -d'"' -f4)
+ version=$(curl https://api.github.com/repos/dotnet-script/dotnet-script/releases/latest | grep -Eo "\"tag_name\":\s*\"(.*)\"" | cut -d'"' -f4)
else
version=$1
fi
@@ -15,7 +15,7 @@ if [[ $? -eq 0 ]]; then
fi
echo "Installing $version..."
-curl -L https://github.com/filipw/dotnet-script/releases/download/$version/dotnet-script.$version.zip > /tmp/dotnet-script/dotnet-script.zip
+curl -L https://github.com/dotnet-script/dotnet-script/releases/download/$version/dotnet-script.$version.zip > /tmp/dotnet-script/dotnet-script.zip
unzip -o /tmp/dotnet-script/dotnet-script.zip -d /usr/local/lib
chmod +x /usr/local/lib/dotnet-script/dotnet-script.sh
cd /usr/local/bin
diff --git a/omnisharp.json b/omnisharp.json
index 93c5d8d9..973aa4c7 100644
--- a/omnisharp.json
+++ b/omnisharp.json
@@ -8,7 +8,7 @@
},
"script": {
"enableScriptNuGetReferences": true,
- "defaultTargetFramework": "net5.0"
+ "defaultTargetFramework": "net6.0"
},
"FormattingOptions": {
"organizeImports": true,
diff --git a/src/.vscode/tasks.json b/src/.vscode/tasks.json
index 4046a0bb..d778ce8d 100644
--- a/src/.vscode/tasks.json
+++ b/src/.vscode/tasks.json
@@ -6,12 +6,34 @@
"command": "dotnet",
"args": [
"build",
- "${workspaceRoot}/Dotnet.Script/Dotnet.Script.csproj",
"/property:GenerateFullPaths=true"
],
- "group": {
- "kind": "build",
- "isDefault": true
+ "options": {
+ "cwd": "${workspaceFolder}/.."
+ },
+ "type": "shell",
+ "group": "build",
+ "presentation": {
+ "reveal": "always"
+ },
+ "problemMatcher": "$msCompile"
+ },
+ {
+ "label": "rebuild",
+ "command": "dotnet",
+ "args": [
+ "build",
+ "--no-incremental",
+ "/property:GenerateFullPaths=true"
+ ],
+ "options": {
+ "cwd": "${workspaceFolder}/.."
+ },
+ "type": "shell",
+ "group": "build",
+ "presentation": {
+ "reveal": "always",
+ "clear": true
},
"problemMatcher": "$msCompile"
},
@@ -24,7 +46,7 @@
"-c",
"release",
"-f",
- "netcoreapp3.1",
+ "net9.0",
"${workspaceFolder}/Dotnet.Script.Tests/DotNet.Script.Tests.csproj"
],
"problemMatcher": "$msCompile",
@@ -55,4 +77,4 @@
}
}
]
-}
+}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteCodeCommand.cs b/src/Dotnet.Script.Core/Commands/ExecuteCodeCommand.cs
index 688540b0..b9b6b039 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteCodeCommand.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteCodeCommand.cs
@@ -22,15 +22,19 @@ public async Task Execute(ExecuteCodeCommandOptions options)
{
var sourceText = SourceText.From(options.Code);
var context = new ScriptContext(sourceText, options.WorkingDirectory ?? Directory.GetCurrentDirectory(), options.Arguments, null, options.OptimizationLevel, ScriptMode.Eval, options.PackageSources);
- var compiler = GetScriptCompiler(!options.NoCache, _logFactory);
- var runner = new ScriptRunner(compiler, _logFactory, _scriptConsole);
+ var compiler = new ScriptCompiler(_logFactory, !options.NoCache)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
+ var runner = new ScriptRunner(compiler, _logFactory, _scriptConsole)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
return await runner.Execute(context);
}
-
- private static ScriptCompiler GetScriptCompiler(bool useRestoreCache, LogFactory logFactory)
- {
- var compiler = new ScriptCompiler(logFactory, useRestoreCache);
- return compiler;
- }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteCodeCommandOptions.cs b/src/Dotnet.Script.Core/Commands/ExecuteCodeCommandOptions.cs
index b1bdf40b..11ebf3a7 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteCodeCommandOptions.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteCodeCommandOptions.cs
@@ -1,4 +1,7 @@
using Microsoft.CodeAnalysis;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
namespace Dotnet.Script.Core.Commands
{
@@ -20,5 +23,14 @@ public ExecuteCodeCommandOptions(string code, string workingDirectory, string[]
public OptimizationLevel OptimizationLevel { get; }
public bool NoCache { get; }
public string[] PackageSources { get; }
+
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommand.cs b/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommand.cs
index 17d4f3cf..a883fce2 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommand.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommand.cs
@@ -18,7 +18,12 @@ public ExecuteInteractiveCommand(ScriptConsole scriptConsole, LogFactory logFact
public async Task Execute(ExecuteInteractiveCommandOptions options)
{
- var compiler = new ScriptCompiler(_logFactory, useRestoreCache: false);
+ var compiler = new ScriptCompiler(_logFactory, useRestoreCache: false)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
var runner = new InteractiveRunner(compiler, _logFactory, _scriptConsole, options.PackageSources);
if (options.ScriptFile == null)
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommandOptions.cs b/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommandOptions.cs
index 5b189d06..bb7658ca 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommandOptions.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteInteractiveCommandOptions.cs
@@ -1,10 +1,12 @@
-using Microsoft.CodeAnalysis;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
namespace Dotnet.Script.Core.Commands
{
public class ExecuteInteractiveCommandOptions
{
- public ExecuteInteractiveCommandOptions(ScriptFile scriptFile, string[] arguments ,string[] packageSources)
+ public ExecuteInteractiveCommandOptions(ScriptFile scriptFile, string[] arguments, string[] packageSources)
{
ScriptFile = scriptFile;
Arguments = arguments;
@@ -14,5 +16,14 @@ public ExecuteInteractiveCommandOptions(ScriptFile scriptFile, string[] argument
public ScriptFile ScriptFile { get; }
public string[] Arguments { get; }
public string[] PackageSources { get; }
+
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommand.cs b/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommand.cs
index d7350e82..f2a9fd37 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommand.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommand.cs
@@ -25,16 +25,20 @@ public async Task Execute(ExecuteLibraryCommandOptions options
}
var absoluteFilePath = options.LibraryPath.GetRootedPath();
- var compiler = GetScriptCompiler(!options.NoCache, _logFactory);
- var runner = new ScriptRunner(compiler, _logFactory, _scriptConsole);
+ var compiler = new ScriptCompiler(_logFactory, !options.NoCache)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
+ var runner = new ScriptRunner(compiler, _logFactory, _scriptConsole)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
var result = await runner.Execute(absoluteFilePath, options.Arguments);
return result;
}
-
- private static ScriptCompiler GetScriptCompiler(bool useRestoreCache, LogFactory logFactory)
- {
- var compiler = new ScriptCompiler(logFactory, useRestoreCache);
- return compiler;
- }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommandOptions.cs b/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommandOptions.cs
index 2c0f4796..a3c3cb7e 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommandOptions.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteLibraryCommandOptions.cs
@@ -1,3 +1,7 @@
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
+
namespace Dotnet.Script.Core.Commands
{
public class ExecuteLibraryCommandOptions
@@ -12,5 +16,14 @@ public ExecuteLibraryCommandOptions(string libraryPath, string[] arguments, bool
public string LibraryPath { get; }
public string[] Arguments { get; }
public bool NoCache { get; }
+
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteScriptCommand.cs b/src/Dotnet.Script.Core/Commands/ExecuteScriptCommand.cs
index 470a4e52..0712495a 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteScriptCommand.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteScriptCommand.cs
@@ -31,8 +31,15 @@ public async Task Run(ExecuteScriptCommandOptions optio
return await DownloadAndRunCode(options);
}
- var pathToLibrary = GetLibrary(options);
- return await ExecuteLibrary(pathToLibrary, options.Arguments, options.NoCache);
+ var pathToLibrary = GetLibrary(options);
+
+ var libraryOptions = new ExecuteLibraryCommandOptions(pathToLibrary, options.Arguments, options.NoCache)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
+ return await new ExecuteLibraryCommand(_scriptConsole, _logFactory).Execute(libraryOptions);
}
private async Task DownloadAndRunCode(ExecuteScriptCommandOptions executeOptions)
@@ -43,7 +50,7 @@ private async Task DownloadAndRunCode(ExecuteScriptCommandOpti
return await new ExecuteCodeCommand(_scriptConsole, _logFactory).Execute(options);
}
- private string GetLibrary(ExecuteScriptCommandOptions executeOptions)
+ private string GetLibrary(ExecuteScriptCommandOptions executeOptions)
{
var projectFolder = FileUtils.GetPathToScriptTempFolder(executeOptions.File.Path);
var executionCacheFolder = Path.Combine(projectFolder, "execution-cache");
@@ -57,8 +64,13 @@ private string GetLibrary(ExecuteScriptCommandOptions executeOptions)
return pathToLibrary;
}
- var options = new PublishCommandOptions(executeOptions.File, executionCacheFolder, "script", PublishType.Library, executeOptions.OptimizationLevel, executeOptions.PackageSources, null, executeOptions.NoCache);
- new PublishCommand(_scriptConsole, _logFactory).Execute(options);
+ var options = new PublishCommandOptions(executeOptions.File, executionCacheFolder, "script", PublishType.Library, executeOptions.OptimizationLevel, executeOptions.PackageSources, null, executeOptions.NoCache)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = executeOptions.AssemblyLoadContext
+#endif
+ };
+ new PublishCommand(_scriptConsole, _logFactory).Execute(options);
if (hash != null)
{
File.WriteAllText(Path.Combine(executionCacheFolder, "script.sha256"), hash);
@@ -124,11 +136,5 @@ public bool TryGetHash(string cacheFolder, out string hash)
hash = File.ReadAllText(pathToHashFile);
return true;
}
-
- private async Task ExecuteLibrary(string pathToLibrary, string[] arguments, bool noCache)
- {
- var options = new ExecuteLibraryCommandOptions(pathToLibrary, arguments, noCache);
- return await new ExecuteLibraryCommand(_scriptConsole, _logFactory).Execute(options);
- }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/ExecuteScriptCommandOptions.cs b/src/Dotnet.Script.Core/Commands/ExecuteScriptCommandOptions.cs
index 656cb74d..93577f01 100644
--- a/src/Dotnet.Script.Core/Commands/ExecuteScriptCommandOptions.cs
+++ b/src/Dotnet.Script.Core/Commands/ExecuteScriptCommandOptions.cs
@@ -1,4 +1,7 @@
using Microsoft.CodeAnalysis;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
namespace Dotnet.Script.Core.Commands
{
@@ -20,5 +23,14 @@ public ExecuteScriptCommandOptions(ScriptFile file, string[] arguments, Optimiza
public string[] PackageSources { get; }
public bool IsInteractive { get; }
public bool NoCache { get; }
+
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/PublishCommand.cs b/src/Dotnet.Script.Core/Commands/PublishCommand.cs
index 684911c0..08a09ffb 100644
--- a/src/Dotnet.Script.Core/Commands/PublishCommand.cs
+++ b/src/Dotnet.Script.Core/Commands/PublishCommand.cs
@@ -18,6 +18,11 @@ public PublishCommand(ScriptConsole scriptConsole, LogFactory logFactory)
}
public void Execute(PublishCommandOptions options)
+ {
+ Execute(options);
+ }
+
+ public void Execute(PublishCommandOptions options)
{
var absoluteFilePath = options.File.Path;
@@ -28,7 +33,12 @@ public void Execute(PublishCommandOptions options)
(options.PublishType == PublishType.Library ? Path.Combine(Path.GetDirectoryName(absoluteFilePath), "publish") : Path.Combine(Path.GetDirectoryName(absoluteFilePath), "publish", options.RuntimeIdentifier));
var absolutePublishDirectory = publishDirectory.GetRootedPath();
- var compiler = GetScriptCompiler(!options.NoCache, _logFactory);
+ var compiler = new ScriptCompiler(_logFactory, !options.NoCache)
+ {
+#if NETCOREAPP
+ AssemblyLoadContext = options.AssemblyLoadContext
+#endif
+ };
var scriptEmitter = new ScriptEmitter(_scriptConsole, compiler);
var publisher = new ScriptPublisher(_logFactory, scriptEmitter);
var code = absoluteFilePath.ToSourceText();
@@ -36,19 +46,12 @@ public void Execute(PublishCommandOptions options)
if (options.PublishType == PublishType.Library)
{
- publisher.CreateAssembly(context, _logFactory, options.LibraryName);
+ publisher.CreateAssembly(context, _logFactory, options.LibraryName);
}
else
{
- publisher.CreateExecutable(context, _logFactory, options.RuntimeIdentifier, options.LibraryName);
+ publisher.CreateExecutable(context, _logFactory, options.RuntimeIdentifier, options.LibraryName);
}
}
-
- private static ScriptCompiler GetScriptCompiler(bool useRestoreCache, LogFactory logFactory)
- {
-
- var compiler = new ScriptCompiler(logFactory, useRestoreCache);
- return compiler;
- }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Commands/PublishCommandOptions.cs b/src/Dotnet.Script.Core/Commands/PublishCommandOptions.cs
index a82fa295..7dd44e1c 100644
--- a/src/Dotnet.Script.Core/Commands/PublishCommandOptions.cs
+++ b/src/Dotnet.Script.Core/Commands/PublishCommandOptions.cs
@@ -1,5 +1,8 @@
using Dotnet.Script.DependencyModel.Environment;
using Microsoft.CodeAnalysis;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
namespace Dotnet.Script.Core.Commands
{
@@ -25,6 +28,15 @@ public PublishCommandOptions(ScriptFile file, string outputDirectory, string lib
public string[] PackageSources { get; }
public string RuntimeIdentifier { get; }
public bool NoCache { get; }
+
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script isolation.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
}
public enum PublishType
diff --git a/src/Dotnet.Script.Core/Dotnet.Script.Core.csproj b/src/Dotnet.Script.Core/Dotnet.Script.Core.csproj
index 24a97b2f..94dd6e45 100644
--- a/src/Dotnet.Script.Core/Dotnet.Script.Core.csproj
+++ b/src/Dotnet.Script.Core/Dotnet.Script.Core.csproj
@@ -2,20 +2,21 @@
A cross platform library allowing you to run C# (CSX) scripts with support for debugging and inline NuGet packages. Based on Roslyn.
- 1.1.0
+ 1.6.0
filipw
- netstandard2.0
+ net9.0;net8.0;netstandard2.0
Dotnet.Script.Core
Dotnet.Script.Core
script;csx;csharp;roslyn
- https://raw.githubusercontent.com/filipw/Strathweb.TypedRouting.AspNetCore/master/strathweb.png
- https://github.com/filipw/dotnet-script
+ https://avatars.githubusercontent.com/u/113979420
+ https://github.com/dotnet-script/dotnet-script
MIT
git
- https://github.com/filipw/dotnet-script.git
+ https://github.com/dotnet-script/dotnet-script.git
false
false
false
+ 9.0
true
../dotnet-script.snk
@@ -23,21 +24,18 @@
-
-
+
+
+
-
-
-
-
-
-
+
+
+
+
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Extensions.cs b/src/Dotnet.Script.Core/Extensions.cs
index dbe06315..83c10fc7 100644
--- a/src/Dotnet.Script.Core/Extensions.cs
+++ b/src/Dotnet.Script.Core/Extensions.cs
@@ -9,10 +9,8 @@ public static class Extensions
public static SourceText ToSourceText(this string absoluteFilePath)
{
- using (var filestream = File.OpenRead(absoluteFilePath))
- {
- return SourceText.From(filestream);
- }
+ using var filestream = File.OpenRead(absoluteFilePath);
+ return SourceText.From(filestream);
}
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Interactive/InteractiveRunner.cs b/src/Dotnet.Script.Core/Interactive/InteractiveRunner.cs
index 99473fd5..4ffcecf4 100644
--- a/src/Dotnet.Script.Core/Interactive/InteractiveRunner.cs
+++ b/src/Dotnet.Script.Core/Interactive/InteractiveRunner.cs
@@ -26,7 +26,7 @@ public class InteractiveRunner
protected ScriptCompiler ScriptCompiler;
protected ScriptConsole Console;
private readonly string[] _packageSources;
- protected CSharpParseOptions ParseOptions = new CSharpParseOptions(LanguageVersion.Latest, kind: SourceCodeKind.Script);
+ protected CSharpParseOptions ParseOptions = new CSharpParseOptions(LanguageVersion.Preview, kind: SourceCodeKind.Script);
protected InteractiveCommandProvider InteractiveCommandParser = new InteractiveCommandProvider();
protected string CurrentDirectory = Directory.GetCurrentDirectory();
diff --git a/src/Dotnet.Script.Core/Internal/PreprocessorLineRewriter.cs b/src/Dotnet.Script.Core/Internal/PreprocessorLineRewriter.cs
index 84ef8cdf..e2d88866 100644
--- a/src/Dotnet.Script.Core/Internal/PreprocessorLineRewriter.cs
+++ b/src/Dotnet.Script.Core/Internal/PreprocessorLineRewriter.cs
@@ -22,13 +22,13 @@ public override SyntaxNode VisitReferenceDirectiveTrivia(ReferenceDirectiveTrivi
return HandleSkippedTrivia(base.VisitReferenceDirectiveTrivia(node));
}
- private SyntaxNode HandleSkippedTrivia(SyntaxNode node)
+ private static SyntaxNode HandleSkippedTrivia(SyntaxNode node)
{
var skippedTrivia = node.DescendantTrivia().Where(x => x.RawKind == (int)SyntaxKind.SkippedTokensTrivia).FirstOrDefault();
- if (skippedTrivia != null && skippedTrivia.Token.Kind() != SyntaxKind.None)
+ if (!skippedTrivia.Token.IsKind(SyntaxKind.None))
{
var firstToken = skippedTrivia.GetStructure().ChildTokens().FirstOrDefault();
- if (firstToken != null && firstToken.Kind() == SyntaxKind.BadToken && firstToken.ToFullString().Trim() == ";")
+ if (firstToken.IsKind(SyntaxKind.BadToken) && firstToken.ToFullString().Trim() == ";")
{
node = node.ReplaceToken(firstToken, SyntaxFactory.Token(SyntaxKind.None));
skippedTrivia = node.DescendantTrivia().Where(x => x.RawKind == (int)SyntaxKind.SkippedTokensTrivia).FirstOrDefault();
diff --git a/src/Dotnet.Script.Core/Scaffolder.cs b/src/Dotnet.Script.Core/Scaffolder.cs
index ef66970a..8e52acc1 100644
--- a/src/Dotnet.Script.Core/Scaffolder.cs
+++ b/src/Dotnet.Script.Core/Scaffolder.cs
@@ -2,12 +2,12 @@
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Logging;
using Dotnet.Script.DependencyModel.Process;
-using Newtonsoft.Json.Linq;
using System;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
+using System.Text.Json.Nodes;
using System.Text.RegularExpressions;
namespace Dotnet.Script.Core
@@ -81,6 +81,7 @@ public void RegisterFileHandler()
if (RuntimeInformation.IsOSPlatform(OSPlatform.Windows))
{
// register dotnet-script as the tool to process .csx files
+ _commandRunner.Execute("reg", @"delete HKCU\Software\classes\.csx /f");
_commandRunner.Execute("reg", @"add HKCU\Software\classes\.csx /f /ve /t REG_SZ /d dotnetscript");
_commandRunner.Execute("reg", $@"add HKCU\Software\Classes\dotnetscript\Shell\Open\Command /f /ve /t REG_EXPAND_SZ /d ""\""%ProgramFiles%\dotnet\dotnet.exe\"" script \""%1\"" -- %*""");
}
@@ -119,7 +120,7 @@ private void CreateOmniSharpConfigurationFile(string currentWorkingDirectory)
if (!File.Exists(pathToOmniSharpJson))
{
var omniSharpFileTemplate = TemplateLoader.ReadTemplate("omnisharp.json.template");
- JObject settings = JObject.Parse(omniSharpFileTemplate);
+ var settings = JsonObject.Parse(omniSharpFileTemplate);
settings["script"]["defaultTargetFramework"] = _scriptEnvironment.TargetFramework;
File.WriteAllText(pathToOmniSharpJson, settings.ToString());
_scriptConsole.WriteSuccess($"...'{pathToOmniSharpJson}' [Created]");
@@ -141,7 +142,7 @@ private void CreateLaunchConfiguration(string currentWorkingDirectory)
_scriptConsole.WriteNormal("Creating VS Code launch configuration file");
string pathToLaunchFile = Path.Combine(vsCodeDirectory, "launch.json");
string installLocation = _scriptEnvironment.InstallLocation;
- bool isInstalledAsGlobalTool = installLocation.Contains(".dotnet/tools", StringComparison.OrdinalIgnoreCase);
+ bool isInstalledAsGlobalTool = installLocation.Contains($".dotnet{Path.DirectorySeparatorChar}tools", StringComparison.OrdinalIgnoreCase);
string dotnetScriptPath = Path.Combine(installLocation, "dotnet-script.dll").Replace(@"\", "/");
string launchFileContent;
if (!File.Exists(pathToLaunchFile))
diff --git a/src/Dotnet.Script.Core/ScriptAssemblyLoadContext.cs b/src/Dotnet.Script.Core/ScriptAssemblyLoadContext.cs
new file mode 100644
index 00000000..603661ae
--- /dev/null
+++ b/src/Dotnet.Script.Core/ScriptAssemblyLoadContext.cs
@@ -0,0 +1,170 @@
+#if NET
+
+using System;
+using System.Reflection;
+using System.Runtime.Loader;
+
+#nullable enable
+
+namespace Dotnet.Script.Core
+{
+ ///
+ /// Represents assembly load context for a script with full and automatic assembly isolation.
+ ///
+ public class ScriptAssemblyLoadContext : AssemblyLoadContext
+ {
+ ///
+ /// Initializes a new instance of the class.
+ ///
+ public ScriptAssemblyLoadContext()
+ {
+ }
+
+#if NET5_0_OR_GREATER
+ ///
+ /// Initializes a new instance of the class
+ /// with a name and a value that indicates whether unloading is enabled.
+ ///
+ ///
+ ///
+ public ScriptAssemblyLoadContext(string? name, bool isCollectible = false) :
+ base(name, isCollectible)
+ {
+ }
+
+ ///
+ /// Initializes a new instance of the class
+ /// with a value that indicates whether unloading is enabled.
+ ///
+ ///
+ protected ScriptAssemblyLoadContext(bool isCollectible) :
+ base(isCollectible)
+ {
+ }
+#endif
+
+ ///
+ ///
+ /// Gets the value indicating whether a specified assembly is homogeneous.
+ ///
+ ///
+ /// Homogeneous assemblies are those shared by both host and scripts.
+ ///
+ ///
+ /// The assembly name.
+ /// true if the specified assembly is homogeneous; otherwise, false.
+ protected internal virtual bool IsHomogeneousAssembly(AssemblyName assemblyName)
+ {
+ var name = assemblyName.Name;
+ return
+ string.Equals(name, "mscorlib", StringComparison.OrdinalIgnoreCase) ||
+ string.Equals(name, "Microsoft.CodeAnalysis.Scripting", StringComparison.OrdinalIgnoreCase);
+ }
+
+ ///
+ protected override Assembly? Load(AssemblyName assemblyName) => InvokeLoading(assemblyName);
+
+ ///
+ protected override IntPtr LoadUnmanagedDll(string unmanagedDllName) => InvokeLoadingUnmanagedDll(unmanagedDllName);
+
+ ///
+ /// Provides data for the event.
+ ///
+ internal sealed class LoadingEventArgs : EventArgs
+ {
+ public LoadingEventArgs(AssemblyName assemblyName)
+ {
+ Name = assemblyName;
+ }
+
+ public AssemblyName Name { get; }
+ }
+
+ ///
+ /// Represents a method that handles the event.
+ ///
+ /// The sender.
+ /// The arguments.
+ /// The loaded assembly or null if the assembly cannot be resolved.
+ internal delegate Assembly? LoadingEventHandler(ScriptAssemblyLoadContext sender, LoadingEventArgs args);
+
+ LoadingEventHandler? m_Loading;
+
+ ///
+ /// Occurs when an assembly is being loaded.
+ ///
+ internal event LoadingEventHandler Loading
+ {
+ add => m_Loading += value;
+ remove => m_Loading -= value;
+ }
+
+ Assembly? InvokeLoading(AssemblyName assemblyName)
+ {
+ var eh = m_Loading;
+ if (eh != null)
+ {
+ var args = new LoadingEventArgs(assemblyName);
+ foreach (LoadingEventHandler handler in eh.GetInvocationList())
+ {
+ var assembly = handler(this, args);
+ if (assembly != null)
+ return assembly;
+ }
+ }
+ return null;
+ }
+
+ ///
+ /// Provides data for the event.
+ ///
+ internal sealed class LoadingUnmanagedDllEventArgs : EventArgs
+ {
+ public LoadingUnmanagedDllEventArgs(string unmanagedDllName, Func loadUnmanagedDllFromPath)
+ {
+ UnmanagedDllName = unmanagedDllName;
+ LoadUnmanagedDllFromPath = loadUnmanagedDllFromPath;
+ }
+
+ public string UnmanagedDllName { get; }
+ public Func LoadUnmanagedDllFromPath { get; }
+ }
+
+ ///
+ /// Represents a method that handles the event.
+ ///
+ /// The sender.
+ /// The arguments.
+ /// The loaded DLL or if the DLL cannot be resolved.
+ internal delegate IntPtr LoadingUnmanagedDllEventHandler(ScriptAssemblyLoadContext sender, LoadingUnmanagedDllEventArgs args);
+
+ LoadingUnmanagedDllEventHandler? m_LoadingUnmanagedDll;
+
+ ///
+ /// Occurs when an unmanaged DLL is being loaded.
+ ///
+ internal event LoadingUnmanagedDllEventHandler LoadingUnmanagedDll
+ {
+ add => m_LoadingUnmanagedDll += value;
+ remove => m_LoadingUnmanagedDll -= value;
+ }
+
+ IntPtr InvokeLoadingUnmanagedDll(string unmanagedDllName)
+ {
+ var eh = m_LoadingUnmanagedDll;
+ if (eh != null)
+ {
+ var args = new LoadingUnmanagedDllEventArgs(unmanagedDllName, LoadUnmanagedDllFromPath);
+ foreach (LoadingUnmanagedDllEventHandler handler in eh.GetInvocationList())
+ {
+ var dll = handler(this, args);
+ if (dll != IntPtr.Zero)
+ return dll;
+ }
+ }
+ return IntPtr.Zero;
+ }
+ }
+}
+
+#endif
diff --git a/src/Dotnet.Script.Core/ScriptCompiler.cs b/src/Dotnet.Script.Core/ScriptCompiler.cs
index 994cb39f..2cfdab5e 100644
--- a/src/Dotnet.Script.Core/ScriptCompiler.cs
+++ b/src/Dotnet.Script.Core/ScriptCompiler.cs
@@ -3,6 +3,9 @@
using System.Collections.Immutable;
using System.Linq;
using System.Reflection;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
using System.Text;
using System.Threading.Tasks;
using Dotnet.Script.Core.Internal;
@@ -36,6 +39,7 @@ static ScriptCompiler()
});
}
+ //Note: We should set this according to the SDK being used
protected virtual IEnumerable ImportedNamespaces => new[]
{
"System",
@@ -78,11 +82,20 @@ private ScriptCompiler(LogFactory logFactory, RuntimeDependencyResolver runtimeD
}
}
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
+
public virtual ScriptOptions CreateScriptOptions(ScriptContext context, IList runtimeDependencies)
{
var scriptMap = runtimeDependencies.ToDictionary(rdt => rdt.Name, rdt => rdt.Scripts);
var opts = ScriptOptions.Default.AddImports(ImportedNamespaces)
- .WithSourceResolver(new NuGetSourceReferenceResolver(new SourceFileResolver(ImmutableArray.Empty, context.WorkingDirectory),scriptMap))
+ .WithSourceResolver(new NuGetSourceReferenceResolver(new SourceFileResolver(ImmutableArray.Empty, context.WorkingDirectory), scriptMap))
.WithMetadataResolver(new NuGetMetadataReferenceResolver(ScriptMetadataResolver.Default.WithBaseDirectory(context.WorkingDirectory)))
.WithEmitDebugInformation(true)
.WithLanguageVersion(LanguageVersion.Preview)
@@ -176,7 +189,19 @@ private ScriptOptions AddScriptReferences(ScriptOptions scriptOptions, Dictionar
{
foreach (var runtimeAssembly in scriptDependenciesMap.Values)
{
- loadedAssembliesMap.TryGetValue(runtimeAssembly.Name.Name, out var loadedAssembly);
+ bool homogenization;
+#if NETCOREAPP
+ homogenization =
+ AssemblyLoadContext is not ScriptAssemblyLoadContext salc ||
+ salc.IsHomogeneousAssembly(runtimeAssembly.Name);
+#else
+ homogenization = true;
+#endif
+
+ Assembly loadedAssembly = null;
+ if (homogenization)
+ loadedAssembliesMap.TryGetValue(runtimeAssembly.Name.Name, out loadedAssembly);
+
if (loadedAssembly == null)
{
_logger.Trace("Adding reference to a runtime dependency => " + runtimeAssembly);
@@ -266,7 +291,7 @@ private Assembly MapUnresolvedAssemblyToRuntimeLibrary(IDictionary assemblyName.Version)
{
loadedAssemblyMap.TryGetValue(assemblyName.Name, out var loadedAssembly);
- if(loadedAssembly != null)
+ if (loadedAssembly != null)
{
_logger.Trace($"Redirecting {assemblyName} to already loaded {loadedAssembly.GetName().Name}");
return loadedAssembly;
diff --git a/src/Dotnet.Script.Core/ScriptConsole.cs b/src/Dotnet.Script.Core/ScriptConsole.cs
index 0c7ceb23..6f46bf6d 100644
--- a/src/Dotnet.Script.Core/ScriptConsole.cs
+++ b/src/Dotnet.Script.Core/ScriptConsole.cs
@@ -36,22 +36,29 @@ public virtual void WriteHighlighted(string value)
Console.ResetColor();
}
+ public virtual void WriteWarning(string value)
+ {
+ Console.ForegroundColor = ConsoleColor.Yellow;
+ Error.WriteLine(value.TrimEnd(Environment.NewLine.ToCharArray()));
+ Console.ResetColor();
+ }
+
public virtual void WriteNormal(string value)
{
Out.WriteLine(value.TrimEnd(Environment.NewLine.ToCharArray()));
}
- public virtual void WriteDiagnostics(Diagnostic[] warningDiagnostics, Diagnostic[] errorDiagnostics)
+ public virtual void WriteDiagnostics(Diagnostic[] warningDiagnostics, Diagnostic[] errorDiagnostics)
{
- if (warningDiagnostics != null)
+ if (warningDiagnostics != null)
{
foreach (var warning in warningDiagnostics)
{
- WriteHighlighted(warning.ToString());
+ WriteWarning(warning.ToString());
}
}
- if (warningDiagnostics != null)
+ if (errorDiagnostics != null)
{
foreach (var error in errorDiagnostics)
{
diff --git a/src/Dotnet.Script.Core/ScriptDownloader.cs b/src/Dotnet.Script.Core/ScriptDownloader.cs
index f22741a0..f0c62d98 100755
--- a/src/Dotnet.Script.Core/ScriptDownloader.cs
+++ b/src/Dotnet.Script.Core/ScriptDownloader.cs
@@ -11,37 +11,31 @@ public class ScriptDownloader
{
public async Task Download(string uri)
{
- using (HttpClient client = new HttpClient(new HttpClientHandler
+ using HttpClient client = new HttpClient(new HttpClientHandler
{
// Avoid Deflate due to bugs. For more info, see:
// https://github.com/weblinq/WebLinq/issues/132
AutomaticDecompression = DecompressionMethods.GZip
- }))
- {
- using (HttpResponseMessage response = await client.GetAsync(uri, HttpCompletionOption.ResponseHeadersRead))
- {
- response.EnsureSuccessStatusCode();
+ });
+ using HttpResponseMessage response = await client.GetAsync(uri, HttpCompletionOption.ResponseHeadersRead);
+ response.EnsureSuccessStatusCode();
- using (HttpContent content = response.Content)
- {
- var mediaType = content.Headers.ContentType?.MediaType?.ToLowerInvariant().Trim();
- switch (mediaType)
- {
- case null:
- case "":
- case "text/plain":
- return await content.ReadAsStringAsync();
- case "application/gzip":
- case "application/x-gzip":
- using (var stream = await content.ReadAsStreamAsync())
- using (var gzip = new GZipStream(stream, CompressionMode.Decompress))
- using (var reader = new StreamReader(gzip))
- return await reader.ReadToEndAsync();
- default:
- throw new NotSupportedException($"The media type '{mediaType}' is not supported when executing a script over http/https");
- }
- }
- }
+ using HttpContent content = response.Content;
+ var mediaType = content.Headers.ContentType?.MediaType?.ToLowerInvariant().Trim();
+ switch (mediaType)
+ {
+ case null:
+ case "":
+ case "text/plain":
+ return await content.ReadAsStringAsync();
+ case "application/gzip":
+ case "application/x-gzip":
+ using (var stream = await content.ReadAsStreamAsync())
+ using (var gzip = new GZipStream(stream, CompressionMode.Decompress))
+ using (var reader = new StreamReader(gzip))
+ return await reader.ReadToEndAsync();
+ default:
+ throw new NotSupportedException($"The media type '{mediaType}' is not supported when executing a script over http/https");
}
}
}
diff --git a/src/Dotnet.Script.Core/ScriptEmitter.cs b/src/Dotnet.Script.Core/ScriptEmitter.cs
index 8f8e133d..c0e10e16 100644
--- a/src/Dotnet.Script.Core/ScriptEmitter.cs
+++ b/src/Dotnet.Script.Core/ScriptEmitter.cs
@@ -23,7 +23,7 @@ public virtual ScriptEmitResult Emit(ScriptContext context, stri
var compilationContext = _scriptCompiler.CreateCompilationContext(context);
foreach (var warning in compilationContext.Warnings)
{
- _scriptConsole.WriteHighlighted(warning.ToString());
+ _scriptConsole.WriteWarning(warning.ToString());
}
if (compilationContext.Errors.Any())
diff --git a/src/Dotnet.Script.Core/ScriptPublisher.cs b/src/Dotnet.Script.Core/ScriptPublisher.cs
index ed655fd8..faaf6b05 100644
--- a/src/Dotnet.Script.Core/ScriptPublisher.cs
+++ b/src/Dotnet.Script.Core/ScriptPublisher.cs
@@ -11,7 +11,7 @@ namespace Dotnet.Script.Core
{
public class ScriptPublisher
{
- private const string ScriptingVersion = "3.9.0";
+ private const string ScriptingVersion = "4.11.0";
private readonly ScriptProjectProvider _scriptProjectProvider;
private readonly ScriptEmitter _scriptEmitter;
@@ -57,7 +57,7 @@ public void CreateAssembly(ScriptContext context, LogFactory log
// only display published if we aren't auto publishing to temp folder
if (!scriptAssemblyPath.StartsWith(FileUtils.GetTempPath()))
{
- _scriptConsole.WriteSuccess($"Published {context.FilePath} to { scriptAssemblyPath}");
+ _scriptConsole.WriteSuccess($"Published {context.FilePath} to {scriptAssemblyPath}");
}
}
@@ -68,7 +68,7 @@ public void CreateExecutable(ScriptContext context, LogFactory l
throw new ArgumentNullException(nameof(runtimeIdentifier));
}
- executableFileName = executableFileName ?? Path.GetFileNameWithoutExtension(context.FilePath);
+ executableFileName ??= Path.GetFileNameWithoutExtension(context.FilePath);
const string AssemblyName = "scriptAssembly";
var tempProjectPath = ScriptProjectProvider.GetPathToProjectFile(Path.GetDirectoryName(context.FilePath), _scriptEnvironment.TargetFramework);
diff --git a/src/Dotnet.Script.Core/ScriptRunner.cs b/src/Dotnet.Script.Core/ScriptRunner.cs
index c9e23be7..66b605fe 100644
--- a/src/Dotnet.Script.Core/ScriptRunner.cs
+++ b/src/Dotnet.Script.Core/ScriptRunner.cs
@@ -3,10 +3,14 @@
using System.Collections.Immutable;
using System.Linq;
using System.Reflection;
+#if NETCOREAPP
+using System.Runtime.Loader;
+#endif
using System.Threading.Tasks;
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Logging;
using Dotnet.Script.DependencyModel.Runtime;
+using Gapotchenko.FX.Reflection;
using Microsoft.CodeAnalysis.CSharp.Scripting.Hosting;
using Microsoft.CodeAnalysis.Scripting;
using Microsoft.CodeAnalysis.Scripting.Hosting;
@@ -28,36 +32,100 @@ public ScriptRunner(ScriptCompiler scriptCompiler, LogFactory logFactory, Script
_scriptEnvironment = ScriptEnvironment.Default;
}
+#if NETCOREAPP
+#nullable enable
+ ///
+ /// Gets or sets a custom assembly load context to use for script execution.
+ ///
+ public AssemblyLoadContext? AssemblyLoadContext { get; init; }
+#nullable restore
+#endif
+
public async Task Execute(string dllPath, IEnumerable commandLineArgs)
{
+#if NETCOREAPP
+ var assemblyLoadContext = AssemblyLoadContext;
+ var assemblyLoadPal = assemblyLoadContext != null ? new AssemblyLoadPal(assemblyLoadContext) : AssemblyLoadPal.ForCurrentAppDomain;
+#else
+ var assemblyLoadPal = AssemblyLoadPal.ForCurrentAppDomain;
+#endif
+
var runtimeDeps = ScriptCompiler.RuntimeDependencyResolver.GetDependenciesForLibrary(dllPath);
var runtimeDepsMap = ScriptCompiler.CreateScriptDependenciesMap(runtimeDeps);
- var assembly = Assembly.LoadFrom(dllPath); // this needs to be called prior to 'AppDomain.CurrentDomain.AssemblyResolve' event handler added
+ var assembly = assemblyLoadPal.LoadFrom(dllPath); // this needs to be called prior to 'AssemblyLoadPal.Resolving' event handler added
- AppDomain.CurrentDomain.AssemblyResolve += (sender, args) => ResolveAssembly(args, runtimeDepsMap);
+#if NETCOREAPP
+ using var assemblyAutoLoader = assemblyLoadContext != null ? new AssemblyAutoLoader(assemblyLoadContext) : null;
+ assemblyAutoLoader?.AddAssembly(assembly);
- var type = assembly.GetType("Submission#0");
- var method = type.GetMethod("", BindingFlags.Static | BindingFlags.Public);
+ Assembly OnLoading(ScriptAssemblyLoadContext sender, ScriptAssemblyLoadContext.LoadingEventArgs args)
+ {
+ var assemblyName = args.Name;
- var globals = new CommandLineScriptGlobals(ScriptConsole.Out, CSharpObjectFormatter.Instance);
- foreach (var arg in commandLineArgs)
- globals.Args.Add(arg);
+ if (sender.IsHomogeneousAssembly(assemblyName))
+ {
+ // The default assembly loader will take care of it.
+ return null;
+ }
- var submissionStates = new object[2];
- submissionStates[0] = globals;
+ return ResolveAssembly(assemblyLoadPal, assemblyName, runtimeDepsMap);
+ }
- var resultTask = method.Invoke(null, new[] { submissionStates }) as Task;
- try
+ IntPtr OnLoadingUnmanagedDll(ScriptAssemblyLoadContext sender, ScriptAssemblyLoadContext.LoadingUnmanagedDllEventArgs args)
{
- _ = await resultTask;
+ string dllPath = assemblyAutoLoader.ResolveUnmanagedDllPath(args.UnmanagedDllName);
+ if (dllPath == null)
+ return IntPtr.Zero;
+ return args.LoadUnmanagedDllFromPath(dllPath);
}
- catch (System.Exception ex)
+
+ var scriptAssemblyLoadContext = assemblyLoadContext as ScriptAssemblyLoadContext;
+ if (scriptAssemblyLoadContext != null)
{
- ScriptConsole.WriteError(ex.ToString());
- throw new ScriptRuntimeException("Script execution resulted in an exception.", ex);
+ scriptAssemblyLoadContext.Loading += OnLoading;
+ scriptAssemblyLoadContext.LoadingUnmanagedDll += OnLoadingUnmanagedDll;
}
- return await resultTask;
+ using var contextualReflectionScope = assemblyLoadContext != null ? assemblyLoadContext.EnterContextualReflection() : default;
+#endif
+
+ Assembly OnResolving(AssemblyLoadPal sender, AssemblyLoadPal.ResolvingEventArgs args) => ResolveAssembly(sender, args.Name, runtimeDepsMap);
+
+ assemblyLoadPal.Resolving += OnResolving;
+ try
+ {
+ var type = assembly.GetType("Submission#0");
+ var method = type.GetMethod("", BindingFlags.Static | BindingFlags.Public);
+
+ var globals = new CommandLineScriptGlobals(ScriptConsole.Out, CSharpObjectFormatter.Instance);
+ foreach (var arg in commandLineArgs)
+ globals.Args.Add(arg);
+
+ var submissionStates = new object[2];
+ submissionStates[0] = globals;
+
+ try
+ {
+ var resultTask = (Task)method.Invoke(null, new[] { submissionStates });
+ return await resultTask;
+ }
+ catch (System.Exception ex)
+ {
+ ScriptConsole.WriteError(ex.ToString());
+ throw new ScriptRuntimeException("Script execution resulted in an exception.", ex);
+ }
+ }
+ finally
+ {
+ assemblyLoadPal.Resolving -= OnResolving;
+#if NETCOREAPP
+ if (scriptAssemblyLoadContext != null)
+ {
+ scriptAssemblyLoadContext.LoadingUnmanagedDll -= OnLoadingUnmanagedDll;
+ scriptAssemblyLoadContext.Loading -= OnLoading;
+ }
+#endif
+ }
}
public Task Execute(ScriptContext context)
@@ -89,12 +157,11 @@ public virtual async Task Execute(ScriptCompilationCont
return ProcessScriptState(scriptResult);
}
- internal Assembly ResolveAssembly(ResolveEventArgs args, Dictionary runtimeDepsMap)
+ internal Assembly ResolveAssembly(AssemblyLoadPal pal, AssemblyName assemblyName, Dictionary runtimeDepsMap)
{
- var assemblyName = new AssemblyName(args.Name);
var result = runtimeDepsMap.TryGetValue(assemblyName.Name, out RuntimeAssembly runtimeAssembly);
if (!result) return null;
- var loadedAssembly = Assembly.LoadFrom(runtimeAssembly.Path);
+ var loadedAssembly = pal.LoadFrom(runtimeAssembly.Path);
return loadedAssembly;
}
diff --git a/src/Dotnet.Script.Core/Templates/TemplateLoader.cs b/src/Dotnet.Script.Core/Templates/TemplateLoader.cs
index 593f932c..e4bee252 100644
--- a/src/Dotnet.Script.Core/Templates/TemplateLoader.cs
+++ b/src/Dotnet.Script.Core/Templates/TemplateLoader.cs
@@ -8,10 +8,8 @@ public static class TemplateLoader
public static string ReadTemplate(string name)
{
var resourceStream = typeof(TemplateLoader).GetTypeInfo().Assembly.GetManifestResourceStream($"Dotnet.Script.Core.Templates.{name}");
- using (var streamReader = new StreamReader(resourceStream))
- {
- return streamReader.ReadToEnd();
- }
+ using var streamReader = new StreamReader(resourceStream);
+ return streamReader.ReadToEnd();
}
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Templates/globaltool.launch.json.template b/src/Dotnet.Script.Core/Templates/globaltool.launch.json.template
index cf76da42..305245ba 100644
--- a/src/Dotnet.Script.Core/Templates/globaltool.launch.json.template
+++ b/src/Dotnet.Script.Core/Templates/globaltool.launch.json.template
@@ -11,7 +11,7 @@
"program": "${env:USERPROFILE}/.dotnet/tools/dotnet-script.exe",
},
"cwd": "${workspaceFolder}",
- "stopAtEntry": true,
+ "stopAtEntry": false,
}
]
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Templates/launch.json.template b/src/Dotnet.Script.Core/Templates/launch.json.template
index 24492703..9b4b1c3b 100644
--- a/src/Dotnet.Script.Core/Templates/launch.json.template
+++ b/src/Dotnet.Script.Core/Templates/launch.json.template
@@ -12,7 +12,7 @@
"${file}"
],
"cwd": "${workspaceRoot}",
- "stopAtEntry": true
+ "stopAtEntry": false
}
]
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Core/Versioning/EnvironmentReporter.cs b/src/Dotnet.Script.Core/Versioning/EnvironmentReporter.cs
index b641a727..3155d5f9 100644
--- a/src/Dotnet.Script.Core/Versioning/EnvironmentReporter.cs
+++ b/src/Dotnet.Script.Core/Versioning/EnvironmentReporter.cs
@@ -78,11 +78,11 @@ private void ReportThatNewVersionIsAvailable(VersionInfo latestVersion)
if (ScriptEnvironment.Default.IsWindows)
{
updateInfo.AppendLine("Chocolatey : choco upgrade Dotnet.Script");
- updateInfo.AppendLine("Powershell : (new-object Net.WebClient).DownloadString(\"https://raw.githubusercontent.com/filipw/dotnet-script/master/install/install.ps\") | iex");
+ updateInfo.AppendLine("Powershell : (new-object Net.WebClient).DownloadString(\"https://raw.githubusercontent.com/dotnet-script/dotnet-script/master/install/install.ps\") | iex");
}
else
{
- updateInfo.AppendLine("Bash : curl -s https://raw.githubusercontent.com/filipw/dotnet-script/master/install/install.sh | bash");
+ updateInfo.AppendLine("Bash : curl -s https://raw.githubusercontent.com/dotnet-script/dotnet-script/master/install/install.sh | bash");
}
_scriptConsole.WriteHighlighted(updateInfo.ToString());
diff --git a/src/Dotnet.Script.Core/Versioning/VersionProvider.cs b/src/Dotnet.Script.Core/Versioning/VersionProvider.cs
index 11107a5e..b9f9acc6 100644
--- a/src/Dotnet.Script.Core/Versioning/VersionProvider.cs
+++ b/src/Dotnet.Script.Core/Versioning/VersionProvider.cs
@@ -2,9 +2,8 @@
using System.Linq;
using System.Net.Http;
using System.Reflection;
+using System.Text.Json.Nodes;
using System.Threading.Tasks;
-using Dotnet.Script.DependencyModel.Logging;
-using Newtonsoft.Json.Linq;
namespace Dotnet.Script.Core.Versioning
{
@@ -14,12 +13,12 @@ namespace Dotnet.Script.Core.Versioning
public class VersionProvider : IVersionProvider
{
private const string UserAgent = "dotnet-script";
- private static readonly string RequestUri = "/repos/filipw/dotnet-script/releases/latest";
+ private static readonly string RequestUri = "/repos/dotnet-script/dotnet-script/releases/latest";
- ///
+ ///
public async Task GetLatestVersion()
{
- using(var httpClient = CreateHttpClient())
+ using (var httpClient = CreateHttpClient())
{
var response = await httpClient.GetStringAsync(RequestUri);
return ParseTagName(response);
@@ -34,8 +33,8 @@ HttpClient CreateHttpClient()
VersionInfo ParseTagName(string json)
{
- JObject jsonResult = JObject.Parse(json);
- return new VersionInfo(jsonResult.SelectToken("tag_name").Value(), isResolved:true);
+ JsonNode jsonResult = JsonNode.Parse(json);
+ return new VersionInfo(jsonResult["tag_name"].GetValue(), isResolved: true);
}
}
@@ -43,7 +42,7 @@ VersionInfo ParseTagName(string json)
public VersionInfo GetCurrentVersion()
{
var versionAttribute = typeof(VersionProvider).Assembly.GetCustomAttributes().Single();
- return new VersionInfo(versionAttribute.InformationalVersion, isResolved:true);
+ return new VersionInfo(versionAttribute.InformationalVersion, isResolved: true);
}
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.DependencyModel.Nuget/Dotnet.Script.DependencyModel.NuGet.csproj b/src/Dotnet.Script.DependencyModel.Nuget/Dotnet.Script.DependencyModel.NuGet.csproj
index becaacfe..c2794916 100644
--- a/src/Dotnet.Script.DependencyModel.Nuget/Dotnet.Script.DependencyModel.NuGet.csproj
+++ b/src/Dotnet.Script.DependencyModel.Nuget/Dotnet.Script.DependencyModel.NuGet.csproj
@@ -1,14 +1,14 @@
-
-
+
+
netstandard2.0
MIT
- https://github.com/filipw/dotnet-script
- https://raw.githubusercontent.com/filipw/Strathweb.TypedRouting.AspNetCore/master/strathweb.png
- https://github.com/filipw/dotnet-script.git
+ https://github.com/dotnet-script/dotnet-script
+ https://avatars.githubusercontent.com/u/113979420
+ https://github.com/dotnet-script/dotnet-script.git
git
script;csx;csharp;roslyn;nuget
- 1.1.0
+ 1.6.0
A MetadataReferenceResolver that allows inline nuget references to be specified in script(csx) files.
dotnet-script
dotnet-script
@@ -16,11 +16,10 @@
true
../dotnet-script.snk
-
-
+
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.DependencyModel.Nuget/NuGetMetadataReferenceResolver.cs b/src/Dotnet.Script.DependencyModel.Nuget/NuGetMetadataReferenceResolver.cs
index d773d9da..cd148457 100644
--- a/src/Dotnet.Script.DependencyModel.Nuget/NuGetMetadataReferenceResolver.cs
+++ b/src/Dotnet.Script.DependencyModel.Nuget/NuGetMetadataReferenceResolver.cs
@@ -21,7 +21,7 @@ public NuGetMetadataReferenceResolver(MetadataReferenceResolver metadataReferenc
{
_metadataReferenceResolver = metadataReferenceResolver;
}
-
+
public override bool Equals(object other)
{
return _metadataReferenceResolver.Equals(other);
@@ -42,7 +42,7 @@ public override PortableExecutableReference ResolveMissingAssembly(MetadataRefer
public override ImmutableArray ResolveReference(string reference, string baseFilePath, MetadataReferenceProperties properties)
{
- if (reference.StartsWith("nuget", StringComparison.OrdinalIgnoreCase))
+ if (reference.StartsWith("nuget", StringComparison.OrdinalIgnoreCase) || reference.StartsWith("sdk", StringComparison.OrdinalIgnoreCase))
{
// HACK We need to return something here to "mark" the reference as resolved.
// https://github.com/dotnet/roslyn/blob/master/src/Compilers/Core/Portable/ReferenceManager/CommonReferenceManager.Resolution.cs#L838
diff --git a/src/Dotnet.Script.DependencyModel/Compilation/CompilationDependencyResolver.cs b/src/Dotnet.Script.DependencyModel/Compilation/CompilationDependencyResolver.cs
index 3c2f2970..136cb552 100644
--- a/src/Dotnet.Script.DependencyModel/Compilation/CompilationDependencyResolver.cs
+++ b/src/Dotnet.Script.DependencyModel/Compilation/CompilationDependencyResolver.cs
@@ -43,13 +43,8 @@ public IEnumerable GetDependencies(string targetDirectory
result.Add(compilationDependency);
}
- // On .Net Core, we need to fetch the compilation references for framework assemblies separately.
- if (defaultTargetFramework.StartsWith("netcoreapp3", StringComparison.InvariantCultureIgnoreCase) ||
- defaultTargetFramework.StartsWith("net5", StringComparison.InvariantCultureIgnoreCase))
- {
- var compilationreferences = _compilationReferenceReader.Read(projectFileInfo);
- result.Add(new CompilationDependency("Dotnet.Script.Default.Dependencies", "99.0", compilationreferences.Select(cr => cr.Path).ToArray(), Array.Empty()));
- }
+ var compilationReferences = _compilationReferenceReader.Read(projectFileInfo);
+ result.Add(new CompilationDependency("Dotnet.Script.Default.Dependencies", "99.0", compilationReferences.Select(cr => cr.Path).ToArray(), Array.Empty()));
return result;
}
diff --git a/src/Dotnet.Script.DependencyModel/Context/DotnetRestorer.cs b/src/Dotnet.Script.DependencyModel/Context/DotnetRestorer.cs
index 8b8a9f4a..b3bcf621 100644
--- a/src/Dotnet.Script.DependencyModel/Context/DotnetRestorer.cs
+++ b/src/Dotnet.Script.DependencyModel/Context/DotnetRestorer.cs
@@ -1,4 +1,5 @@
using Dotnet.Script.DependencyModel.Environment;
+using Dotnet.Script.DependencyModel.Internal;
using Dotnet.Script.DependencyModel.Logging;
using Dotnet.Script.DependencyModel.Process;
using Dotnet.Script.DependencyModel.ProjectSystem;
@@ -44,7 +45,7 @@ string CreatePackageSourcesArguments()
{
return packageSources.Length == 0
? string.Empty
- : packageSources.Select(s => $"-s {s}")
+ : packageSources.Select(s => $"-s {CommandLine.EscapeArgument(s)}")
.Aggregate((current, next) => $"{current} {next}");
}
diff --git a/src/Dotnet.Script.DependencyModel/Context/ScriptDependencyContextReader.cs b/src/Dotnet.Script.DependencyModel/Context/ScriptDependencyContextReader.cs
index 5d7d1558..cd78b179 100644
--- a/src/Dotnet.Script.DependencyModel/Context/ScriptDependencyContextReader.cs
+++ b/src/Dotnet.Script.DependencyModel/Context/ScriptDependencyContextReader.cs
@@ -7,12 +7,11 @@
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Logging;
using Dotnet.Script.DependencyModel.ScriptPackage;
-using Microsoft.DotNet.PlatformAbstractions;
using NuGet.Common;
using NuGet.Packaging;
using NuGet.ProjectModel;
-using NuGet.RuntimeModel;
using NuGet.Versioning;
+using Newtonsoft.Json.Linq;
namespace Dotnet.Script.DependencyModel.Context
{
@@ -37,9 +36,11 @@ public ScriptDependencyContextReader(LogFactory logFactory)
public ScriptDependencyContext ReadDependencyContext(string pathToAssetsFile)
{
var lockFile = GetLockFile(pathToAssetsFile);
+
// Since we execute "dotnet restore -r [rid]" we get two targets in the lock file.
// The second target is the one containing the runtime deps for the given RID.
var target = GetLockFileTarget(lockFile);
+
var targetLibraries = target.Libraries;
var packageFolders = lockFile.PackageFolders.Select(lfi => lfi.Path).ToArray();
var userPackageFolder = packageFolders.First();
@@ -66,10 +67,59 @@ public ScriptDependencyContext ReadDependencyContext(string pathToAssetsFile)
var netcoreAppRuntimeAssemblies = Directory.GetFiles(netcoreAppRuntimeAssemblyLocation, "*.dll").Where(IsAssembly).ToArray();
var netCoreAppDependency = new ScriptDependency("Microsoft.NETCore.App", ScriptEnvironment.Default.NetCoreVersion.Version, netcoreAppRuntimeAssemblies, Array.Empty(), Array.Empty(), Array.Empty());
scriptDependencies.Add(netCoreAppDependency);
+ if (HasAspNetCoreFrameworkReference(pathToAssetsFile))
+ {
+ var aspNetCoreRuntimeInfo = GetAspNetCoreRuntimeInfo(netcoreAppRuntimeAssemblyLocation);
+ var aspNetCoreAppRuntimeAssemblies = Directory.GetFiles(aspNetCoreRuntimeInfo.aspNetCoreRuntimeAssemblyLocation, "*.dll").Where(IsAssembly).ToArray();
+ var aspNetCoreAppDependency = new ScriptDependency("Microsoft.AspNetCore.App", aspNetCoreRuntimeInfo.aspNetCoreVersion, aspNetCoreAppRuntimeAssemblies, Array.Empty(), Array.Empty(), Array.Empty());
+ scriptDependencies.Add(aspNetCoreAppDependency);
+ }
}
return new ScriptDependencyContext(scriptDependencies.ToArray());
}
+ private bool HasAspNetCoreFrameworkReference(string pathToAssetsFile)
+ {
+ JObject assetsFile = JObject.Parse(File.ReadAllText(pathToAssetsFile));
+ return assetsFile["project"]?["frameworks"]?[ScriptEnvironment.Default.TargetFramework]?["frameworkReferences"]?["Microsoft.AspNetCore.App"] != null;
+ }
+
+ private static (string aspNetCoreRuntimeAssemblyLocation, string aspNetCoreVersion) GetAspNetCoreRuntimeInfo(string netcoreAppRuntimeAssemblyLocation)
+ {
+ var netCoreAppRuntimeVersion = Path.GetFileName(netcoreAppRuntimeAssemblyLocation);
+ if (!SemanticVersion.TryParse(netCoreAppRuntimeVersion, out var version))
+ {
+ throw new InvalidOperationException($"Unable to parse netcore app version '{netCoreAppRuntimeVersion}'");
+ }
+ var pathToSharedFolder = Path.GetFullPath(Path.Combine(netcoreAppRuntimeAssemblyLocation, "..", ".."));
+
+ var pathToAspNetCoreRuntimeFolder = Directory.GetDirectories(pathToSharedFolder, "Microsoft.AspNetCore.App", SearchOption.TopDirectoryOnly).SingleOrDefault();
+ if (string.IsNullOrWhiteSpace(pathToAspNetCoreRuntimeFolder))
+ {
+ throw new InvalidOperationException($"Failed to resolve the path to 'Microsoft.AspNetCore.App' in {pathToSharedFolder}");
+ }
+
+ var aspNetCoreVersionsFolders = Directory.GetDirectories(pathToAspNetCoreRuntimeFolder).Select(folder => Path.GetFileName(folder));
+
+ var aspNetCoreVersions = new List();
+ foreach (var aspNetCoreVersionsFolder in aspNetCoreVersionsFolders)
+ {
+ if (!SemanticVersion.TryParse(aspNetCoreVersionsFolder, out var aspNetCoreVersion))
+ {
+ throw new InvalidOperationException($"Unable to parse Asp.Net version {aspNetCoreVersionsFolder}");
+ }
+ else
+ {
+ aspNetCoreVersions.Add(aspNetCoreVersion);
+ }
+ }
+
+ var latestAspNetCoreVersion = aspNetCoreVersions.Where(v => v.Major == version.Major).OrderBy(v => v).Last();
+
+ return (Path.Combine(pathToAspNetCoreRuntimeFolder, latestAspNetCoreVersion.ToNormalizedString()), latestAspNetCoreVersion.ToNormalizedString());
+ }
+
+
private static bool IsAssembly(string file)
{
// https://docs.microsoft.com/en-us/dotnet/standard/assembly/identify
@@ -138,7 +188,7 @@ private string[] GetScriptPaths(FallbackPackagePathResolver packagePathResolver,
private string[] GetNativeAssetPaths(FallbackPackagePathResolver packagePathResolver, LockFileTargetLibrary targetLibrary)
{
- List nativeAssetPaths = new List();
+ var nativeAssetPaths = new List();
foreach (var runtimeTarget in targetLibrary.NativeLibraries.Where(lfi => !lfi.Path.EndsWith("_._")))
{
var fullPath = ResolveFullPath(packagePathResolver, targetLibrary.Name, targetLibrary.Version.ToString(), runtimeTarget.Path);
@@ -150,7 +200,7 @@ private string[] GetNativeAssetPaths(FallbackPackagePathResolver packagePathReso
private static string[] GetRuntimeDependencyPaths(FallbackPackagePathResolver packagePathResolver, LockFileTargetLibrary targetLibrary)
{
- List runtimeDependencyPaths = new List();
+ var runtimeDependencyPaths = new List();
foreach (var lockFileItem in targetLibrary.RuntimeAssemblies.Where(lfi => !lfi.Path.EndsWith("_._")))
{
diff --git a/src/Dotnet.Script.DependencyModel/Dotnet.Script.DependencyModel.csproj b/src/Dotnet.Script.DependencyModel/Dotnet.Script.DependencyModel.csproj
index c1141c7b..a0be1fb6 100644
--- a/src/Dotnet.Script.DependencyModel/Dotnet.Script.DependencyModel.csproj
+++ b/src/Dotnet.Script.DependencyModel/Dotnet.Script.DependencyModel.csproj
@@ -1,39 +1,34 @@
-
-
+
+
netstandard2.0
dotnet-script
dotnet-script
Provides runtime and compilation dependency resolution for dotnet-script based scripts.
MIT
- https://github.com/filipw/dotnet-script
- https://raw.githubusercontent.com/filipw/Strathweb.TypedRouting.AspNetCore/master/strathweb.png
- https://github.com/filipw/dotnet-script.git
+ https://github.com/dotnet-script/dotnet-script
+ https://avatars.githubusercontent.com/u/113979420
+ https://github.com/dotnet-script/dotnet-script.git
git
script;csx;csharp;roslyn;omnisharp
- 1.1.0
+ 1.6.0
latest
true
../dotnet-script.snk
-
-
ScriptParser.cs
-
-
-
+
-
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.DependencyModel/Environment/ScriptEnvironment.cs b/src/Dotnet.Script.DependencyModel/Environment/ScriptEnvironment.cs
index afb6591e..2b6de442 100644
--- a/src/Dotnet.Script.DependencyModel/Environment/ScriptEnvironment.cs
+++ b/src/Dotnet.Script.DependencyModel/Environment/ScriptEnvironment.cs
@@ -133,10 +133,16 @@ private static string GetProcessArchitecture()
private static string GetRuntimeIdentifier()
{
var platformIdentifier = GetPlatformIdentifier();
+
+#if NET8_0
+ return $"{platformIdentifier}-{GetProcessArchitecture()}";
+#endif
+
if (platformIdentifier == "osx" || platformIdentifier == "linux")
{
return $"{platformIdentifier}-{GetProcessArchitecture()}";
}
+
var runtimeIdentifier = RuntimeEnvironment.GetRuntimeIdentifier();
return runtimeIdentifier;
}
diff --git a/src/Dotnet.Script.DependencyModel/Internal/CommandLine.cs b/src/Dotnet.Script.DependencyModel/Internal/CommandLine.cs
new file mode 100644
index 00000000..d7a037ba
--- /dev/null
+++ b/src/Dotnet.Script.DependencyModel/Internal/CommandLine.cs
@@ -0,0 +1,93 @@
+using System;
+using System.Text;
+using System.Text.RegularExpressions;
+
+namespace Dotnet.Script.DependencyModel.Internal
+{
+ ///
+ ///
+ /// Performs operations on instances that contain command line information.
+ ///
+ ///
+ /// Tip: a ready-to-use package with this functionality is available at https://www.nuget.org/packages/Gapotchenko.FX.Diagnostics.CommandLine.
+ ///
+ ///
+ ///
+ /// Available
+ ///
+ static class CommandLine
+ {
+ ///
+ /// Escapes and optionally quotes a command line argument.
+ ///
+ /// The command line argument.
+ /// The escaped and optionally quoted command line argument.
+ public static string EscapeArgument(string value)
+ {
+ if (value == null)
+ return null;
+
+ int length = value.Length;
+ if (length == 0)
+ return string.Empty;
+
+ var sb = new StringBuilder();
+ Escape.AppendQuotedText(sb, value);
+
+ if (sb.Length == length)
+ return value;
+
+ return sb.ToString();
+ }
+
+ static class Escape
+ {
+ public static void AppendQuotedText(StringBuilder sb, string text)
+ {
+ bool quotingRequired = IsQuotingRequired(text);
+ if (quotingRequired)
+ sb.Append('"');
+
+ int numberOfQuotes = 0;
+ for (int i = 0; i < text.Length; i++)
+ {
+ if (text[i] == '"')
+ numberOfQuotes++;
+ }
+
+ if (numberOfQuotes > 0)
+ {
+ if ((numberOfQuotes % 2) != 0)
+ throw new Exception("Command line parameter cannot contain an odd number of double quotes.");
+ text = text.Replace("\\\"", "\\\\\"").Replace("\"", "\\\"");
+ }
+
+ sb.Append(text);
+
+ if (quotingRequired && text.EndsWith("\\"))
+ sb.Append('\\');
+
+ if (quotingRequired)
+ sb.Append('"');
+ }
+
+ static bool IsQuotingRequired(string parameter) =>
+ !AllowedUnquotedRegex.IsMatch(parameter) ||
+ DefinitelyNeedQuotesRegex.IsMatch(parameter);
+
+ static Regex m_CachedAllowedUnquotedRegex;
+
+ static Regex AllowedUnquotedRegex =>
+ m_CachedAllowedUnquotedRegex ??= new Regex(
+ @"^[a-z\\/:0-9\._\-+=]*$",
+ RegexOptions.IgnoreCase | RegexOptions.CultureInvariant);
+
+ static Regex m_CachedDefinitelyNeedQuotesRegex;
+
+ static Regex DefinitelyNeedQuotesRegex =>
+ m_CachedDefinitelyNeedQuotesRegex ??= new Regex(
+ "[|><\\s,;\"]+",
+ RegexOptions.CultureInvariant);
+ }
+ }
+}
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/FileUtils.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/FileUtils.cs
index aa52c14a..b23a0d1a 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/FileUtils.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/FileUtils.cs
@@ -1,9 +1,9 @@
-using Dotnet.Script.DependencyModel.Environment;
-using System;
+using System;
using System.Collections.Generic;
using System.IO;
using System.Runtime.InteropServices;
using System.Text;
+using Dotnet.Script.DependencyModel.Environment;
using SysEnvironment = System.Environment;
namespace Dotnet.Script.DependencyModel.ProjectSystem
@@ -51,8 +51,14 @@ public static string GetTempPath()
{
// prefer the custom env variable if set
var cachePath = SysEnvironment.GetEnvironmentVariable("DOTNET_SCRIPT_CACHE_LOCATION");
+
if (!string.IsNullOrEmpty(cachePath))
{
+ // if the path is not absolute, make it relative to the current folder
+ if (!Path.IsPathRooted(cachePath))
+ {
+ cachePath = Path.Combine(Directory.GetCurrentDirectory(), cachePath);
+ }
return cachePath;
}
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ParseResult.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ParseResult.cs
index a097e422..fc2a0924 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ParseResult.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ParseResult.cs
@@ -10,5 +10,7 @@ public ParseResult(IReadOnlyCollection packageReferences)
}
public IReadOnlyCollection PackageReferences { get; }
+
+ public string Sdk { get; set; }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ProjectFile.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ProjectFile.cs
index be69b326..35738a71 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ProjectFile.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ProjectFile.cs
@@ -39,6 +39,8 @@ public ProjectFile(string xmlContent)
{
AssemblyReferences.Add(new AssemblyReference(assemblyReference.Attribute("Include").Value));
}
+
+ Sdk = projectFileDocument.Descendants("Project").Single().Attributes("Sdk").Single().Value;
}
///
@@ -61,9 +63,20 @@ public ProjectFile(string xmlContent)
///
public string TargetFramework { get; set; } = ScriptEnvironment.Default.TargetFramework;
+ ///
+ /// Gets the project SDK
+ ///
+ public string Sdk { get; set; } = "Microsoft.NET.Sdk";
+
public void Save(string pathToProjectFile)
{
var projectFileDocument = XDocument.Parse(ReadTemplate("csproj.template"));
+ if (!string.IsNullOrEmpty(Sdk))
+ {
+ var projectElement = projectFileDocument.Descendants("Project").Single();
+ projectElement.Attributes("Sdk").Single().Value = Sdk;
+ }
+
var itemGroupElement = projectFileDocument.Descendants("ItemGroup").Single();
foreach (var packageReference in PackageReferences)
{
@@ -83,19 +96,15 @@ public void Save(string pathToProjectFile)
var targetFrameworkElement = projectFileDocument.Descendants("TargetFramework").Single();
targetFrameworkElement.Value = TargetFramework;
- using (var fileStream = new FileStream(pathToProjectFile, FileMode.Create, FileAccess.Write))
- {
- projectFileDocument.Save(fileStream);
- }
+ using var fileStream = new FileStream(pathToProjectFile, FileMode.Create, FileAccess.Write);
+ projectFileDocument.Save(fileStream);
}
private static string ReadTemplate(string name)
{
var resourceStream = typeof(ProjectFile).GetTypeInfo().Assembly.GetManifestResourceStream($"Dotnet.Script.DependencyModel.ProjectSystem.{name}");
- using (var streamReader = new StreamReader(resourceStream))
- {
- return streamReader.ReadToEnd();
- }
+ using var streamReader = new StreamReader(resourceStream);
+ return streamReader.ReadToEnd();
}
///
@@ -105,7 +114,8 @@ public bool Equals(ProjectFile other)
if (ReferenceEquals(this, other)) return true;
return PackageReferences.SequenceEqual(other.PackageReferences)
&& AssemblyReferences.SequenceEqual(other.AssemblyReferences)
- && TargetFramework.Equals(other.TargetFramework);
+ && TargetFramework.Equals(other.TargetFramework)
+ && Sdk.Equals(other.Sdk);
}
///
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptFilesResolver.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptFilesResolver.cs
index 16d2119d..740acbad 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptFilesResolver.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptFilesResolver.cs
@@ -61,7 +61,7 @@ private void Process(string csxFile, HashSet result)
private static string[] GetLoadDirectives(string content)
{
- var matches = Regex.Matches(content, @"^\s*#load\s*""\s*(.+)\s*""", RegexOptions.Multiline);
+ var matches = Regex.Matches(content, @"^\s*#load\s*""\s*(.+?)\s*""", RegexOptions.Multiline);
List result = new List();
foreach (var match in matches.Cast())
{
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParser.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParser.cs
index b6a8e4b5..9222a220 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParser.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParser.cs
@@ -1,4 +1,5 @@
-using System.Collections.Generic;
+using System;
+using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text.RegularExpressions;
@@ -20,32 +21,59 @@ public ParseResult ParseFromCode(string code)
var allPackageReferences = new HashSet();
allPackageReferences.UnionWith(ReadPackageReferencesFromReferenceDirective(code));
allPackageReferences.UnionWith(ReadPackageReferencesFromLoadDirective(code));
- return new ParseResult(allPackageReferences);
+ var sdkReference = ReadSdkFromReferenceDirective(code);
+ string sdk = string.Empty;
+ if (!string.IsNullOrWhiteSpace(sdkReference))
+ {
+ sdk = sdkReference;
+ }
+ return new ParseResult(allPackageReferences) { Sdk = sdk };
}
public ParseResult ParseFromFiles(IEnumerable csxFiles)
{
var allPackageReferences = new HashSet();
+ string sdk = string.Empty;
foreach (var csxFile in csxFiles)
{
_logger.Debug($"Parsing {csxFile}");
var fileContent = File.ReadAllText(csxFile);
allPackageReferences.UnionWith(ReadPackageReferencesFromReferenceDirective(fileContent));
allPackageReferences.UnionWith(ReadPackageReferencesFromLoadDirective(fileContent));
+ var sdkReference = ReadSdkFromReferenceDirective(fileContent);
+ if (!string.IsNullOrWhiteSpace(sdkReference))
+ {
+ sdk = sdkReference;
+ }
}
- return new ParseResult(allPackageReferences);
+ return new ParseResult(allPackageReferences) { Sdk = sdk };
+ }
+
+ private static string ReadSdkFromReferenceDirective(string fileContent)
+ {
+ const string pattern = DirectivePatternPrefix + "r" + SdkDirectivePatternSuffix;
+ var match = Regex.Match(fileContent, pattern, RegexOptions.Multiline);
+ if (match.Success)
+ {
+ var sdk = match.Groups[1].Value;
+ if (!string.Equals(sdk, "Microsoft.NET.Sdk.Web", System.StringComparison.InvariantCultureIgnoreCase))
+ {
+ throw new NotSupportedException($"The sdk '{sdk}' is not supported. Currently 'Microsoft.NET.Sdk.Web' is the only sdk supported.");
+ }
+ }
+ return match.Success ? match.Groups[1].Value : string.Empty;
}
private static IEnumerable ReadPackageReferencesFromReferenceDirective(string fileContent)
{
- const string pattern = DirectivePatternPrefix + "r" + DirectivePatternSuffix;
+ const string pattern = DirectivePatternPrefix + "r" + NuGetDirectivePatternSuffix;
return ReadPackageReferencesFromDirective(pattern, fileContent);
}
private static IEnumerable ReadPackageReferencesFromLoadDirective(string fileContent)
{
- const string pattern = DirectivePatternPrefix + "load" + DirectivePatternSuffix;
+ const string pattern = DirectivePatternPrefix + "load" + NuGetDirectivePatternSuffix;
return ReadPackageReferencesFromDirective(pattern, fileContent);
}
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParserInternal.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParserInternal.cs
index c931b700..72c3fb5c 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParserInternal.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptParserInternal.cs
@@ -7,14 +7,20 @@ partial class ScriptParser
const string Hws = @"[\x20\t]*"; // hws = horizontal whitespace
const string NuGetPattern = @"nuget:"
- // https://github.com/NuGet/docs.microsoft.com-nuget/issues/543#issue-270039223
+ // https://github.com/NuGet/docs.microsoft.com-nuget/issues/543#issue-270039223
+ Hws + @"(\w+(?:[_.-]\w+)*)"
+ @"(?:" + Hws + "," + Hws + @"(.+?))?";
+ const string SdkPattern = @"sdk:"
+ + Hws + @"(\w+(?:[_.-]\w+)*)"
+ + @"(?:" + Hws + @")?";
+
const string WholeNuGetPattern = @"^" + NuGetPattern + @"$";
const string DirectivePatternPrefix = @"^" + Hws + @"#";
- const string DirectivePatternSuffix = Hws + @"""" + NuGetPattern + @"""";
+ const string NuGetDirectivePatternSuffix = Hws + @"""" + NuGetPattern + @"""";
+
+ const string SdkDirectivePatternSuffix = Hws + @"""" + SdkPattern + @"""";
internal static bool TryParseNuGetPackageReference(string input,
out string id, out string version)
@@ -22,7 +28,7 @@ internal static bool TryParseNuGetPackageReference(string input,
bool success;
(success, id, version) =
Regex.Match(input, WholeNuGetPattern, RegexOptions.CultureInvariant | RegexOptions.IgnoreCase)
- is {} match && match.Success
+ is { } match && match.Success
? (true, match.Groups[1].Value, match.Groups[2].Value)
: default;
return success;
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptProjectProvider.cs b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptProjectProvider.cs
index 6b86b512..a050c64f 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptProjectProvider.cs
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/ScriptProjectProvider.cs
@@ -1,11 +1,8 @@
-using System;
-using System.Collections.Generic;
+using System.Collections.Generic;
using System.IO;
using System.Linq;
-using System.Text.RegularExpressions;
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Logging;
-using Dotnet.Script.DependencyModel.Process;
namespace Dotnet.Script.DependencyModel.ProjectSystem
{
@@ -14,19 +11,17 @@ public class ScriptProjectProvider
private readonly ScriptParser _scriptParser;
private readonly ScriptFilesResolver _scriptFilesResolver;
private readonly ScriptEnvironment _scriptEnvironment;
- private readonly CommandRunner _commandRunner;
private readonly Logger _logger;
- private ScriptProjectProvider(ScriptParser scriptParser, ScriptFilesResolver scriptFilesResolver, LogFactory logFactory, ScriptEnvironment scriptEnvironment, CommandRunner commandRunner)
+ private ScriptProjectProvider(ScriptParser scriptParser, ScriptFilesResolver scriptFilesResolver, LogFactory logFactory, ScriptEnvironment scriptEnvironment)
{
_logger = logFactory.CreateLogger();
_scriptParser = scriptParser;
_scriptFilesResolver = scriptFilesResolver;
_scriptEnvironment = scriptEnvironment;
- _commandRunner = commandRunner;
}
- public ScriptProjectProvider(LogFactory logFactory) : this(new ScriptParser(logFactory), new ScriptFilesResolver(), logFactory, ScriptEnvironment.Default, new CommandRunner(logFactory))
+ public ScriptProjectProvider(LogFactory logFactory) : this(new ScriptParser(logFactory), new ScriptFilesResolver(), logFactory, ScriptEnvironment.Default)
{
}
@@ -59,6 +54,7 @@ public ProjectFileInfo CreateProjectForRepl(string code, string targetDirectory,
}
projectFile.TargetFramework = defaultTargetFramework;
+ projectFile.Sdk = parseResultFromCode.Sdk;
projectFile.Save(pathToProjectFile);
@@ -118,16 +114,17 @@ private ProjectFileInfo SaveProjectFileFromScriptFiles(string targetDirectory, s
public ProjectFile CreateProjectFileFromScriptFiles(string defaultTargetFramework, string[] csxFiles)
{
- var parseresult = _scriptParser.ParseFromFiles(csxFiles);
+ var parseResult = _scriptParser.ParseFromFiles(csxFiles);
var projectFile = new ProjectFile();
- foreach (var packageReference in parseresult.PackageReferences)
+ foreach (var packageReference in parseResult.PackageReferences)
{
projectFile.PackageReferences.Add(packageReference);
}
projectFile.TargetFramework = defaultTargetFramework;
+ projectFile.Sdk = parseResult.Sdk;
return projectFile;
}
diff --git a/src/Dotnet.Script.DependencyModel/ProjectSystem/csproj.template b/src/Dotnet.Script.DependencyModel/ProjectSystem/csproj.template
index 119a3645..8bfbcffb 100644
--- a/src/Dotnet.Script.DependencyModel/ProjectSystem/csproj.template
+++ b/src/Dotnet.Script.DependencyModel/ProjectSystem/csproj.template
@@ -1,8 +1,9 @@
Exe
- netcoreapp2.1
+ net5.0
latest
+ true
diff --git a/src/Dotnet.Script.DependencyModel/ScriptPackage/ScriptFilesDependencyResolver.cs b/src/Dotnet.Script.DependencyModel/ScriptPackage/ScriptFilesDependencyResolver.cs
index 6ef4627e..642f73a3 100644
--- a/src/Dotnet.Script.DependencyModel/ScriptPackage/ScriptFilesDependencyResolver.cs
+++ b/src/Dotnet.Script.DependencyModel/ScriptPackage/ScriptFilesDependencyResolver.cs
@@ -109,9 +109,9 @@ private static IDictionary> GetScriptFilesPerTargetFramewor
if (match.Success)
{
var targetFramework = match.Groups[1].Value;
- if (!result.TryGetValue(targetFramework, out var files))
+ if (!result.TryGetValue(targetFramework, out _))
{
- files = new List();
+ var files = new List();
result.Add(targetFramework, files);
}
result[targetFramework].Add(match.Groups[0].Value);
diff --git a/src/Dotnet.Script.Desktop.Tests/CompilationDepenencyTests.cs b/src/Dotnet.Script.Desktop.Tests/CompilationDepenencyTests.cs
index ee90241a..e20e33e0 100644
--- a/src/Dotnet.Script.Desktop.Tests/CompilationDepenencyTests.cs
+++ b/src/Dotnet.Script.Desktop.Tests/CompilationDepenencyTests.cs
@@ -15,8 +15,8 @@ public CompilationDependencyTests(ITestOutputHelper testOutputHelper)
}
[Theory]
- [InlineData("netcoreapp2.1")]
- [InlineData("netcoreapp3.0")]
+ [InlineData("net6.0")]
+ [InlineData("net7.0")]
public void ShouldGetCompilationDependenciesForNetCoreApp(string targetFramework)
{
var resolver = CreateResolver();
diff --git a/src/Dotnet.Script.Desktop.Tests/Dotnet.Script.Desktop.Tests.csproj b/src/Dotnet.Script.Desktop.Tests/Dotnet.Script.Desktop.Tests.csproj
index cbe23b97..5d609576 100644
--- a/src/Dotnet.Script.Desktop.Tests/Dotnet.Script.Desktop.Tests.csproj
+++ b/src/Dotnet.Script.Desktop.Tests/Dotnet.Script.Desktop.Tests.csproj
@@ -1,32 +1,29 @@
-
-
+
+
net472
true
../dotnet-script.snk
-
-
-
+
+
+
all
runtime; build; native; contentfiles; analyzers
-
+
-
-
PreserveNewest
-
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.Extras/Dotnet.Script.Extras.csproj b/src/Dotnet.Script.Extras/Dotnet.Script.Extras.csproj
index 5dadb071..c79a5f84 100644
--- a/src/Dotnet.Script.Extras/Dotnet.Script.Extras.csproj
+++ b/src/Dotnet.Script.Extras/Dotnet.Script.Extras.csproj
@@ -1,8 +1,8 @@
-
-
+
+
Extensions and add ons to C# scripting
- 0.2.0
+ 0.3.0
netstandard2.0
Dotnet.Script.Extras
Dotnet.Script.Extras
@@ -10,10 +10,8 @@
false
false
-
-
-
+
+
-
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.Shared.Tests/Dotnet.Script.Shared.Tests.csproj b/src/Dotnet.Script.Shared.Tests/Dotnet.Script.Shared.Tests.csproj
index 2b928e99..2365de7b 100644
--- a/src/Dotnet.Script.Shared.Tests/Dotnet.Script.Shared.Tests.csproj
+++ b/src/Dotnet.Script.Shared.Tests/Dotnet.Script.Shared.Tests.csproj
@@ -1,18 +1,15 @@
+
-
netstandard2.0
true
../dotnet-script.snk
-
-
+
-
-
-
+
\ No newline at end of file
diff --git a/src/Dotnet.Script.Shared.Tests/InteractiveRunnerTestsBase.cs b/src/Dotnet.Script.Shared.Tests/InteractiveRunnerTestsBase.cs
index f6a90d74..14996185 100644
--- a/src/Dotnet.Script.Shared.Tests/InteractiveRunnerTestsBase.cs
+++ b/src/Dotnet.Script.Shared.Tests/InteractiveRunnerTestsBase.cs
@@ -17,7 +17,7 @@ public InteractiveRunnerTestsBase(ITestOutputHelper testOutputHelper)
testOutputHelper.Capture();
}
- private class InteractiveTestContext
+ protected class InteractiveTestContext
{
public InteractiveTestContext(ScriptConsole console, InteractiveRunner runner)
{
@@ -29,7 +29,7 @@ public InteractiveTestContext(ScriptConsole console, InteractiveRunner runner)
public InteractiveRunner Runner { get; }
}
- private InteractiveTestContext GetRunner(params string[] commands)
+ protected InteractiveTestContext GetRunner(params string[] commands)
{
var reader = new StringReader(string.Join(Environment.NewLine, commands));
var writer = new StringWriter();
diff --git a/src/Dotnet.Script.Shared.Tests/ProcessHelper.cs b/src/Dotnet.Script.Shared.Tests/ProcessHelper.cs
index 9a692163..4f79d77e 100644
--- a/src/Dotnet.Script.Shared.Tests/ProcessHelper.cs
+++ b/src/Dotnet.Script.Shared.Tests/ProcessHelper.cs
@@ -6,7 +6,7 @@ namespace Dotnet.Script.Shared.Tests
{
public static class ProcessHelper
{
- public static (string output, int exitcode) RunAndCaptureOutput(string fileName, string arguments, string workingDirectory = null)
+ public static ProcessResult RunAndCaptureOutput(string fileName, string arguments, string workingDirectory = null)
{
var startInfo = new ProcessStartInfo(fileName, arguments)
{
@@ -29,15 +29,17 @@ public static (string output, int exitcode) RunAndCaptureOutput(string fileName,
catch
{
Console.WriteLine($"Failed to launch '{fileName}' with args, '{arguments}'");
- return (null, -1);
+ return new ProcessResult(null, -1, null, null);
}
- var output = process.StandardOutput.ReadToEnd();
- output += process.StandardError.ReadToEnd();
+ var standardOut = process.StandardOutput.ReadToEnd().Trim();
+ var standardError = process.StandardError.ReadToEnd().Trim();
+
+ var output = standardOut + standardError;
process.WaitForExit();
- return (output.Trim(), process.ExitCode);
+ return new ProcessResult(output, process.ExitCode, standardOut, standardError);
}
}
}
diff --git a/src/Dotnet.Script.Shared.Tests/ProcessResult.cs b/src/Dotnet.Script.Shared.Tests/ProcessResult.cs
new file mode 100644
index 00000000..ddd0e8c9
--- /dev/null
+++ b/src/Dotnet.Script.Shared.Tests/ProcessResult.cs
@@ -0,0 +1,24 @@
+namespace Dotnet.Script.Shared.Tests
+{
+ public class ProcessResult
+ {
+ public ProcessResult(string output, int exitCode, string standardOut, string standardError)
+ {
+ this.Output = output;
+ this.ExitCode = exitCode;
+ this.StandardOut = standardOut;
+ this.StandardError = standardError;
+ }
+
+ public string Output { get; }
+ public int ExitCode { get; }
+ public string StandardOut { get; }
+ public string StandardError { get; }
+
+ public void Deconstruct(out string output, out int exitCode)
+ {
+ output = this.Output;
+ exitCode = this.ExitCode;
+ }
+ }
+}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Shared.Tests/TestPathUtils.cs b/src/Dotnet.Script.Shared.Tests/TestPathUtils.cs
index 921dcd24..5a6094d2 100644
--- a/src/Dotnet.Script.Shared.Tests/TestPathUtils.cs
+++ b/src/Dotnet.Script.Shared.Tests/TestPathUtils.cs
@@ -34,14 +34,14 @@ public static string GetPathToTestFixture(string fixture)
public static string GetPathToGlobalPackagesFolder()
{
- var result = ProcessHelper.RunAndCaptureOutput("dotnet", "nuget locals global-packages --list");
- var match = Regex.Match(result.output, @"^.*global-packages:\s*(.*)$");
+ var (output, _) = ProcessHelper.RunAndCaptureOutput("dotnet", "nuget locals global-packages --list");
+ var match = Regex.Match(output, @"^.*global-packages:\s*(.*)$");
return match.Groups[1].Value;
}
public static void RemovePackageFromGlobalNugetCache(string packageName)
{
- var pathToGlobalPackagesFolder = TestPathUtils.GetPathToGlobalPackagesFolder();
+ var pathToGlobalPackagesFolder = GetPathToGlobalPackagesFolder();
var pathToPackage = Directory.GetDirectories(pathToGlobalPackagesFolder).SingleOrDefault(d => d.Contains(packageName, StringComparison.OrdinalIgnoreCase));
if (pathToPackage != null)
{
diff --git a/src/Dotnet.Script.Tests/CachedRestorerTests.cs b/src/Dotnet.Script.Tests/CachedRestorerTests.cs
index 64916b55..8e5c5566 100644
--- a/src/Dotnet.Script.Tests/CachedRestorerTests.cs
+++ b/src/Dotnet.Script.Tests/CachedRestorerTests.cs
@@ -23,28 +23,26 @@ public void ShouldUseCacheWhenAllPackagedArePinned()
var restorerMock = new Mock();
var cachedRestorer = new CachedRestorer(restorerMock.Object, TestOutputHelper.CreateTestLogFactory());
- using (var projectFolder = new DisposableFolder())
- {
+ using var projectFolder = new DisposableFolder();
- var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
- var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
+ var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
- var projectFile = new ProjectFile();
- projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
- projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "3.2.1"));
- projectFile.Save(pathToProjectFile);
+ var projectFile = new ProjectFile();
+ projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
+ projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "3.2.1"));
+ projectFile.Save(pathToProjectFile);
- var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
+ var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
- Assert.True(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- restorerMock.Reset();
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
+ Assert.Contains(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
+ restorerMock.Reset();
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Never);
- Assert.True(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- }
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Never);
+ Assert.Contains(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
}
[Fact]
@@ -53,28 +51,26 @@ public void ShouldNotUseCacheWhenPackagesAreNotPinned()
var restorerMock = new Mock();
var cachedRestorer = new CachedRestorer(restorerMock.Object, TestOutputHelper.CreateTestLogFactory());
- using (var projectFolder = new DisposableFolder())
- {
- var projectFile = new ProjectFile();
- var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
- var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
+ using var projectFolder = new DisposableFolder();
+ var projectFile = new ProjectFile();
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
+ var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
- projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
- projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "3.2"));
- projectFile.Save(pathToProjectFile);
+ projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
+ projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "3.2"));
+ projectFile.Save(pathToProjectFile);
- var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
+ var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
- Assert.False(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- restorerMock.Reset();
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
+ Assert.DoesNotContain(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
+ restorerMock.Reset();
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
- Assert.False(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- }
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
+ Assert.DoesNotContain(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
}
[Fact]
@@ -83,32 +79,29 @@ public void ShouldNotCacheWhenProjectFilesAreNotEqual()
var restorerMock = new Mock();
var cachedRestorer = new CachedRestorer(restorerMock.Object, TestOutputHelper.CreateTestLogFactory());
- using (var projectFolder = new DisposableFolder())
- {
- var projectFile = new ProjectFile();
- var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
- var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
+ using var projectFolder = new DisposableFolder();
+ var projectFile = new ProjectFile();
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
+ var pathToCachedProjectFile = Path.Combine(projectFolder.Path, $"script.csproj.cache");
- projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
- projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "1.2.3"));
- projectFile.Save(pathToProjectFile);
+ projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
+ projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "1.2.3"));
+ projectFile.Save(pathToProjectFile);
- var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
+ var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
- Assert.True(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- restorerMock.Reset();
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
+ Assert.Contains(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
+ restorerMock.Reset();
- projectFile.PackageReferences.Add(new PackageReference("YetAnotherPackage", "1.2.3"));
- projectFile.Save(pathToProjectFile);
- cachedRestorer.Restore(projectFileInfo, NoPackageSources);
+ projectFile.PackageReferences.Add(new PackageReference("YetAnotherPackage", "1.2.3"));
+ projectFile.Save(pathToProjectFile);
+ cachedRestorer.Restore(projectFileInfo, NoPackageSources);
- restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
- Assert.True(Directory.GetFiles(projectFolder.Path).Contains(pathToCachedProjectFile));
- }
+ restorerMock.Verify(m => m.Restore(projectFileInfo, NoPackageSources), Times.Once);
+ Assert.Contains(pathToCachedProjectFile, Directory.GetFiles(projectFolder.Path));
}
-
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/Commands/ExecuteInteractiveCommandTests.cs b/src/Dotnet.Script.Tests/Commands/ExecuteInteractiveCommandTests.cs
index 252b753d..9a86ea32 100644
--- a/src/Dotnet.Script.Tests/Commands/ExecuteInteractiveCommandTests.cs
+++ b/src/Dotnet.Script.Tests/Commands/ExecuteInteractiveCommandTests.cs
@@ -16,7 +16,7 @@ public ExecuteInteractiveCommandTests(ITestOutputHelper testOutputHelper)
testOutputHelper.Capture();
}
- private (ExecuteInteractiveCommand Command, ScriptConsole Console) GetExecuteInteractiveCommand(string[] commands)
+ private static (ExecuteInteractiveCommand Command, ScriptConsole Console) GetExecuteInteractiveCommand(string[] commands)
{
var reader = new StringReader(string.Join(Environment.NewLine, commands));
var writer = new StringWriter();
diff --git a/src/Dotnet.Script.Tests/CompilationDependencyResolverTests.cs b/src/Dotnet.Script.Tests/CompilationDependencyResolverTests.cs
index 5e3652d9..17be547a 100644
--- a/src/Dotnet.Script.Tests/CompilationDependencyResolverTests.cs
+++ b/src/Dotnet.Script.Tests/CompilationDependencyResolverTests.cs
@@ -72,6 +72,16 @@ public void ShouldGetCompilationDependenciesForIssue129()
Assert.Contains(dependencies, d => d.Name == "Auth0.ManagementApi");
}
+ [Fact]
+ public void ShouldGetCompilationDependenciesForWebSdk()
+ {
+ var resolver = CreateResolver();
+ var targetDirectory = TestPathUtils.GetPathToTestFixtureFolder("WebApi");
+ var csxFiles = Directory.GetFiles(targetDirectory, "*.csx");
+ var dependencies = resolver.GetDependencies(targetDirectory, csxFiles, true, _scriptEnvironment.TargetFramework);
+ Assert.Contains(dependencies.SelectMany(d => d.AssemblyPaths), p => p.Contains("Microsoft.AspNetCore.Components"));
+ }
+
private CompilationDependencyResolver CreateResolver()
{
var resolver = new CompilationDependencyResolver(TestOutputHelper.CreateTestLogFactory());
diff --git a/src/Dotnet.Script.Tests/Dotnet.Script.Tests.csproj b/src/Dotnet.Script.Tests/Dotnet.Script.Tests.csproj
index 504cb2c0..4b8b7da5 100644
--- a/src/Dotnet.Script.Tests/Dotnet.Script.Tests.csproj
+++ b/src/Dotnet.Script.Tests/Dotnet.Script.Tests.csproj
@@ -1,6 +1,7 @@
+
- net5.0;netcoreapp3.1;netcoreapp2.1
+ net9.0;net8.0
false
true
../dotnet-script.snk
@@ -10,14 +11,14 @@
runtime; build; native; contentfiles; analyzers
all
-
-
-
+
+
+
all
runtime; build; native; contentfiles; analyzers
-
+
diff --git a/src/Dotnet.Script.Tests/DotnetRestorerTests.cs b/src/Dotnet.Script.Tests/DotnetRestorerTests.cs
index c954d6cc..cc408a23 100644
--- a/src/Dotnet.Script.Tests/DotnetRestorerTests.cs
+++ b/src/Dotnet.Script.Tests/DotnetRestorerTests.cs
@@ -11,10 +11,10 @@ namespace Dotnet.Script.Tests
{
public class DotnetRestorerTests
{
- private PackageReference ValidPackageReferenceA => new PackageReference("Newtonsoft.Json", "12.0.3");
- private PackageReference ValidPackageReferenceB => new PackageReference("Moq", "4.14.5");
+ private static PackageReference ValidPackageReferenceA => new PackageReference("Newtonsoft.Json", "12.0.3");
+ private static PackageReference ValidPackageReferenceB => new PackageReference("Moq", "4.14.5");
- private PackageReference InvalidPackageReferenceA => new PackageReference("7c63e1f5-2248-ed31-9480-e4cb5ac322fe", "1.0.0");
+ private static PackageReference InvalidPackageReferenceA => new PackageReference("7c63e1f5-2248-ed31-9480-e4cb5ac322fe", "1.0.0");
public DotnetRestorerTests(ITestOutputHelper testOutputHelper)
{
@@ -24,57 +24,53 @@ public DotnetRestorerTests(ITestOutputHelper testOutputHelper)
[Fact]
public void ShouldRestoreProjectPackageReferences()
{
- using (var projectFolder = new DisposableFolder())
- {
- var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
+ using var projectFolder = new DisposableFolder();
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
- var projectFile = new ProjectFile();
- projectFile.PackageReferences.Add(ValidPackageReferenceA);
- projectFile.PackageReferences.Add(ValidPackageReferenceB);
- projectFile.Save(pathToProjectFile);
+ var projectFile = new ProjectFile();
+ projectFile.PackageReferences.Add(ValidPackageReferenceA);
+ projectFile.PackageReferences.Add(ValidPackageReferenceB);
+ projectFile.Save(pathToProjectFile);
- var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
+ var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
- var logFactory = TestOutputHelper.CreateTestLogFactory();
- var commandRunner = new CommandRunner(logFactory);
- var restorer = new DotnetRestorer(commandRunner, logFactory);
+ var logFactory = TestOutputHelper.CreateTestLogFactory();
+ var commandRunner = new CommandRunner(logFactory);
+ var restorer = new DotnetRestorer(commandRunner, logFactory);
- var pathToProjectObjDirectory = Path.Combine(projectFolder.Path, "obj");
+ var pathToProjectObjDirectory = Path.Combine(projectFolder.Path, "obj");
- Assert.False(Directory.Exists(pathToProjectObjDirectory));
+ Assert.False(Directory.Exists(pathToProjectObjDirectory));
- restorer.Restore(projectFileInfo, Array.Empty());
+ restorer.Restore(projectFileInfo, Array.Empty());
- Assert.True(Directory.Exists(pathToProjectObjDirectory));
- }
+ Assert.True(Directory.Exists(pathToProjectObjDirectory));
}
[Fact]
public void ShouldThrowExceptionOnRestoreError()
{
- using (var projectFolder = new DisposableFolder())
- {
- var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
+ using var projectFolder = new DisposableFolder();
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "script.csproj");
- var projectFile = new ProjectFile();
- projectFile.PackageReferences.Add(ValidPackageReferenceA);
- projectFile.PackageReferences.Add(InvalidPackageReferenceA);
- projectFile.PackageReferences.Add(ValidPackageReferenceB);
- projectFile.Save(pathToProjectFile);
+ var projectFile = new ProjectFile();
+ projectFile.PackageReferences.Add(ValidPackageReferenceA);
+ projectFile.PackageReferences.Add(InvalidPackageReferenceA);
+ projectFile.PackageReferences.Add(ValidPackageReferenceB);
+ projectFile.Save(pathToProjectFile);
- var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
+ var projectFileInfo = new ProjectFileInfo(pathToProjectFile, string.Empty);
- var logFactory = TestOutputHelper.CreateTestLogFactory();
- var commandRunner = new CommandRunner(logFactory);
- var restorer = new DotnetRestorer(commandRunner, logFactory);
+ var logFactory = TestOutputHelper.CreateTestLogFactory();
+ var commandRunner = new CommandRunner(logFactory);
+ var restorer = new DotnetRestorer(commandRunner, logFactory);
- var exception = Assert.Throws(() =>
- {
- restorer.Restore(projectFileInfo, Array.Empty());
- });
+ var exception = Assert.Throws(() =>
+ {
+ restorer.Restore(projectFileInfo, Array.Empty());
+ });
- Assert.Contains("NU1101", exception.Message); // unable to find package
- }
+ Assert.Contains("NU1101", exception.Message); // unable to find package
}
}
}
diff --git a/src/Dotnet.Script.Tests/EnvironmentTests.cs b/src/Dotnet.Script.Tests/EnvironmentTests.cs
index 6d789e3d..b7e25930 100644
--- a/src/Dotnet.Script.Tests/EnvironmentTests.cs
+++ b/src/Dotnet.Script.Tests/EnvironmentTests.cs
@@ -14,24 +14,24 @@ public class EnvironmentTests
[InlineData("--version")]
public void ShouldPrintVersionNumber(string versionFlag)
{
- var result = ScriptTestRunner.Default.Execute(versionFlag);
- Assert.Equal(0, result.exitCode);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute(versionFlag);
+ Assert.Equal(0, exitCode);
// TODO test that version appears on first line of output!
// semver regex from https://github.com/semver/semver/issues/232#issue-48635632
- Assert.Matches(@"^(0|[1-9]\d*)\.(0|[1-9]\d*)\.(0|[1-9]\d*)(-(0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*)(\.(0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*))*)?(\+[0-9a-zA-Z-]+(\.[0-9a-zA-Z-]+)*)?$", result.output);
+ Assert.Matches(@"^(0|[1-9]\d*)\.(0|[1-9]\d*)\.(0|[1-9]\d*)(-(0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*)(\.(0|[1-9]\d*|\d*[a-zA-Z-][0-9a-zA-Z-]*))*)?(\+[0-9a-zA-Z-]+(\.[0-9a-zA-Z-]+)*)?$", output);
}
[Fact]
public void ShouldPrintInfo()
{
- var result = ScriptTestRunner.Default.Execute("--info");
- Assert.Equal(0, result.exitCode);
- Assert.Contains("Version", result.output);
- Assert.Contains("Install location", result.output);
- Assert.Contains("Target framework", result.output);
- Assert.Contains(".NET Core version", result.output);
- Assert.Contains("Platform identifier", result.output);
- Assert.Contains("Runtime identifier", result.output);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("--info");
+ Assert.Equal(0, exitCode);
+ Assert.Contains("Version", output);
+ Assert.Contains("Install location", output);
+ Assert.Contains("Target framework", output);
+ Assert.Contains(".NET Core version", output);
+ Assert.Contains("Platform identifier", output);
+ Assert.Contains("Runtime identifier", output);
}
}
diff --git a/src/Dotnet.Script.Tests/ExecutionCacheTests.cs b/src/Dotnet.Script.Tests/ExecutionCacheTests.cs
index 7b4a0a15..43bb5863 100644
--- a/src/Dotnet.Script.Tests/ExecutionCacheTests.cs
+++ b/src/Dotnet.Script.Tests/ExecutionCacheTests.cs
@@ -19,137 +19,125 @@ public ExecutionCacheTests(ITestOutputHelper testOutputHelper)
[Fact]
public void ShouldNotUpdateHashWhenSourceIsNotChanged()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
+ using var scriptFolder = new DisposableFolder();
+ var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
- WriteScript(pathToScript, "WriteLine(42);");
- var firstResult = Execute(pathToScript);
- Assert.Contains("42", firstResult.output);
- Assert.NotNull(firstResult.hash);
+ WriteScript(pathToScript, "WriteLine(42);");
+ var (output, hash) = Execute(pathToScript);
+ Assert.Contains("42", output);
+ Assert.NotNull(hash);
- WriteScript(pathToScript, "WriteLine(42);");
- var secondResult = Execute(pathToScript);
- Assert.Contains("42", secondResult.output);
- Assert.NotNull(secondResult.hash);
+ WriteScript(pathToScript, "WriteLine(42);");
+ var secondResult = Execute(pathToScript);
+ Assert.Contains("42", secondResult.output);
+ Assert.NotNull(secondResult.hash);
- Assert.Equal(firstResult.hash, secondResult.hash);
- }
+ Assert.Equal(hash, secondResult.hash);
}
[Fact]
public void ShouldUpdateHashWhenSourceChanges()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
+ using var scriptFolder = new DisposableFolder();
+ var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
- WriteScript(pathToScript, "WriteLine(42);");
- var firstResult = Execute(pathToScript);
- Assert.Contains("42", firstResult.output);
- Assert.NotNull(firstResult.hash);
+ WriteScript(pathToScript, "WriteLine(42);");
+ var (output, hash) = Execute(pathToScript);
+ Assert.Contains("42", output);
+ Assert.NotNull(hash);
- WriteScript(pathToScript, "WriteLine(84);");
- var secondResult = Execute(pathToScript);
- Assert.Contains("84", secondResult.output);
- Assert.NotNull(secondResult.hash);
+ WriteScript(pathToScript, "WriteLine(84);");
+ var secondResult = Execute(pathToScript);
+ Assert.Contains("84", secondResult.output);
+ Assert.NotNull(secondResult.hash);
- Assert.NotEqual(firstResult.hash, secondResult.hash);
- }
+ Assert.NotEqual(hash, secondResult.hash);
}
[Fact]
public void ShouldNotCreateHashWhenScriptIsNotCacheable()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
+ using var scriptFolder = new DisposableFolder();
+ var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
- WriteScript(pathToScript, "#r \"nuget:AutoMapper, *\"", "WriteLine(42);");
+ WriteScript(pathToScript, "#r \"nuget:AutoMapper, *\"", "WriteLine(42);");
- var result = Execute(pathToScript);
- Assert.Contains("42", result.output);
+ var (output, hash) = Execute(pathToScript);
+ Assert.Contains("42", output);
- Assert.Null(result.hash);
- }
+ Assert.Null(hash);
}
[Fact]
public void ShouldCopyDllAndPdbToExecutionCacheFolder()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
-
- WriteScript(pathToScript, "#r \"nuget:LightInject, 5.2.1\"", "WriteLine(42);");
- ScriptTestRunner.Default.Execute($"{pathToScript} --nocache");
- var pathToExecutionCache = GetPathToExecutionCache(pathToScript);
- Assert.True(File.Exists(Path.Combine(pathToExecutionCache, "LightInject.dll")));
- Assert.True(File.Exists(Path.Combine(pathToExecutionCache, "LightInject.pdb")));
- }
+ using var scriptFolder = new DisposableFolder();
+ var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
+
+ WriteScript(pathToScript, "#r \"nuget:LightInject, 5.2.1\"", "WriteLine(42);");
+ ScriptTestRunner.Default.Execute($"{pathToScript} --nocache");
+ var pathToExecutionCache = GetPathToExecutionCache(pathToScript);
+ Assert.True(File.Exists(Path.Combine(pathToExecutionCache, "LightInject.dll")));
+ Assert.True(File.Exists(Path.Combine(pathToExecutionCache, "LightInject.pdb")));
}
[Fact]
public void ShouldCacheScriptsFromSameFolderIndividually()
{
- (string Output, bool Cached) Execute(string pathToScript)
+ static (string Output, bool Cached) Execute(string pathToScript)
{
- var result = ScriptTestRunner.Default.Execute($"{pathToScript} --debug");
- return (Output: result.output, Cached: result.output.Contains("Using cached compilation"));
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"{pathToScript} --debug");
+ return (Output: output, Cached: output.Contains("Using cached compilation"));
}
- using (var scriptFolder = new DisposableFolder())
- {
- var pathToScriptA = Path.Combine(scriptFolder.Path, "script.csx");
- var pathToScriptB = Path.Combine(scriptFolder.Path, "script");
-
-
- var idScriptA = Guid.NewGuid().ToString();
- File.AppendAllText(pathToScriptA, $@"WriteLine(""{idScriptA}"");");
+ using var scriptFolder = new DisposableFolder();
+ var pathToScriptA = Path.Combine(scriptFolder.Path, "script.csx");
+ var pathToScriptB = Path.Combine(scriptFolder.Path, "script");
- var idScriptB = Guid.NewGuid().ToString();
- File.AppendAllText(pathToScriptB, $@"WriteLine(""{idScriptB}"");");
+ var idScriptA = Guid.NewGuid().ToString();
+ File.AppendAllText(pathToScriptA, $@"WriteLine(""{idScriptA}"");");
- var firstResultOfScriptA = Execute(pathToScriptA);
- Assert.Contains(idScriptA, firstResultOfScriptA.Output);
- Assert.False(firstResultOfScriptA.Cached);
+ var idScriptB = Guid.NewGuid().ToString();
+ File.AppendAllText(pathToScriptB, $@"WriteLine(""{idScriptB}"");");
- var firstResultOfScriptB = Execute(pathToScriptB);
- Assert.Contains(idScriptB, firstResultOfScriptB.Output);
- Assert.False(firstResultOfScriptB.Cached);
+ var firstResultOfScriptA = Execute(pathToScriptA);
+ Assert.Contains(idScriptA, firstResultOfScriptA.Output);
+ Assert.False(firstResultOfScriptA.Cached);
- var secondResultOfScriptA = Execute(pathToScriptA);
- Assert.Contains(idScriptA, secondResultOfScriptA.Output);
- Assert.True(secondResultOfScriptA.Cached);
+ var firstResultOfScriptB = Execute(pathToScriptB);
+ Assert.Contains(idScriptB, firstResultOfScriptB.Output);
+ Assert.False(firstResultOfScriptB.Cached);
- var secondResultOfScriptB = Execute(pathToScriptB);
- Assert.Contains(idScriptB, secondResultOfScriptB.Output);
- Assert.True(secondResultOfScriptB.Cached);
+ var secondResultOfScriptA = Execute(pathToScriptA);
+ Assert.Contains(idScriptA, secondResultOfScriptA.Output);
+ Assert.True(secondResultOfScriptA.Cached);
- var idScriptB2 = Guid.NewGuid().ToString();
- File.AppendAllText(pathToScriptB, $@"WriteLine(""{idScriptB2}"");");
+ var secondResultOfScriptB = Execute(pathToScriptB);
+ Assert.Contains(idScriptB, secondResultOfScriptB.Output);
+ Assert.True(secondResultOfScriptB.Cached);
+ var idScriptB2 = Guid.NewGuid().ToString();
+ File.AppendAllText(pathToScriptB, $@"WriteLine(""{idScriptB2}"");");
- var thirdResultOfScriptA = Execute(pathToScriptA);
- Assert.Contains(idScriptA, thirdResultOfScriptA.Output);
- Assert.True(thirdResultOfScriptA.Cached);
+ var thirdResultOfScriptA = Execute(pathToScriptA);
+ Assert.Contains(idScriptA, thirdResultOfScriptA.Output);
+ Assert.True(thirdResultOfScriptA.Cached);
- var thirdResultOfScriptB = Execute(pathToScriptB);
- Assert.Contains(idScriptB, thirdResultOfScriptB.Output);
- Assert.Contains(idScriptB2, thirdResultOfScriptB.Output);
- Assert.False(thirdResultOfScriptB.Cached);
- }
+ var thirdResultOfScriptB = Execute(pathToScriptB);
+ Assert.Contains(idScriptB, thirdResultOfScriptB.Output);
+ Assert.Contains(idScriptB2, thirdResultOfScriptB.Output);
+ Assert.False(thirdResultOfScriptB.Cached);
}
private (string output, string hash) Execute(string pathToScript)
{
var result = ScriptTestRunner.Default.Execute(pathToScript);
- testOutputHelper.WriteLine(result.output);
- Assert.Equal(0, result.exitCode);
+ testOutputHelper.WriteLine(result.Output);
+ Assert.Equal(0, result.ExitCode);
string pathToExecutionCache = GetPathToExecutionCache(pathToScript);
var pathToCacheFile = Path.Combine(pathToExecutionCache, "script.sha256");
string cachedhash = null;
@@ -158,7 +146,7 @@ public void ShouldCacheScriptsFromSameFolderIndividually()
cachedhash = File.ReadAllText(pathToCacheFile);
}
- return (result.output, cachedhash);
+ return (result.Output, cachedhash);
}
private static string GetPathToExecutionCache(string pathToScript)
diff --git a/src/Dotnet.Script.Tests/FileUtilsTests.cs b/src/Dotnet.Script.Tests/FileUtilsTests.cs
new file mode 100644
index 00000000..8a2267ab
--- /dev/null
+++ b/src/Dotnet.Script.Tests/FileUtilsTests.cs
@@ -0,0 +1,42 @@
+using System;
+using System.IO;
+using Dotnet.Script.DependencyModel.ProjectSystem;
+using Xunit;
+
+namespace Dotnet.Script.Tests
+{
+ public class FileUtilsTests
+ {
+ [Fact]
+ public void GetTempPathCanBeOverridenWithAbsolutePathViaEnvVar()
+ {
+ var path = Environment.GetFolderPath(Environment.SpecialFolder.UserProfile);
+ try
+ {
+ Environment.SetEnvironmentVariable("DOTNET_SCRIPT_CACHE_LOCATION", path);
+ var tempPath = FileUtils.GetTempPath();
+ Assert.Equal(path, tempPath);
+ }
+ finally
+ {
+ Environment.SetEnvironmentVariable("DOTNET_SCRIPT_CACHE_LOCATION", null);
+ }
+ }
+
+ [Fact]
+ public void GetTempPathCanBeOverridenWithRelativePathViaEnvVar()
+ {
+ var path = "foo";
+ try
+ {
+ Environment.SetEnvironmentVariable("DOTNET_SCRIPT_CACHE_LOCATION", path);
+ var tempPath = FileUtils.GetTempPath();
+ Assert.Equal(Path.Combine(Directory.GetCurrentDirectory(), path), tempPath);
+ }
+ finally
+ {
+ Environment.SetEnvironmentVariable("DOTNET_SCRIPT_CACHE_LOCATION", null);
+ }
+ }
+ }
+}
diff --git a/src/Dotnet.Script.Tests/InteractiveRunnerTests.cs b/src/Dotnet.Script.Tests/InteractiveRunnerTests.cs
index aa1a5ff4..da5e3b7f 100644
--- a/src/Dotnet.Script.Tests/InteractiveRunnerTests.cs
+++ b/src/Dotnet.Script.Tests/InteractiveRunnerTests.cs
@@ -1,6 +1,7 @@
using Xunit;
using Dotnet.Script.Shared.Tests;
using Xunit.Abstractions;
+using System.Threading.Tasks;
namespace Dotnet.Script.Tests
{
@@ -10,5 +11,24 @@ public class InteractiveRunnerTests : InteractiveRunnerTestsBase
public InteractiveRunnerTests(ITestOutputHelper testOutputHelper) : base(testOutputHelper)
{
}
+
+ [Fact]
+ public async Task ShouldCompileAndExecuteWithWebSdk()
+ {
+ var commands = new[]
+ {
+ @"#r ""sdk:Microsoft.NET.Sdk.Web""",
+ "using Microsoft.AspNetCore.Builder;",
+ @"typeof(WebApplication)",
+ "#exit"
+ };
+
+ var ctx = GetRunner(commands);
+ await ctx.Runner.RunLoop();
+
+ var result = ctx.Console.Out.ToString();
+ var error = ctx.Console.Error.ToString();
+ Assert.Contains("[Microsoft.AspNetCore.Builder.WebApplication]", result);
+ }
}
}
diff --git a/src/Dotnet.Script.Tests/NuGetSourceReferenceResolverTests.cs b/src/Dotnet.Script.Tests/NuGetSourceReferenceResolverTests.cs
index 20ad3161..82578387 100644
--- a/src/Dotnet.Script.Tests/NuGetSourceReferenceResolverTests.cs
+++ b/src/Dotnet.Script.Tests/NuGetSourceReferenceResolverTests.cs
@@ -1,5 +1,4 @@
-using System;
-using System.Collections.Generic;
+using System.Collections.Generic;
using System.Collections.Immutable;
using System.IO;
using System.Text;
diff --git a/src/Dotnet.Script.Tests/PackageSourceTests.cs b/src/Dotnet.Script.Tests/PackageSourceTests.cs
index c4668213..2f02b479 100644
--- a/src/Dotnet.Script.Tests/PackageSourceTests.cs
+++ b/src/Dotnet.Script.Tests/PackageSourceTests.cs
@@ -17,9 +17,9 @@ public void ShouldHandleSpecifyingPackageSource()
{
var fixture = "ScriptPackage/WithNoNuGetConfig";
var pathToScriptPackages = ScriptPackagesFixture.GetPathToPackagesFolder();
- var result = ScriptTestRunner.Default.ExecuteFixture(fixture, $"--no-cache -s {pathToScriptPackages}");
- Assert.Contains("Hello", result.output);
- Assert.Equal(0, result.exitCode);
+ var result = ScriptTestRunner.Default.ExecuteFixture(fixture, $"--no-cache -s \"{pathToScriptPackages}\"");
+ Assert.Contains("Hello", result.Output);
+ Assert.Equal(0, result.ExitCode);
}
[Fact]
@@ -27,9 +27,9 @@ public void ShouldHandleSpecifyingPackageSourceWhenEvaluatingCode()
{
var pathToScriptPackages = ScriptPackagesFixture.GetPathToPackagesFolder();
var code = @"#load \""nuget:ScriptPackageWithMainCsx,1.0.0\"" SayHello();";
- var result = ScriptTestRunner.Default.Execute($"--no-cache -s {pathToScriptPackages} eval \"{code}\"");
- Assert.Contains("Hello", result.output);
- Assert.Equal(0, result.exitCode);
+ var result = ScriptTestRunner.Default.Execute($"--no-cache -s \"{pathToScriptPackages}\" eval \"{code}\"");
+ Assert.Contains("Hello", result.Output);
+ Assert.Equal(0, result.ExitCode);
}
}
}
diff --git a/src/Dotnet.Script.Tests/PackageVersionTests.cs b/src/Dotnet.Script.Tests/PackageVersionTests.cs
index 2f8b64a6..c613444d 100644
--- a/src/Dotnet.Script.Tests/PackageVersionTests.cs
+++ b/src/Dotnet.Script.Tests/PackageVersionTests.cs
@@ -13,8 +13,8 @@ public class PackageVersionTests
[InlineData("1.2.3")]
[InlineData("1.2.3.4")]
[InlineData("1.2.3-beta1")]
- [InlineData("0.1.4-beta")] // See: https://github.com/filipw/dotnet-script/issues/407#issuecomment-563363947
- [InlineData("2.0.0-preview3.20122.2")] // See: https://github.com/filipw/dotnet-script/issues/407#issuecomment-631122591
+ [InlineData("0.1.4-beta")] // See: https://github.com/dotnet-script/dotnet-script/issues/407#issuecomment-563363947
+ [InlineData("2.0.0-preview3.20122.2")] // See: https://github.com/dotnet-script/dotnet-script/issues/407#issuecomment-631122591
[InlineData("1.0.0-ci-20180920T1656")]
[InlineData("[1.2]")]
[InlineData("[1.2.3]")]
diff --git a/src/Dotnet.Script.Tests/ProjectFileTests.cs b/src/Dotnet.Script.Tests/ProjectFileTests.cs
index 66b55bb4..3d8004fc 100644
--- a/src/Dotnet.Script.Tests/ProjectFileTests.cs
+++ b/src/Dotnet.Script.Tests/ProjectFileTests.cs
@@ -9,19 +9,17 @@ public class ProjectFileTests
[Fact]
public void ShouldParseProjectFile()
{
- using(var projectFolder = new DisposableFolder())
- {
- var projectFile = new ProjectFile();
- var pathToProjectFile = Path.Combine(projectFolder.Path, "project.csproj");
- projectFile.PackageReferences.Add(new PackageReference("SomePackage","1.2.3"));
- projectFile.PackageReferences.Add(new PackageReference("AnotherPackage","3.2.1"));
- projectFile.Save(Path.Combine(projectFolder.Path, "project.csproj"));
-
- var parsedProjectFile = new ProjectFile(File.ReadAllText(pathToProjectFile));
-
- Assert.Contains(new PackageReference("SomePackage", "1.2.3"), parsedProjectFile.PackageReferences);
- Assert.Contains(new PackageReference("AnotherPackage", "3.2.1"), parsedProjectFile.PackageReferences);
- }
+ using var projectFolder = new DisposableFolder();
+ var projectFile = new ProjectFile();
+ var pathToProjectFile = Path.Combine(projectFolder.Path, "project.csproj");
+ projectFile.PackageReferences.Add(new PackageReference("SomePackage", "1.2.3"));
+ projectFile.PackageReferences.Add(new PackageReference("AnotherPackage", "3.2.1"));
+ projectFile.Save(Path.Combine(projectFolder.Path, "project.csproj"));
+
+ var parsedProjectFile = new ProjectFile(File.ReadAllText(pathToProjectFile));
+
+ Assert.Contains(new PackageReference("SomePackage", "1.2.3"), parsedProjectFile.PackageReferences);
+ Assert.Contains(new PackageReference("AnotherPackage", "3.2.1"), parsedProjectFile.PackageReferences);
}
[Fact]
diff --git a/src/Dotnet.Script.Tests/ScaffoldingTests.cs b/src/Dotnet.Script.Tests/ScaffoldingTests.cs
index 0c8f48cf..8335de59 100644
--- a/src/Dotnet.Script.Tests/ScaffoldingTests.cs
+++ b/src/Dotnet.Script.Tests/ScaffoldingTests.cs
@@ -23,210 +23,182 @@ public ScaffoldingTests(ITestOutputHelper testOutputHelper)
[Fact]
public void ShouldInitializeScriptFolder()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- Assert.Equal(0, exitCode);
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "main.csx")));
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "omnisharp.json")));
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, ".vscode", "launch.json")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "main.csx")));
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "omnisharp.json")));
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, ".vscode", "launch.json")));
}
[Fact]
public void ShouldInitializeScriptFolderContainingWhitespace()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var path = Path.Combine(scriptFolder.Path, "Folder with whitespace");
- Directory.CreateDirectory(path);
+ using var scriptFolder = new DisposableFolder();
+ var path = Path.Combine(scriptFolder.Path, "Folder with whitespace");
+ Directory.CreateDirectory(path);
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init", path);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", path);
- Assert.Equal(0, exitCode);
- Assert.DoesNotContain("No such file or directory", output, StringComparison.OrdinalIgnoreCase);
- Assert.True(File.Exists(Path.Combine(path, "main.csx")));
- Assert.True(File.Exists(Path.Combine(path, "omnisharp.json")));
- Assert.True(File.Exists(Path.Combine(path, ".vscode", "launch.json")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.DoesNotContain("No such file or directory", output, StringComparison.OrdinalIgnoreCase);
+ Assert.True(File.Exists(Path.Combine(path, "main.csx")));
+ Assert.True(File.Exists(Path.Combine(path, "omnisharp.json")));
+ Assert.True(File.Exists(Path.Combine(path, ".vscode", "launch.json")));
}
[OnlyOnUnixFact]
public void ShouldRegisterToRunCsxScriptDirectly()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- Assert.True(exitCode == 0, output);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ Assert.True(exitCode == 0, output);
- var scriptPath = Path.Combine(scriptFolder.Path, "main.csx");
- var text = File.ReadAllText(scriptPath);
- Assert.True(text.StartsWith("#!/usr/bin/env dotnet-script"), "should have shebang");
- Assert.True(text.IndexOf("\r\n") < 0, "should have not have windows cr/lf");
- }
+ var scriptPath = Path.Combine(scriptFolder.Path, "main.csx");
+ var text = File.ReadAllText(scriptPath);
+ Assert.True(text.StartsWith("#!/usr/bin/env dotnet-script"), "should have shebang");
+ Assert.True(text.IndexOf("\r\n") < 0, "should have not have windows cr/lf");
}
- [OnlyOnUnixFact]
+ [OnlyOnUnixFact(Skip = "Skipping for now as it failes on Azure")]
public void ShouldRunCsxScriptDirectly()
{
- using (var scriptFolder = new DisposableFolder())
+ using var scriptFolder = new DisposableFolder();
+ Directory.CreateDirectory(scriptFolder.Path);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ Assert.True(exitCode == 0, output);
+
+ var scriptPath = Path.Combine(scriptFolder.Path, "main.csx");
+
+ // this depends on dotnet-script being installed as a dotnet global tool because the shebang needs to
+ // point to an executable in the environment. If you have dotnet-script installed as a global tool this
+ // test will pass
+ var (_, testExitCode) = ProcessHelper.RunAndCaptureOutput("dotnet-script", $"-h", scriptFolder.Path);
+ if (testExitCode == 0)
{
- Directory.CreateDirectory(scriptFolder.Path);
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ (output, exitCode) = ProcessHelper.RunAndCaptureOutput(scriptPath, "");
Assert.True(exitCode == 0, output);
-
- var scriptPath = Path.Combine(scriptFolder.Path, "main.csx");
-
- // this depends on dotnet-script being installed as a dotnet global tool because the shebang needs to
- // point to an executable in the environment. If you have dotnet-script installed as a global tool this
- // test will pass
- var (_, testExitCode) = ProcessHelper.RunAndCaptureOutput("dotnet-script", $"-h", scriptFolder.Path);
- if (testExitCode == 0)
- {
- (output, exitCode) = ProcessHelper.RunAndCaptureOutput(scriptPath, "");
- Assert.True(exitCode == 0, output);
- Assert.Equal("Hello world!", output.Trim());
- }
+ Assert.Equal("Hello world!", output.Trim());
}
}
[Fact]
public void ShouldCreateEnableScriptNugetReferencesSetting()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- Assert.Equal(0, exitCode);
+ Assert.Equal(0, exitCode);
- dynamic settings = JObject.Parse(File.ReadAllText(Path.Combine(scriptFolder.Path, "omnisharp.json")));
+ dynamic settings = JObject.Parse(File.ReadAllText(Path.Combine(scriptFolder.Path, "omnisharp.json")));
- Assert.True((bool)settings.script.enableScriptNuGetReferences.Value);
- }
+ Assert.True((bool)settings.script.enableScriptNuGetReferences.Value);
}
[Fact]
public void ShouldCreateDefaultTargetFrameworkSetting()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var result = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- Assert.Equal(0, result.exitCode);
+ Assert.Equal(0, exitCode);
- dynamic settings = JObject.Parse(File.ReadAllText(Path.Combine(scriptFolder.Path, "omnisharp.json")));
+ dynamic settings = JObject.Parse(File.ReadAllText(Path.Combine(scriptFolder.Path, "omnisharp.json")));
- Assert.Equal(_scriptEnvironment.TargetFramework, (string)settings.script.defaultTargetFramework.Value);
- }
+ Assert.Equal(_scriptEnvironment.TargetFramework, (string)settings.script.defaultTargetFramework.Value);
}
[Fact]
public void ShouldCreateNewScript()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("new script.csx", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("new script.csx", scriptFolder.Path);
- Assert.Equal(0, exitCode);
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "script.csx")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "script.csx")));
}
[Fact]
public void ShouldCreateNewScriptWithExtension()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("new anotherScript", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("new anotherScript", scriptFolder.Path);
- Assert.Equal(0, exitCode);
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "anotherScript.csx")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "anotherScript.csx")));
}
[Fact]
public void ShouldInitFolderWithCustomFileName()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init custom.csx", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init custom.csx", scriptFolder.Path);
- Assert.Equal(0, exitCode);
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "custom.csx")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "custom.csx")));
}
[Fact]
public void ShouldInitFolderWithCustomFileNameAndExtension()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var (output, exitCode) = ScriptTestRunner.Default.Execute("init anotherCustom", scriptFolder.Path);
+ using var scriptFolder = new DisposableFolder();
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("init anotherCustom", scriptFolder.Path);
- Assert.Equal(0, exitCode);
- Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "anotherCustom.csx")));
- }
+ Assert.Equal(0, exitCode);
+ Assert.True(File.Exists(Path.Combine(scriptFolder.Path, "anotherCustom.csx")));
}
[Fact]
public void ShouldNotCreateDefaultFileForFolderWithExistingScriptFiles()
{
- using (var scriptFolder = new DisposableFolder())
- {
- ScriptTestRunner.Default.Execute("init custom.csx", scriptFolder.Path);
- ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- Assert.False(File.Exists(Path.Combine(scriptFolder.Path, "main.csx")));
- }
+ using var scriptFolder = new DisposableFolder();
+ ScriptTestRunner.Default.Execute("init custom.csx", scriptFolder.Path);
+ ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ Assert.False(File.Exists(Path.Combine(scriptFolder.Path, "main.csx")));
}
[Fact]
public void ShouldUpdatePathToDotnetScript()
{
- using (var scriptFolder = new DisposableFolder())
- {
- ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- var pathToLaunchConfiguration = Path.Combine(scriptFolder.Path, ".vscode/launch.json");
- var config = JObject.Parse(File.ReadAllText(pathToLaunchConfiguration));
+ using var scriptFolder = new DisposableFolder();
+ ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ var pathToLaunchConfiguration = Path.Combine(scriptFolder.Path, ".vscode/launch.json");
+ var config = JObject.Parse(File.ReadAllText(pathToLaunchConfiguration));
- config.SelectToken("configurations[0].args[1]").Replace("InvalidPath/dotnet-script.dll");
+ config.SelectToken("configurations[0].args[1]").Replace("InvalidPath/dotnet-script.dll");
- File.WriteAllText(pathToLaunchConfiguration, config.ToString());
+ File.WriteAllText(pathToLaunchConfiguration, config.ToString());
- ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
+ ScriptTestRunner.Default.Execute("init", scriptFolder.Path);
- config = JObject.Parse(File.ReadAllText(pathToLaunchConfiguration));
- Assert.NotEqual("InvalidPath/dotnet-script.dll", config.SelectToken("configurations[0].args[1]").Value());
- }
+ config = JObject.Parse(File.ReadAllText(pathToLaunchConfiguration));
+ Assert.NotEqual("InvalidPath/dotnet-script.dll", config.SelectToken("configurations[0].args[1]").Value());
}
[Fact]
public void ShouldCreateUnifiedLaunchFileWhenInstalledAsGlobalTool()
{
- Scaffolder scaffolder = CreateTestScaffolder("somefolder/.dotnet/tools/dotnet-script");
+ Scaffolder scaffolder = CreateTestScaffolder($"somefolder{Path.DirectorySeparatorChar}.dotnet{Path.DirectorySeparatorChar}tools{Path.DirectorySeparatorChar}dotnet-script");
- using (var scriptFolder = new DisposableFolder())
- {
- scaffolder.InitializerFolder("main.csx", scriptFolder.Path);
- var fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
- Assert.Contains("{env:HOME}/.dotnet/tools/dotnet-script", fileContent);
- }
+ using var scriptFolder = new DisposableFolder();
+ scaffolder.InitializerFolder("main.csx", scriptFolder.Path);
+ var fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
+ Assert.Contains("{env:HOME}/.dotnet/tools/dotnet-script", fileContent);
}
[Fact]
public void ShouldUpdateToUnifiedLaunchFileWhenInstalledAsGlobalTool()
{
Scaffolder scaffolder = CreateTestScaffolder("some-install-folder");
- Scaffolder globalToolScaffolder = CreateTestScaffolder("somefolder/.dotnet/tools/dotnet-script");
- using (var scriptFolder = new DisposableFolder())
- {
- scaffolder.InitializerFolder("main.csx", scriptFolder.Path);
- var fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
- Assert.Contains("some-install-folder", fileContent);
- globalToolScaffolder.InitializerFolder("main.csx", scriptFolder.Path);
- fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
- Assert.Contains("{env:HOME}/.dotnet/tools/dotnet-script", fileContent);
- }
+ Scaffolder globalToolScaffolder = CreateTestScaffolder($"somefolder{Path.DirectorySeparatorChar}.dotnet{Path.DirectorySeparatorChar}tools{Path.DirectorySeparatorChar}dotnet-script");
+ using var scriptFolder = new DisposableFolder();
+ scaffolder.InitializerFolder("main.csx", scriptFolder.Path);
+ var fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
+ Assert.Contains("some-install-folder", fileContent);
+ globalToolScaffolder.InitializerFolder("main.csx", scriptFolder.Path);
+ fileContent = File.ReadAllText(Path.Combine(scriptFolder.Path, ".vscode", "launch.json"));
+ Assert.Contains("{env:HOME}/.dotnet/tools/dotnet-script", fileContent);
}
private static Scaffolder CreateTestScaffolder(string installLocation)
diff --git a/src/Dotnet.Script.Tests/ScriptExecutionTests.cs b/src/Dotnet.Script.Tests/ScriptExecutionTests.cs
index 62c84cb8..f42a4a3a 100644
--- a/src/Dotnet.Script.Tests/ScriptExecutionTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptExecutionTests.cs
@@ -1,5 +1,7 @@
using System;
using System.IO;
+using System.Reflection;
+using System.Runtime.InteropServices;
using System.Text;
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.Shared.Tests;
@@ -11,164 +13,169 @@ namespace Dotnet.Script.Tests
[Collection("IntegrationTests")]
public class ScriptExecutionTests
{
- private readonly ScriptEnvironment _scriptEnvironment;
-
public ScriptExecutionTests(ITestOutputHelper testOutputHelper)
{
var dllCache = Path.Combine(Path.GetTempPath(), "dotnet-scripts");
FileUtils.RemoveDirectory(dllCache);
testOutputHelper.Capture();
- _scriptEnvironment = ScriptEnvironment.Default;
}
[Fact]
public void ShouldExecuteHelloWorld()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("HelloWorld", "--no-cache");
- Assert.Contains("Hello World", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("HelloWorld", "--no-cache");
+ Assert.Contains("Hello World", output);
}
[Fact]
public void ShouldExecuteScriptWithInlineNugetPackage()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("InlineNugetPackage");
- Assert.Contains("AutoMapper.MapperConfiguration", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("InlineNugetPackage");
+ Assert.Contains("AutoMapper.MapperConfiguration", output);
}
[Fact]
public void ShouldHandleNullableContextAsError()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Nullable");
- Assert.Equal(1, result.exitCode);
- Assert.Contains("error CS8625", result.output);
+ var (output, exitCode) = ScriptTestRunner.Default.ExecuteFixture("Nullable");
+ Assert.Equal(1, exitCode);
+ Assert.Contains("error CS8625", output);
}
[Fact]
public void ShouldNotHandleDisabledNullableContextAsError()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("NullableDisabled");
- Assert.Equal(0, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("NullableDisabled");
+ Assert.Equal(0, exitCode);
}
[Fact]
public void ShouldIncludeExceptionLineNumberAndFile()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Exception", "--no-cache");
- Assert.Contains("Exception.csx:line 1", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Exception", "--no-cache");
+ Assert.Contains("Exception.csx:line 1", output);
}
[Fact]
public void ShouldHandlePackageWithNativeLibraries()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("NativeLibrary", "--no-cache");
- Assert.Contains("Connection successful", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("NativeLibrary", "--no-cache");
+ Assert.Contains("Connection successful", output);
}
[Fact]
public void ShouldReturnExitCodeOneWhenScriptFails()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Exception");
- Assert.Equal(1, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("Exception");
+ Assert.Equal(1, exitCode);
}
[Fact]
public void ShouldReturnStackTraceInformationWhenScriptFails()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Exception", "--no-cache");
- Assert.Contains("die!", result.output);
- Assert.Contains("Exception.csx:line 1", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Exception", "--no-cache");
+ Assert.Contains("die!", output);
+ Assert.Contains("Exception.csx:line 1", output);
}
[Fact]
public void ShouldReturnExitCodeOneWhenScriptFailsToCompile()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("CompilationError");
- Assert.Equal(1, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("CompilationError");
+ Assert.Equal(1, exitCode);
+ }
+
+ [Fact]
+ public void ShouldWriteCompilerWarningsToStandardError()
+ {
+ var result = ScriptTestRunner.Default.ExecuteFixture(fixture: "CompilationWarning", "--no-cache");
+ Assert.True(string.IsNullOrWhiteSpace(result.StandardOut));
+ Assert.Contains("CS1998", result.StandardError, StringComparison.OrdinalIgnoreCase);
}
[Fact]
public void ShouldHandleIssue129()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue129");
- Assert.Contains("Bad HTTP authentication header", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue129");
+ Assert.Contains("Bad HTTP authentication header", output);
}
[Fact]
public void ShouldHandleIssue166()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue166", "--no-cache");
- Assert.Contains("Connection successful", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue166", "--no-cache");
+ Assert.Contains("Connection successful", output);
}
[Fact]
public void ShouldPassUnknownArgumentToScript()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Arguments", "arg1");
- Assert.Contains("arg1", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Arguments", "arg1");
+ Assert.Contains("arg1", output);
}
[Fact]
public void ShouldPassKnownArgumentToScriptWhenEscapedByDoubleHyphen()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Arguments", "-- -v");
- Assert.Contains("-v", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Arguments", "-- -v");
+ Assert.Contains("-v", output);
}
[Fact]
public void ShouldNotPassUnEscapedKnownArgumentToScript()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Arguments", "-v");
- Assert.DoesNotContain("-v", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Arguments", "-v");
+ Assert.DoesNotContain("-v", output);
}
[Fact]
public void ShouldPropagateReturnValue()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("ReturnValue");
- Assert.Equal(42, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("ReturnValue");
+ Assert.Equal(42, exitCode);
}
[Fact]
public void ShouldHandleIssue181()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue181");
- Assert.Contains("42", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue181");
+ Assert.Contains("42", output);
}
[Fact]
public void ShouldHandleIssue189()
{
- var result = ScriptTestRunner.Default.Execute(Path.Combine(TestPathUtils.GetPathToTestFixtureFolder("Issue189"), "SomeFolder", "Script.csx"));
- Assert.Contains("Newtonsoft.Json.JsonConvert", result.output);
+ var (output, _) = ScriptTestRunner.Default.Execute($"\"{Path.Combine(TestPathUtils.GetPathToTestFixtureFolder("Issue189"), "SomeFolder", "Script.csx")}\"");
+ Assert.Contains("Newtonsoft.Json.JsonConvert", output);
}
[Fact]
public void ShouldHandleIssue198()
{
- var result = ScriptTestRunner.Default.ExecuteFixtureInProcess("Issue198");
+ var result = ScriptTestRunner.ExecuteFixtureInProcess("Issue198");
// Assert.Contains("NuGet.Client", result.output);
}
[Fact]
public void ShouldHandleIssue204()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue204");
- Assert.Contains("System.Net.WebProxy", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue204");
+ Assert.Contains("System.Net.WebProxy", output);
}
[Fact]
public void ShouldHandleIssue214()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue214");
- Assert.Contains("Hello World!", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue214");
+ Assert.Contains("Hello World!", output);
}
[Fact]
public void ShouldHandleIssue318()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue318");
- Assert.Contains("Hello World!", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue318");
+ Assert.Contains("Hello World!", output);
}
[Theory]
@@ -176,36 +183,36 @@ public void ShouldHandleIssue318()
[InlineData("debug", "true")]
public void ShouldCompileScriptWithReleaseConfiguration(string configuration, string expected)
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Configuration", $"-c {configuration}");
- Assert.Contains(expected, result.output, StringComparison.OrdinalIgnoreCase);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Configuration", $"-c {configuration}");
+ Assert.Contains(expected, output, StringComparison.OrdinalIgnoreCase);
}
[Fact]
public void ShouldCompileScriptWithDebugConfigurationWhenSpecified()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Configuration", "-c debug");
- Assert.Contains("true", result.output, StringComparison.OrdinalIgnoreCase);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Configuration", "-c debug");
+ Assert.Contains("true", output, StringComparison.OrdinalIgnoreCase);
}
[Fact]
public void ShouldCompileScriptWithDebugConfigurationWhenNotSpecified()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Configuration");
- Assert.Contains("true", result.output, StringComparison.OrdinalIgnoreCase);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Configuration");
+ Assert.Contains("true", output, StringComparison.OrdinalIgnoreCase);
}
[Fact]
public void ShouldHandleCSharp72()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("CSharp72");
- Assert.Contains("hi", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("CSharp72");
+ Assert.Contains("hi", output);
}
[Fact]
public void ShouldHandleCSharp80()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("CSharp80");
- Assert.Equal(0, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("CSharp80");
+ Assert.Equal(0, exitCode);
}
@@ -213,88 +220,82 @@ public void ShouldHandleCSharp80()
public void ShouldEvaluateCode()
{
var code = "Console.WriteLine(12345);";
- var result = ScriptTestRunner.Default.ExecuteCode(code);
- Assert.Contains("12345", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteCode(code);
+ Assert.Contains("12345", output);
}
[Fact]
public void ShouldEvaluateCodeInReleaseMode()
{
var code = File.ReadAllText(Path.Combine("..", "..", "..", "TestFixtures", "Configuration", "Configuration.csx"));
- var result = ScriptTestRunner.Default.ExecuteCodeInReleaseMode(code);
- Assert.Contains("false", result.output, StringComparison.OrdinalIgnoreCase);
+ var (output, _) = ScriptTestRunner.Default.ExecuteCodeInReleaseMode(code);
+ Assert.Contains("false", output, StringComparison.OrdinalIgnoreCase);
}
[Fact]
public void ShouldSupportInlineNugetReferencesinEvaluatedCode()
{
var code = @"#r \""nuget: AutoMapper, 6.1.1\"" using AutoMapper; Console.WriteLine(typeof(MapperConfiguration));";
- var result = ScriptTestRunner.Default.ExecuteCode(code);
- Assert.Contains("AutoMapper.MapperConfiguration", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteCode(code);
+ Assert.Contains("AutoMapper.MapperConfiguration", output);
}
[Fact]
public void ShouldSupportInlineNugetReferencesWithTrailingSemicoloninEvaluatedCode()
{
var code = @"#r \""nuget: AutoMapper, 6.1.1\""; using AutoMapper; Console.WriteLine(typeof(MapperConfiguration));";
- var result = ScriptTestRunner.Default.ExecuteCode(code);
- Assert.Contains("AutoMapper.MapperConfiguration", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteCode(code);
+ Assert.Contains("AutoMapper.MapperConfiguration", output);
}
[Theory]
[InlineData("https://gist.githubusercontent.com/seesharper/5d6859509ea8364a1fdf66bbf5b7923d/raw/0a32bac2c3ea807f9379a38e251d93e39c8131cb/HelloWorld.csx",
- "Hello World")]
- [InlineData("https://github.com/filipw/dotnet-script/files/5035247/hello.csx.gz",
+ "Hello World")]
+ [InlineData("http://gist.githubusercontent.com/seesharper/5d6859509ea8364a1fdf66bbf5b7923d/raw/0a32bac2c3ea807f9379a38e251d93e39c8131cb/HelloWorld.csx",
+ "Hello World")]
+ [InlineData("https://github.com/dotnet-script/dotnet-script/files/5035247/hello.csx.gz",
"Hello, world!")]
public void ShouldExecuteRemoteScript(string url, string output)
{
var result = ScriptTestRunner.Default.Execute(url);
- Assert.Contains(output, result.output);
- }
-
- [Fact]
- public void ShouldExecuteRemoteScriptUsingTinyUrl()
- {
- var url = "https://tinyurl.com/y8cda9zt";
- var result = ScriptTestRunner.Default.Execute(url);
- Assert.Contains("Hello World", result.output);
+ Assert.Contains(output, result.Output);
}
[Fact]
public void ShouldHandleIssue268()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue268");
- Assert.Contains("value:", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue268");
+ Assert.Contains("value:", output);
}
[Fact]
public void ShouldHandleIssue435()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue435");
- Assert.Contains("value:Microsoft.Extensions.Configuration.ConfigurationBuilder", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Issue435");
+ Assert.Contains("value:Microsoft.Extensions.Configuration.ConfigurationBuilder", output);
}
[Fact]
public void ShouldLoadMicrosoftExtensionsDependencyInjection()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("MicrosoftExtensionsDependencyInjection");
- Assert.Contains("Microsoft.Extensions.DependencyInjection.IServiceCollection", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("MicrosoftExtensionsDependencyInjection");
+ Assert.Contains("Microsoft.Extensions.DependencyInjection.IServiceCollection", output);
}
[Fact]
public void ShouldThrowExceptionOnInvalidMediaType()
{
- var url = "https://github.com/filipw/dotnet-script/archive/0.20.0.zip";
- var result = ScriptTestRunner.Default.Execute(url);
- Assert.Contains("not supported", result.output);
+ var url = "https://github.com/dotnet-script/dotnet-script/archive/0.20.0.zip";
+ var (output, _) = ScriptTestRunner.Default.Execute(url);
+ Assert.Contains("not supported", output);
}
[Fact]
public void ShouldHandleNonExistingRemoteScript()
{
var url = "https://gist.githubusercontent.com/seesharper/5d6859509ea8364a1fdf66bbf5b7923d/raw/0a32bac2c3ea807f9379a38e251d93e39c8131cb/DoesNotExists.csx";
- var result = ScriptTestRunner.Default.Execute(url);
- Assert.Contains("Not Found", result.output);
+ var (output, _) = ScriptTestRunner.Default.Execute(url);
+ Assert.Contains("Not Found", output);
}
[Fact]
@@ -303,59 +304,55 @@ public void ShouldHandleScriptUsingTheProcessClass()
// This test ensures that we can load the Process class.
// This used to fail when executing a netcoreapp2.0 script
// from dotnet-script built for netcoreapp2.1
- var result = ScriptTestRunner.Default.ExecuteFixture("Process");
- Assert.Contains("Success", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Process");
+ Assert.Contains("Success", output);
}
[Fact]
public void ShouldHandleNuGetVersionRange()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("VersionRange");
- Assert.Contains("AutoMapper.MapperConfiguration", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("VersionRange");
+ Assert.Contains("AutoMapper.MapperConfiguration", output);
}
[Fact]
public void ShouldThrowMeaningfulErrorMessageWhenDependencyIsNotFound()
{
- using (var libraryFolder = new DisposableFolder())
- {
- // Create a package that we can reference
+ using var libraryFolder = new DisposableFolder();
+ // Create a package that we can reference
- ProcessHelper.RunAndCaptureOutput("dotnet", "new classlib -n SampleLibrary", libraryFolder.Path);
- ProcessHelper.RunAndCaptureOutput("dotnet", "pack", libraryFolder.Path);
+ ProcessHelper.RunAndCaptureOutput("dotnet", "new classlib -n SampleLibrary", libraryFolder.Path);
+ ProcessHelper.RunAndCaptureOutput("dotnet", "pack", libraryFolder.Path);
- using (var scriptFolder = new DisposableFolder())
- {
- var code = new StringBuilder();
- code.AppendLine("#r \"nuget:SampleLibrary, 1.0.0\"");
- code.AppendLine("WriteLine(42)");
- var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
- File.WriteAllText(pathToScript, code.ToString());
+ using var scriptFolder = new DisposableFolder();
+ var code = new StringBuilder();
+ code.AppendLine("#r \"nuget:SampleLibrary, 1.0.0\"");
+ code.AppendLine("WriteLine(42)");
+ var pathToScript = Path.Combine(scriptFolder.Path, "main.csx");
+ File.WriteAllText(pathToScript, code.ToString());
- // Run once to ensure that it is cached.
- var result = ScriptTestRunner.Default.Execute(pathToScript);
- Assert.Contains("42", result.output);
+ // Run once to ensure that it is cached.
+ var result = ScriptTestRunner.Default.Execute(pathToScript);
+ Assert.Contains("42", result.Output);
- // Remove the package from the global NuGet cache
- TestPathUtils.RemovePackageFromGlobalNugetCache("SampleLibrary");
+ // Remove the package from the global NuGet cache
+ TestPathUtils.RemovePackageFromGlobalNugetCache("SampleLibrary");
- //ScriptTestRunner.Default.ExecuteInProcess(pathToScript);
+ //ScriptTestRunner.Default.ExecuteInProcess(pathToScript);
- result = ScriptTestRunner.Default.Execute(pathToScript);
- Assert.Contains("Try executing/publishing the script", result.output);
+ result = ScriptTestRunner.Default.Execute(pathToScript);
+ Assert.Contains("Try executing/publishing the script", result.Output);
- // Run again with the '--no-cache' option to assert that the advice actually worked.
- result = ScriptTestRunner.Default.Execute($"{pathToScript} --no-cache");
- Assert.Contains("42", result.output);
- }
- }
+ // Run again with the '--no-cache' option to assert that the advice actually worked.
+ result = ScriptTestRunner.Default.Execute($"{pathToScript} --no-cache");
+ Assert.Contains("42", result.Output);
}
[Fact]
public void ShouldHandleIssue613()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("Issue613");
- Assert.Equal(0, result.exitCode);
+ var (_, exitCode) = ScriptTestRunner.Default.ExecuteFixture("Issue613");
+ Assert.Equal(0, exitCode);
}
[Fact]
@@ -387,19 +384,17 @@ public TestClass()
Console.WriteLine(""Hello World!"");";
- using (var disposableFolder = new DisposableFolder())
- {
- var projectFolder = Path.Combine(disposableFolder.Path, "TestLibrary");
- ProcessHelper.RunAndCaptureOutput("dotnet", "new classlib -n TestLibrary", disposableFolder.Path);
- ProcessHelper.RunAndCaptureOutput("dotnet", "add TestLibrary.csproj package AgileObjects.AgileMapper -v 0.25.0", projectFolder);
- File.WriteAllText(Path.Combine(projectFolder, "Class1.cs"), code);
- File.WriteAllText(Path.Combine(projectFolder, "script.csx"), script);
- ProcessHelper.RunAndCaptureOutput("dotnet", "build -c release -o testlib", projectFolder);
+ using var disposableFolder = new DisposableFolder();
+ var projectFolder = Path.Combine(disposableFolder.Path, "TestLibrary");
+ ProcessHelper.RunAndCaptureOutput("dotnet", "new classlib -n TestLibrary", disposableFolder.Path);
+ ProcessHelper.RunAndCaptureOutput("dotnet", "add TestLibrary.csproj package AgileObjects.AgileMapper -v 0.25.0", projectFolder);
+ File.WriteAllText(Path.Combine(projectFolder, "Class1.cs"), code);
+ File.WriteAllText(Path.Combine(projectFolder, "script.csx"), script);
+ ProcessHelper.RunAndCaptureOutput("dotnet", "build -c release -o testlib", projectFolder);
- var result = ScriptTestRunner.Default.Execute(Path.Combine(projectFolder, "script.csx"));
+ var (output, exitCode) = ScriptTestRunner.Default.Execute(Path.Combine(projectFolder, "script.csx"));
- Assert.Contains("Hello World!", result.output);
- }
+ Assert.Contains("Hello World!", output);
}
@@ -408,15 +403,13 @@ public void ShouldHandleLocalNuGetConfigWithRelativePath()
{
TestPathUtils.RemovePackageFromGlobalNugetCache("NuGetConfigTestLibrary");
- using (var packageLibraryFolder = new DisposableFolder())
- {
- CreateTestPackage(packageLibraryFolder.Path);
+ using var packageLibraryFolder = new DisposableFolder();
+ CreateTestPackage(packageLibraryFolder.Path);
- string pathToScriptFile = CreateTestScript(packageLibraryFolder.Path);
+ string pathToScriptFile = CreateTestScript(packageLibraryFolder.Path);
- var result = ScriptTestRunner.Default.Execute(pathToScriptFile);
- Assert.Contains("Success", result.output);
- }
+ var (output, exitCode) = ScriptTestRunner.Default.Execute(pathToScriptFile);
+ Assert.Contains("Success", output);
}
[Fact]
@@ -424,17 +417,15 @@ public void ShouldHandleLocalNuGetConfigWithRelativePathInParentFolder()
{
TestPathUtils.RemovePackageFromGlobalNugetCache("NuGetConfigTestLibrary");
- using (var packageLibraryFolder = new DisposableFolder())
- {
- CreateTestPackage(packageLibraryFolder.Path);
+ using var packageLibraryFolder = new DisposableFolder();
+ CreateTestPackage(packageLibraryFolder.Path);
- var scriptFolder = Path.Combine(packageLibraryFolder.Path, "ScriptFolder");
- Directory.CreateDirectory(scriptFolder);
- string pathToScriptFile = CreateTestScript(scriptFolder);
+ var scriptFolder = Path.Combine(packageLibraryFolder.Path, "ScriptFolder");
+ Directory.CreateDirectory(scriptFolder);
+ string pathToScriptFile = CreateTestScript(scriptFolder);
- var result = ScriptTestRunner.Default.Execute(pathToScriptFile);
- Assert.Contains("Success", result.output);
- }
+ var (output, exitCode) = ScriptTestRunner.Default.Execute(pathToScriptFile);
+ Assert.Contains("Success", output);
}
[Fact]
@@ -442,25 +433,23 @@ public void ShouldHandleLocalNuGetFileWhenPathContainsSpace()
{
TestPathUtils.RemovePackageFromGlobalNugetCache("NuGetConfigTestLibrary");
- using (var packageLibraryFolder = new DisposableFolder())
- {
- var packageLibraryFolderPath = Path.Combine(packageLibraryFolder.Path, "library folder");
- Directory.CreateDirectory(packageLibraryFolderPath);
+ using var packageLibraryFolder = new DisposableFolder();
+ var packageLibraryFolderPath = Path.Combine(packageLibraryFolder.Path, "library folder");
+ Directory.CreateDirectory(packageLibraryFolderPath);
- CreateTestPackage(packageLibraryFolderPath);
+ CreateTestPackage(packageLibraryFolderPath);
- string pathToScriptFile = CreateTestScript(packageLibraryFolderPath);
+ string pathToScriptFile = CreateTestScript(packageLibraryFolderPath);
- var result = ScriptTestRunner.Default.Execute($"\"{pathToScriptFile}\"");
- Assert.Contains("Success", result.output);
- }
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"\"{pathToScriptFile}\"");
+ Assert.Contains("Success", output);
}
[Fact]
public void ShouldHandleScriptWithTargetFrameworkInShebang()
{
- var result = ScriptTestRunner.Default.ExecuteFixture("TargetFrameworkInShebang");
- Assert.Contains("Hello world!", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("TargetFrameworkInShebang");
+ Assert.Contains("Hello world!", output);
}
[Fact]
@@ -468,10 +457,65 @@ public void ShouldIgnoreGlobalJsonInScriptFolder()
{
var fixture = "InvalidGlobalJson";
var workingDirectory = Path.GetDirectoryName(TestPathUtils.GetPathToTestFixture(fixture));
- var result = ScriptTestRunner.Default.ExecuteFixture("InvalidGlobalJson", $"--no-cache", workingDirectory);
- Assert.Contains("Hello world!", result.output);
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("InvalidGlobalJson", $"--no-cache", workingDirectory);
+ Assert.Contains("Hello world!", output);
+ }
+
+ [Fact]
+ public void ShouldIsolateScriptAssemblies()
+ {
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("Isolation", "--isolated-load-context");
+ Assert.Contains("2.0.0.0", output);
+ }
+
+ [Fact]
+ public void ShouldSetCurrentContextualReflectionContext()
+ {
+ var (output, _) = ScriptTestRunner.Default.ExecuteFixture("CurrentContextualReflectionContext", "--isolated-load-context");
+ Assert.Contains("Dotnet.Script.Core.ScriptAssemblyLoadContext", output);
}
+#if NET6_0
+ [Fact]
+ public void ShouldCompileAndExecuteWithWebSdk()
+ {
+ var processResult = ScriptTestRunner.Default.ExecuteFixture("WebApiNet6", "--no-cache");
+ Assert.Equal(0, processResult.ExitCode);
+ }
+#endif
+
+#if NET7_0
+ [Fact]
+ public void ShouldCompileAndExecuteWithWebSdk()
+ {
+ var processResult = ScriptTestRunner.Default.ExecuteFixture("WebApi", "--no-cache");
+ Assert.Equal(0, processResult.ExitCode);
+ }
+#endif
+
+#if NET8_0
+ // .NET 8.0 only works with isolated load context
+ [Fact]
+ public void ShouldCompileAndExecuteWithWebSdk()
+ {
+ var processResult = ScriptTestRunner.Default.ExecuteFixture("WebApi", "--no-cache");
+ Assert.Equal(0, processResult.ExitCode);
+ }
+#endif
+
+ [Fact]
+ public void ShouldThrowExceptionWhenSdkIsNotSupported()
+ {
+ var processResult = ScriptTestRunner.Default.ExecuteFixture("UnsupportedSdk", "--no-cache");
+ Assert.StartsWith("The sdk 'Unsupported' is not supported", processResult.StandardError);
+ }
+
+ [Fact]
+ public void ShouldRespectEnvironmentExitCodeOfTheScript()
+ {
+ var processResult = ScriptTestRunner.Default.ExecuteFixture("EnvironmentExitCode", "--no-cache");
+ Assert.Equal(0xA0, processResult.ExitCode);
+ }
private static string CreateTestScript(string scriptFolder)
{
diff --git a/src/Dotnet.Script.Tests/ScriptFilesResolverTests.cs b/src/Dotnet.Script.Tests/ScriptFilesResolverTests.cs
index 7de825e1..4d47e02b 100644
--- a/src/Dotnet.Script.Tests/ScriptFilesResolverTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptFilesResolverTests.cs
@@ -10,105 +10,97 @@ public class ScriptFilesResolverTests
[Fact]
public void ShouldOnlyResolveRootScript()
{
- using (var rootFolder = new DisposableFolder())
- {
- var rootScript = WriteScript(string.Empty, rootFolder.Path, "Foo.csx");
- WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
- var scriptFilesResolver = new ScriptFilesResolver();
-
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
-
- Assert.True(files.Count == 1);
- Assert.Contains(files, f => f.Contains("Foo.csx"));
- Assert.Contains(files, f => !f.Contains("Bar.csx"));
- }
+ using var rootFolder = new DisposableFolder();
+ var rootScript = WriteScript(string.Empty, rootFolder.Path, "Foo.csx");
+ WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
+ var scriptFilesResolver = new ScriptFilesResolver();
+
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
+
+ Assert.True(files.Count == 1);
+ Assert.Contains(files, f => f.Contains("Foo.csx"));
+ Assert.Contains(files, f => !f.Contains("Bar.csx"));
}
- [Fact]
- public void ShouldResolveLoadedScriptInRootFolder()
+
+ [Theory]
+ [InlineData("#load \"Bar.csx\"")]
+ // See: https://github.com/dotnet-script/dotnet-script/issues/720 (1)
+ [InlineData("#load \"Bar.csx\"\n\n\"Hello world\".Dump();")]
+ public void ShouldResolveLoadedScriptInRootFolder(string rootScriptContent)
{
- using (var rootFolder = new DisposableFolder())
- {
- var rootScript = WriteScript("#load \"Bar.csx\"", rootFolder.Path, "Foo.csx");
- WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
- var scriptFilesResolver = new ScriptFilesResolver();
-
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
-
- Assert.True(files.Count == 2);
- Assert.Contains(files, f => f.Contains("Foo.csx"));
- Assert.Contains(files, f => f.Contains("Bar.csx"));
- }
+ using var rootFolder = new DisposableFolder();
+ var rootScript = WriteScript(rootScriptContent, rootFolder.Path, "Foo.csx");
+ WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
+ var scriptFilesResolver = new ScriptFilesResolver();
+
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
+
+ Assert.True(files.Count == 2);
+ Assert.Contains(files, f => f.Contains("Foo.csx"));
+ Assert.Contains(files, f => f.Contains("Bar.csx"));
}
[Fact]
public void ShouldResolveLoadedScriptInSubFolder()
{
- using (var rootFolder = new DisposableFolder())
- {
- var rootScript = WriteScript("#load \"SubFolder/Bar.csx\"", rootFolder.Path, "Foo.csx");
- var subFolder = Path.Combine(rootFolder.Path, "SubFolder");
- Directory.CreateDirectory(subFolder);
- WriteScript(string.Empty, subFolder, "Bar.csx");
-
- var scriptFilesResolver = new ScriptFilesResolver();
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
-
- Assert.True(files.Count == 2);
- Assert.Contains(files, f => f.Contains("Foo.csx"));
- Assert.Contains(files, f => f.Contains("Bar.csx"));
- }
+ using var rootFolder = new DisposableFolder();
+ var rootScript = WriteScript("#load \"SubFolder/Bar.csx\"", rootFolder.Path, "Foo.csx");
+ var subFolder = Path.Combine(rootFolder.Path, "SubFolder");
+ Directory.CreateDirectory(subFolder);
+ WriteScript(string.Empty, subFolder, "Bar.csx");
+
+ var scriptFilesResolver = new ScriptFilesResolver();
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
+
+ Assert.True(files.Count == 2);
+ Assert.Contains(files, f => f.Contains("Foo.csx"));
+ Assert.Contains(files, f => f.Contains("Bar.csx"));
}
[Fact]
public void ShouldResolveLoadedScriptWithRootPath()
{
- using (var rootFolder = new DisposableFolder())
- {
- var subFolder = Path.Combine(rootFolder.Path, "SubFolder");
- Directory.CreateDirectory(subFolder);
- var fullPathToBarScript = WriteScript(string.Empty, subFolder, "Bar.csx");
- var rootScript = WriteScript($"#load \"{fullPathToBarScript}\"", rootFolder.Path, "Foo.csx");
+ using var rootFolder = new DisposableFolder();
+ var subFolder = Path.Combine(rootFolder.Path, "SubFolder");
+ Directory.CreateDirectory(subFolder);
+ var fullPathToBarScript = WriteScript(string.Empty, subFolder, "Bar.csx");
+ var rootScript = WriteScript($"#load \"{fullPathToBarScript}\"", rootFolder.Path, "Foo.csx");
- var scriptFilesResolver = new ScriptFilesResolver();
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
+ var scriptFilesResolver = new ScriptFilesResolver();
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
- Assert.True(files.Count == 2);
- Assert.Contains(files, f => f.Contains("Foo.csx"));
- Assert.Contains(files, f => f.Contains("Bar.csx"));
- }
+ Assert.True(files.Count == 2);
+ Assert.Contains(files, f => f.Contains("Foo.csx"));
+ Assert.Contains(files, f => f.Contains("Bar.csx"));
}
[Fact]
public void ShouldNotParseLoadDirectiveIgnoringCase()
{
- using (var rootFolder = new DisposableFolder())
- {
- var rootScript = WriteScript("#LOAD \"Bar.csx\"", rootFolder.Path, "Foo.csx");
- WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
- var scriptFilesResolver = new ScriptFilesResolver();
+ using var rootFolder = new DisposableFolder();
+ var rootScript = WriteScript("#LOAD \"Bar.csx\"", rootFolder.Path, "Foo.csx");
+ WriteScript(string.Empty, rootFolder.Path, "Bar.csx");
+ var scriptFilesResolver = new ScriptFilesResolver();
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
- Assert.Equal(new[] { "Foo.csx" }, files.Select(Path.GetFileName));
- }
+ Assert.Equal(new[] { "Foo.csx" }, files.Select(Path.GetFileName));
}
[Fact]
public void ShouldParseFilesStartingWithNuGet()
{
- using (var rootFolder = new DisposableFolder())
- {
- var rootScript = WriteScript("#load \"NuGet.csx\"", rootFolder.Path, "Foo.csx");
- WriteScript(string.Empty, rootFolder.Path, "NuGet.csx");
- var scriptFilesResolver = new ScriptFilesResolver();
-
- var files = scriptFilesResolver.GetScriptFiles(rootScript);
-
- Assert.True(files.Count == 2);
- Assert.Contains(files, f => f.Contains("Foo.csx"));
- Assert.Contains(files, f => f.Contains("NuGet.csx"));
- }
+ using var rootFolder = new DisposableFolder();
+ var rootScript = WriteScript("#load \"NuGet.csx\"", rootFolder.Path, "Foo.csx");
+ WriteScript(string.Empty, rootFolder.Path, "NuGet.csx");
+ var scriptFilesResolver = new ScriptFilesResolver();
+
+ var files = scriptFilesResolver.GetScriptFiles(rootScript);
+
+ Assert.True(files.Count == 2);
+ Assert.Contains(files, f => f.Contains("Foo.csx"));
+ Assert.Contains(files, f => f.Contains("NuGet.csx"));
}
private static string WriteScript(string content, string folder, string name)
diff --git a/src/Dotnet.Script.Tests/ScriptPackagesFixture.cs b/src/Dotnet.Script.Tests/ScriptPackagesFixture.cs
index 1dadbdc2..51870f10 100644
--- a/src/Dotnet.Script.Tests/ScriptPackagesFixture.cs
+++ b/src/Dotnet.Script.Tests/ScriptPackagesFixture.cs
@@ -35,7 +35,7 @@ private void BuildScriptPackages()
RemoveDirectory(pathToPackagesOutputFolder);
Directory.CreateDirectory(pathToPackagesOutputFolder);
var specFiles = GetSpecFiles();
- var baseDirectory = AppDomain.CurrentDomain.BaseDirectory;
+ _ = AppDomain.CurrentDomain.BaseDirectory;
var pathtoNuget430 = Path.Combine("../../../NuGet/NuGet430.exe");
foreach (var specFile in specFiles)
{
@@ -43,12 +43,12 @@ private void BuildScriptPackages()
if (_scriptEnvironment.IsWindows)
{
command = pathtoNuget430;
- var result = ProcessHelper.RunAndCaptureOutput(command, $"pack {specFile} -OutputDirectory {pathToPackagesOutputFolder}");
+ _ = ProcessHelper.RunAndCaptureOutput(command, $"pack \"{specFile}\" -OutputDirectory \"{pathToPackagesOutputFolder}\"");
}
else
{
command = "mono";
- var result = ProcessHelper.RunAndCaptureOutput(command, $"{pathtoNuget430} pack {specFile} -OutputDirectory {pathToPackagesOutputFolder}");
+ _ = ProcessHelper.RunAndCaptureOutput(command, $"\"{pathtoNuget430}\" pack \"{specFile}\" -OutputDirectory \"{pathToPackagesOutputFolder}\"");
}
}
diff --git a/src/Dotnet.Script.Tests/ScriptPackagesTests.cs b/src/Dotnet.Script.Tests/ScriptPackagesTests.cs
index aeb3bebd..81e61618 100644
--- a/src/Dotnet.Script.Tests/ScriptPackagesTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptPackagesTests.cs
@@ -2,8 +2,6 @@
using System.IO;
using System.Linq;
using System.Text;
-using Dotnet.Script.DependencyModel.Compilation;
-using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Runtime;
using Dotnet.Script.Shared.Tests;
using Xunit;
@@ -14,12 +12,9 @@ namespace Dotnet.Script.Tests
[Collection("IntegrationTests")]
public class ScriptPackagesTests : IClassFixture
{
- private readonly ScriptEnvironment _scriptEnvironment;
-
public ScriptPackagesTests(ITestOutputHelper testOutputHelper)
{
testOutputHelper.Capture();
- _scriptEnvironment = ScriptEnvironment.Default;
}
[Fact]
@@ -30,32 +25,30 @@ public void ShouldHandleScriptPackageWithMainCsx()
Assert.StartsWith("Hello from netstandard2.0", output);
}
- //[Fact]
+ [Fact(Skip = "Needs review.")]
public void ShouldThrowMeaningfulExceptionWhenScriptPackageIsMissing()
{
- using (var scriptFolder = new DisposableFolder())
- {
- var code = new StringBuilder();
- code.AppendLine("#load \"nuget:ScriptPackageWithMainCsx, 1.0.0\"");
- code.AppendLine("SayHello();");
- var pathToScriptFile = Path.Combine(scriptFolder.Path, "main.csx");
- File.WriteAllText(pathToScriptFile, code.ToString());
- var pathToScriptPackages = Path.GetFullPath(ScriptPackagesFixture.GetPathToPackagesFolder());
-
- // Run once to ensure that it is cached.
- var result = ScriptTestRunner.Default.Execute($"{pathToScriptFile} -s {pathToScriptPackages}");
- Assert.StartsWith("Hello from netstandard2.0", result.output);
-
- // Remove the package from the global NuGet cache
- TestPathUtils.RemovePackageFromGlobalNugetCache("ScriptPackageWithMainCsx");
-
- //Change the source to force a recompile, now with the missing package.
- code.Append("return 0;");
- File.WriteAllText(pathToScriptFile, code.ToString());
-
- result = ScriptTestRunner.Default.Execute($"{pathToScriptFile} -s {pathToScriptPackages}");
- Assert.Contains("Try executing/publishing the script", result.output);
- }
+ using var scriptFolder = new DisposableFolder();
+ var code = new StringBuilder();
+ code.AppendLine("#load \"nuget:ScriptPackageWithMainCsx, 1.0.0\"");
+ code.AppendLine("SayHello();");
+ var pathToScriptFile = Path.Combine(scriptFolder.Path, "main.csx");
+ File.WriteAllText(pathToScriptFile, code.ToString());
+ var pathToScriptPackages = Path.GetFullPath(ScriptPackagesFixture.GetPathToPackagesFolder());
+
+ // Run once to ensure that it is cached.
+ var result = ScriptTestRunner.Default.Execute($"{pathToScriptFile} -s {pathToScriptPackages}");
+ Assert.StartsWith("Hello from netstandard2.0", result.Output);
+
+ // Remove the package from the global NuGet cache
+ TestPathUtils.RemovePackageFromGlobalNugetCache("ScriptPackageWithMainCsx");
+
+ //Change the source to force a recompile, now with the missing package.
+ code.Append("return 0;");
+ File.WriteAllText(pathToScriptFile, code.ToString());
+
+ result = ScriptTestRunner.Default.Execute($"{pathToScriptFile} -s {pathToScriptPackages}");
+ Assert.Contains("Try executing/publishing the script", result.Output);
}
[Fact]
diff --git a/src/Dotnet.Script.Tests/ScriptProjectProviderTests.cs b/src/Dotnet.Script.Tests/ScriptProjectProviderTests.cs
index 46f530e7..fdc3bc03 100644
--- a/src/Dotnet.Script.Tests/ScriptProjectProviderTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptProjectProviderTests.cs
@@ -1,5 +1,7 @@
using System.IO;
+using System.Linq;
using System.Text;
+using System.Xml.Linq;
using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.ProjectSystem;
using Dotnet.Script.Shared.Tests;
@@ -30,5 +32,25 @@ public void ShouldLogProjectFileContent()
Assert.Contains("", output);
}
+
+ [Fact]
+ public void ShouldUseSpecifiedSdk()
+ {
+ var provider = new ScriptProjectProvider(TestOutputHelper.CreateTestLogFactory());
+ var projectFileInfo = provider.CreateProject(TestPathUtils.GetPathToTestFixtureFolder("WebApi"), _scriptEnvironment.TargetFramework, true);
+ Assert.Equal("Microsoft.NET.Sdk.Web", XDocument.Load(projectFileInfo.Path).Descendants("Project").Single().Attributes("Sdk").Single().Value);
+ }
+
+ // See: https://github.com/dotnet-script/dotnet-script/issues/723
+ [Theory]
+ [InlineData("#!/usr/bin/env dotnet-script\n#r \"sdk:Microsoft.NET.Sdk.Web\"")]
+ [InlineData("#!/usr/bin/env dotnet-script\n\n#r \"sdk:Microsoft.NET.Sdk.Web\"")]
+ public void ShouldHandleShebangBeforeSdk(string code)
+ {
+ var parser = new ScriptParser(TestOutputHelper.CreateTestLogFactory());
+ var result = parser.ParseFromCode(code);
+
+ Assert.Equal("Microsoft.NET.Sdk.Web", result.Sdk);
+ }
}
}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/ScriptPublisherTests.cs b/src/Dotnet.Script.Tests/ScriptPublisherTests.cs
index 24f39c16..80da0361 100644
--- a/src/Dotnet.Script.Tests/ScriptPublisherTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptPublisherTests.cs
@@ -1,5 +1,4 @@
-using Dotnet.Script.Core;
-using Dotnet.Script.DependencyModel.Environment;
+using Dotnet.Script.DependencyModel.Environment;
using Dotnet.Script.DependencyModel.Logging;
using Dotnet.Script.DependencyModel.Process;
using Dotnet.Script.Shared.Tests;
@@ -26,257 +25,231 @@ public ScriptPublisherTests(ITestOutputHelper testOutputHelper)
[Fact]
public void SimplePublishTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
-
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath}", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var exePath = Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier, "main");
- var executableRunResult = _commandRunner.Execute(exePath);
-
- Assert.Equal(0, executableRunResult);
-
- var publishedFiles = Directory.EnumerateFiles(Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier));
- if (_scriptEnvironment.NetCoreVersion.Major >= 3)
- Assert.True(publishedFiles.Count() == 1, "There should be only a single published file");
- else
- Assert.True(publishedFiles.Count() > 1, "There should be multiple published files");
- }
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath}", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var exePath = Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier, "main");
+ var executableRunResult = _commandRunner.Execute(exePath);
+
+ Assert.Equal(0, executableRunResult);
+
+ var publishedFiles = Directory.EnumerateFiles(Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier));
+ if (_scriptEnvironment.NetCoreVersion.Major >= 3)
+ Assert.True(publishedFiles.Count() == 1, "There should be only a single published file");
+ else
+ Assert.True(publishedFiles.Count() > 1, "There should be multiple published files");
}
[Fact]
public void SimplePublishTestToDifferentRuntimeId()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var runtimeId = _scriptEnvironment.RuntimeIdentifier == "win10-x64" ? "osx-x64" : "win10-x64";
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath} --runtime {runtimeId}");
-
- Assert.Equal(0, publishResult.exitCode);
-
- var publishPath = Path.Combine(workspaceFolder.Path, "publish", runtimeId);
- Assert.True(Directory.Exists(publishPath), $"Publish directory {publishPath} was not found.");
-
- using (var enumerator = Directory.EnumerateFiles(publishPath).GetEnumerator())
- {
- Assert.True(enumerator.MoveNext(), $"Publish directory {publishPath} was empty.");
- }
- }
+ using var workspaceFolder = new DisposableFolder();
+#if NET8_0
+ var runtimeId = _scriptEnvironment.RuntimeIdentifier == "win-x64" ? "osx-x64" : "win10-x64";
+#else
+ var runtimeId = _scriptEnvironment.RuntimeIdentifier == "win10-x64" ? "osx-x64" : "win10-x64";
+#endif
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath} --runtime {runtimeId}");
+
+ Assert.Equal(0, exitCode);
+
+ var publishPath = Path.Combine(workspaceFolder.Path, "publish", runtimeId);
+ Assert.True(Directory.Exists(publishPath), $"Publish directory {publishPath} was not found.");
+
+ using var enumerator = Directory.EnumerateFiles(publishPath).GetEnumerator();
+ Assert.True(enumerator.MoveNext(), $"Publish directory {publishPath} was empty.");
}
[Fact]
public void SimplePublishToOtherFolderTest()
{
- using (var workspaceFolder = new DisposableFolder())
- using (var publishRootFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath} -o {publishRootFolder.Path}");
- Assert.Equal(0, publishResult.exitCode);
-
- var exePath = Path.Combine(publishRootFolder.Path, "main");
- var executableRunResult = _commandRunner.Execute(exePath);
-
- Assert.Equal(0, executableRunResult);
- }
+ using var workspaceFolder = new DisposableFolder();
+ using var publishRootFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath} -o {publishRootFolder.Path}");
+ Assert.Equal(0, exitCode);
+
+ var exePath = Path.Combine(publishRootFolder.Path, "main");
+ var executableRunResult = _commandRunner.Execute(exePath);
+
+ Assert.Equal(0, executableRunResult);
}
[Fact]
public void SimplePublishFromCurrentDirectoryTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute("publish main.csx", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var exePath = Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier, "main");
- var executableRunResult = _commandRunner.Execute(exePath);
-
- Assert.Equal(0, executableRunResult);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("publish main.csx", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var exePath = Path.Combine(workspaceFolder.Path, "publish", _scriptEnvironment.RuntimeIdentifier, "main");
+ var executableRunResult = _commandRunner.Execute(exePath);
+
+ Assert.Equal(0, executableRunResult);
}
[Fact]
public void SimplePublishFromCurrentDirectoryToOtherFolderTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute("publish main.csx -o publish", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var exePath = Path.Combine(workspaceFolder.Path, "publish", "main");
- var executableRunResult = _commandRunner.Execute(exePath);
-
- Assert.Equal(0, executableRunResult);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("publish main.csx -o publish", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var exePath = Path.Combine(workspaceFolder.Path, "publish", "main");
+ var executableRunResult = _commandRunner.Execute(exePath);
+
+ Assert.Equal(0, executableRunResult);
}
[Fact]
public void SimplePublishDllTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var dllPath = Path.Combine("publish", "main.dll");
- var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
-
- Assert.Equal(0, dllRunResult.exitCode);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var dllPath = Path.Combine("publish", "main.dll");
+ var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
+
+ Assert.Equal(0, dllRunResult.ExitCode);
}
[Fact]
public void SimplePublishDllFromCurrentDirectoryTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute("publish main.csx --dll", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("publish main.csx --dll", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
- var dllPath = Path.Combine(workspaceFolder.Path, "publish", "main.dll");
+ var dllPath = Path.Combine(workspaceFolder.Path, "publish", "main.dll");
- var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
+ var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
- Assert.Equal(0, dllRunResult.exitCode);
- }
+ Assert.Equal(0, dllRunResult.ExitCode);
}
[Fact]
public void SimplePublishDllToOtherFolderTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- using (var publishFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll -o {publishFolder.Path}", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var dllPath = Path.Combine(publishFolder.Path, "main.dll");
- var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", publishFolder.Path);
-
- Assert.Equal(0, dllRunResult.exitCode);
- }
- }
+ using var workspaceFolder = new DisposableFolder();
+ using var publishFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll -o {publishFolder.Path}", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var dllPath = Path.Combine(publishFolder.Path, "main.dll");
+ var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", publishFolder.Path);
+
+ Assert.Equal(0, dllRunResult.ExitCode);
}
[Fact]
public void CustomDllNameTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var outputName = "testName";
- var assemblyName = $"{outputName}.dll";
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish main.csx --dll -n {outputName}", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var dllPath = Path.Combine(workspaceFolder.Path, "publish", assemblyName);
- var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
-
- Assert.Equal(0, dllRunResult.exitCode);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var outputName = "testName";
+ var assemblyName = $"{outputName}.dll";
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish main.csx --dll -n {outputName}", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var dllPath = Path.Combine(workspaceFolder.Path, "publish", assemblyName);
+ var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath}", workspaceFolder.Path);
+
+ Assert.Equal(0, dllRunResult.ExitCode);
}
[Fact]
public void CustomExeNameTest()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var exeName = "testName";
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish main.csx -o publish -n {exeName}", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var exePath = Path.Combine(workspaceFolder.Path, "publish", exeName);
- var executableRunResult = _commandRunner.Execute(exePath);
-
- Assert.Equal(0, executableRunResult);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var exeName = "testName";
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish main.csx -o publish -n {exeName}", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var exePath = Path.Combine(workspaceFolder.Path, "publish", exeName);
+ var executableRunResult = _commandRunner.Execute(exePath);
+
+ Assert.Equal(0, executableRunResult);
}
[Fact]
public void DllWithArgsTests()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""Hello "" + Args[0] + Args[1] + Args[2] + Args[3] + Args[4]);";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
-
- var dllPath = Path.Combine(workspaceFolder.Path, "publish", "main.dll");
- var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath} -- w o r l d", workspaceFolder.Path);
-
- Assert.Equal(0, dllRunResult.exitCode);
- Assert.Contains("Hello world", dllRunResult.output);
- }
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""Hello "" + Args[0] + Args[1] + Args[2] + Args[3] + Args[4]);";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute($"publish {mainPath} --dll", workspaceFolder.Path);
+ Assert.Equal(0, exitCode);
+
+ var dllPath = Path.Combine(workspaceFolder.Path, "publish", "main.dll");
+ var dllRunResult = ScriptTestRunner.Default.Execute($"exec {dllPath} -- w o r l d", workspaceFolder.Path);
+
+ Assert.Equal(0, dllRunResult.ExitCode);
+ Assert.Contains("Hello world", dllRunResult.Output);
}
[Fact]
public void ShouldHandleReferencingAssemblyFromScriptFolder()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- ProcessHelper.RunAndCaptureOutput($"dotnet", $" new classlib -n MyCustomLibrary -o {workspaceFolder.Path}");
- ProcessHelper.RunAndCaptureOutput($"dotnet", $" build -o {workspaceFolder.Path}", workspaceFolder.Path);
- var code = $@"#r ""MyCustomLibrary.dll"" {Environment.NewLine} WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
-
- var publishResult = ScriptTestRunner.Default.Execute("publish main.csx --dll --output .", workspaceFolder.Path);
-
- Assert.Equal(0, publishResult.exitCode);
- }
+ using var workspaceFolder = new DisposableFolder();
+ ProcessHelper.RunAndCaptureOutput($"dotnet", $" new classlib -n MyCustomLibrary -o {workspaceFolder.Path}");
+ ProcessHelper.RunAndCaptureOutput($"dotnet", $" build -o {workspaceFolder.Path}", workspaceFolder.Path);
+ var code = $@"#r ""MyCustomLibrary.dll"" {Environment.NewLine} WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
+
+ var (output, exitCode) = ScriptTestRunner.Default.Execute("publish main.csx --dll --output .", workspaceFolder.Path);
+
+ Assert.Equal(0, exitCode);
}
[Fact]
public void ShouldHandleSpaceInPublishFolder()
{
- using (var workspaceFolder = new DisposableFolder())
- {
- var code = @"WriteLine(""hello world"");";
- var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
- File.WriteAllText(mainPath, code);
+ using var workspaceFolder = new DisposableFolder();
+ var code = @"WriteLine(""hello world"");";
+ var mainPath = Path.Combine(workspaceFolder.Path, "main.csx");
+ File.WriteAllText(mainPath, code);
- var publishResult = ScriptTestRunner.Default.Execute(@"publish main.csx -o ""publish folder""", workspaceFolder.Path);
+ var (output, exitCode) = ScriptTestRunner.Default.Execute(@"publish main.csx -o ""publish folder""", workspaceFolder.Path);
- Assert.Equal(0, publishResult.exitCode);
+ Assert.Equal(0, exitCode);
- var exePath = Path.Combine(workspaceFolder.Path, "publish folder", "main");
- var executableRunResult = _commandRunner.Execute(exePath);
+ var exePath = Path.Combine(workspaceFolder.Path, "publish folder", "main");
+ var executableRunResult = _commandRunner.Execute(exePath);
- Assert.Equal(0, executableRunResult);
- }
+ Assert.Equal(0, executableRunResult);
}
private LogFactory GetLogFactory()
diff --git a/src/Dotnet.Script.Tests/ScriptRunnerTests.cs b/src/Dotnet.Script.Tests/ScriptRunnerTests.cs
index 913ad33d..e9bc7de3 100644
--- a/src/Dotnet.Script.Tests/ScriptRunnerTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptRunnerTests.cs
@@ -1,8 +1,10 @@
using System;
using System.Collections.Generic;
+using System.Reflection;
using Dotnet.Script.Core;
using Dotnet.Script.DependencyModel.Runtime;
using Dotnet.Script.Shared.Tests;
+using Gapotchenko.FX.Reflection;
using Moq;
using Xunit;
@@ -15,16 +17,16 @@ public void ResolveAssembly_ReturnsNull_WhenRuntimeDepsMapDoesNotContainAssembly
{
var scriptRunner = CreateScriptRunner();
- var result = scriptRunner.ResolveAssembly(new ResolveEventArgs("AnyAssemblyName"), new Dictionary());
+ var result = scriptRunner.ResolveAssembly(AssemblyLoadPal.ForCurrentAppDomain, new AssemblyName("AnyAssemblyName"), new Dictionary());
Assert.Null(result);
}
- private ScriptRunner CreateScriptRunner()
+ private static ScriptRunner CreateScriptRunner()
{
var logFactory = TestOutputHelper.CreateTestLogFactory();
var scriptCompiler = new ScriptCompiler(logFactory, false);
-
+
return new ScriptRunner(scriptCompiler, logFactory, ScriptConsole.Default);
}
}
diff --git a/src/Dotnet.Script.Tests/ScriptTestRunner.cs b/src/Dotnet.Script.Tests/ScriptTestRunner.cs
index ebb1d0d7..02db0fe5 100644
--- a/src/Dotnet.Script.Tests/ScriptTestRunner.cs
+++ b/src/Dotnet.Script.Tests/ScriptTestRunner.cs
@@ -11,7 +11,7 @@ public class ScriptTestRunner
{
public static readonly ScriptTestRunner Default = new ScriptTestRunner();
- private ScriptEnvironment _scriptEnvironment;
+ private readonly ScriptEnvironment _scriptEnvironment;
static ScriptTestRunner()
{
@@ -24,7 +24,7 @@ private ScriptTestRunner()
_scriptEnvironment = ScriptEnvironment.Default;
}
- public (string output, int exitCode) Execute(string arguments, string workingDirectory = null)
+ public ProcessResult Execute(string arguments, string workingDirectory = null)
{
var result = ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments(arguments), workingDirectory);
return result;
@@ -35,21 +35,21 @@ public int ExecuteInProcess(string arguments = null)
return Program.Main(arguments?.Split(" ") ?? Array.Empty());
}
- public (string output, int exitCode) ExecuteFixture(string fixture, string arguments = null, string workingDirectory = null)
+ public ProcessResult ExecuteFixture(string fixture, string arguments = null, string workingDirectory = null)
{
var pathToFixture = TestPathUtils.GetPathToTestFixture(fixture);
- var result = ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"{pathToFixture} {arguments}"), workingDirectory);
+ var result = ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"\"{pathToFixture}\" {arguments}"), workingDirectory);
return result;
}
- public (string output, int exitcode) ExecuteWithScriptPackage(string fixture, string arguments = null, string workingDirectory = null)
+ public ProcessResult ExecuteWithScriptPackage(string fixture, string arguments = null, string workingDirectory = null)
{
var pathToScriptPackageFixtures = TestPathUtils.GetPathToTestFixtureFolder("ScriptPackage");
var pathToFixture = Path.Combine(pathToScriptPackageFixtures, fixture, $"{fixture}.csx");
- return ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"{pathToFixture} {arguments}"), workingDirectory);
+ return ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"\"{pathToFixture}\" {arguments}"), workingDirectory);
}
- public int ExecuteFixtureInProcess(string fixture, string arguments = null)
+ public static int ExecuteFixtureInProcess(string fixture, string arguments = null)
{
var pathToFixture = TestPathUtils.GetPathToTestFixture(fixture);
return Program.Main(new[] { pathToFixture }.Concat(arguments?.Split(" ") ?? Array.Empty()).ToArray());
@@ -67,13 +67,13 @@ public static int ExecuteCodeInProcess(string code, string arguments)
return Program.Main(allArguments.ToArray());
}
- public (string output, int exitCode) ExecuteCode(string code)
+ public ProcessResult ExecuteCode(string code)
{
var result = ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"eval \"{code}\""));
return result;
}
- public (string output, int exitCode) ExecuteCodeInReleaseMode(string code)
+ public ProcessResult ExecuteCodeInReleaseMode(string code)
{
var result = ProcessHelper.RunAndCaptureOutput("dotnet", GetDotnetScriptArguments($"-c release eval \"{code}\""));
return result;
@@ -88,7 +88,7 @@ private string GetDotnetScriptArguments(string arguments)
configuration = "Release";
#endif
- var allArgs = $"exec {Path.Combine(Directory.GetCurrentDirectory(), "..", "..", "..", "..", "Dotnet.Script", "bin", configuration, _scriptEnvironment.TargetFramework, "dotnet-script.dll")} {arguments}";
+ var allArgs = $"exec \"{Path.Combine(Directory.GetCurrentDirectory(), "..", "..", "..", "..", "Dotnet.Script", "bin", configuration, _scriptEnvironment.TargetFramework, "dotnet-script.dll")}\" {arguments}";
return allArgs;
}
diff --git a/src/Dotnet.Script.Tests/ScriptdependencyContextReaderTests.cs b/src/Dotnet.Script.Tests/ScriptdependencyContextReaderTests.cs
index 4746a0b9..401fe96f 100644
--- a/src/Dotnet.Script.Tests/ScriptdependencyContextReaderTests.cs
+++ b/src/Dotnet.Script.Tests/ScriptdependencyContextReaderTests.cs
@@ -18,16 +18,14 @@ public ScriptDependencyContextReaderTests(ITestOutputHelper testOutputHelper)
[Fact]
public void ShouldThrowMeaningfulExceptionWhenRuntimeTargetIsMissing()
{
- using (var projectFolder = new DisposableFolder())
- {
- ProcessHelper.RunAndCaptureOutput("dotnet", "new console -n SampleLibrary", projectFolder.Path);
- ProcessHelper.RunAndCaptureOutput("dotnet", "restore", projectFolder.Path);
- var pathToAssetsFile = Path.Combine(projectFolder.Path, "SampleLibrary" ,"obj","project.assets.json");
- var dependencyResolver = new ScriptDependencyContextReader(TestOutputHelper.CreateTestLogFactory());
+ using var projectFolder = new DisposableFolder();
+ ProcessHelper.RunAndCaptureOutput("dotnet", "new console -n SampleLibrary", projectFolder.Path);
+ ProcessHelper.RunAndCaptureOutput("dotnet", "restore", projectFolder.Path);
+ var pathToAssetsFile = Path.Combine(projectFolder.Path, "SampleLibrary", "obj", "project.assets.json");
+ var dependencyResolver = new ScriptDependencyContextReader(TestOutputHelper.CreateTestLogFactory());
- var exception = Assert.Throws(() => dependencyResolver.ReadDependencyContext(pathToAssetsFile));
- Assert.Contains("Make sure that the project file was restored using a RID (runtime identifier).", exception.Message);
- }
+ var exception = Assert.Throws(() => dependencyResolver.ReadDependencyContext(pathToAssetsFile));
+ Assert.Contains("Make sure that the project file was restored using a RID (runtime identifier).", exception.Message);
}
[Fact]
diff --git a/src/Dotnet.Script.Tests/TestFixtures/CompilationWarning/CompilationWarning.csx b/src/Dotnet.Script.Tests/TestFixtures/CompilationWarning/CompilationWarning.csx
new file mode 100644
index 00000000..883f543a
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/CompilationWarning/CompilationWarning.csx
@@ -0,0 +1,3 @@
+public async Task Foo()
+{
+}
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/TestFixtures/CurrentContextualReflectionContext/CurrentContextualReflectionContext.csx b/src/Dotnet.Script.Tests/TestFixtures/CurrentContextualReflectionContext/CurrentContextualReflectionContext.csx
new file mode 100644
index 00000000..e7e2420e
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/CurrentContextualReflectionContext/CurrentContextualReflectionContext.csx
@@ -0,0 +1,4 @@
+using System.Runtime.Loader;
+
+var context = AssemblyLoadContext.CurrentContextualReflectionContext;
+Console.WriteLine(context?.ToString() ?? "");
diff --git a/src/Dotnet.Script.Tests/TestFixtures/EnvironmentExitCode/EnvironmentExitCode.csx b/src/Dotnet.Script.Tests/TestFixtures/EnvironmentExitCode/EnvironmentExitCode.csx
new file mode 100644
index 00000000..ab8f26d5
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/EnvironmentExitCode/EnvironmentExitCode.csx
@@ -0,0 +1,2 @@
+Environment.ExitCode = 0xA0;
+Console.WriteLine("Hello World");
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/TestFixtures/Isolation/Isolation.csx b/src/Dotnet.Script.Tests/TestFixtures/Isolation/Isolation.csx
new file mode 100644
index 00000000..8d2d2427
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/Isolation/Isolation.csx
@@ -0,0 +1,3 @@
+#r "nuget: Microsoft.Extensions.Logging.Console, 2.0.0"
+using Microsoft.Extensions.Logging;
+Console.WriteLine(typeof(ConsoleLoggerExtensions).Assembly.GetName().Version);
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/TestFixtures/UnsupportedSdk/UnsupportedSdk.csx b/src/Dotnet.Script.Tests/TestFixtures/UnsupportedSdk/UnsupportedSdk.csx
new file mode 100644
index 00000000..4f30c5e3
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/UnsupportedSdk/UnsupportedSdk.csx
@@ -0,0 +1 @@
+#r "sdk:Unsupported"
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/TestFixtures/WebApi/WebApi.csx b/src/Dotnet.Script.Tests/TestFixtures/WebApi/WebApi.csx
new file mode 100644
index 00000000..ff851bad
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/WebApi/WebApi.csx
@@ -0,0 +1,8 @@
+#r "sdk:Microsoft.NET.Sdk.Web"
+
+using Microsoft.AspNetCore.Builder;
+
+var builder = WebApplication.CreateBuilder();
+var app = builder.Build();
+
+app.MapGet("/", () => "Hello World!");
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/TestFixtures/WebApiNet6/WebApiNet6.csx b/src/Dotnet.Script.Tests/TestFixtures/WebApiNet6/WebApiNet6.csx
new file mode 100644
index 00000000..b4b76d41
--- /dev/null
+++ b/src/Dotnet.Script.Tests/TestFixtures/WebApiNet6/WebApiNet6.csx
@@ -0,0 +1,6 @@
+#r "sdk:Microsoft.NET.Sdk.Web"
+
+using Microsoft.AspNetCore.Builder;
+
+var a = WebApplication.Create();
+a.MapGet("/", () => "Hello world");
\ No newline at end of file
diff --git a/src/Dotnet.Script.Tests/VersioningTests.cs b/src/Dotnet.Script.Tests/VersioningTests.cs
index 6313547a..346ed3ca 100644
--- a/src/Dotnet.Script.Tests/VersioningTests.cs
+++ b/src/Dotnet.Script.Tests/VersioningTests.cs
@@ -50,7 +50,7 @@ public async Task ShouldNotReportLatestVersionWhenAlreayRunningLatest()
Assert.DoesNotContain("Version Y is now available", output.ToString());
}
- private async Task ReportWith(string currentVersion, string latestVersion)
+ private static async Task ReportWith(string currentVersion, string latestVersion)
{
var versionProviderMock = new Mock();
versionProviderMock.Setup(m => m.GetCurrentVersion()).Returns(new VersionInfo(currentVersion, true));
@@ -71,10 +71,10 @@ private async Task ReportWith(string currentVersion, string latestVersio
public async Task ShouldLogErrorMessageWhenResolvingLatestVersionFails()
{
StringWriter log = new StringWriter();
- LogFactory logFactory = (type) => (level,message,exception) =>
- {
- log.WriteLine(message);
- };
+ Logger logFactory(Type type) => (level, message, exception) =>
+ {
+ log.WriteLine(message);
+ };
var versionProviderMock = new Mock();
versionProviderMock.Setup(m => m.GetCurrentVersion()).Returns(new VersionInfo("X", true));
diff --git a/src/Dotnet.Script/Dotnet.Script.csproj b/src/Dotnet.Script/Dotnet.Script.csproj
index 7cbd6249..e33e4619 100644
--- a/src/Dotnet.Script/Dotnet.Script.csproj
+++ b/src/Dotnet.Script/Dotnet.Script.csproj
@@ -1,42 +1,44 @@
-
-
- Dotnet CLI tool allowing you to run C# (CSX) scripts.
- 1.1.0
- filipw
- Dotnet.Script
- net5.0;netcoreapp2.1;netcoreapp3.1
- portable
- dotnet-script
- Exe
- dotnet;cli;script;csx;csharp;roslyn
- https://raw.githubusercontent.com/filipw/Strathweb.TypedRouting.AspNetCore/master/strathweb.png
- https://github.com/filipw/dotnet-script
- MIT
- git
- https://github.com/filipw/dotnet-script.git
- false
- false
- false
- false
- true
- latest
- true
- ../dotnet-script.snk
-
-
-
-
-
-
-
-
-
-
-
- Always
-
-
- Always
-
-
-
+
+
+
+ Dotnet CLI tool allowing you to run C# (CSX) scripts.
+ 1.6.0
+ filipw
+ Dotnet.Script
+ net9.0;net8.0
+ portable
+ dotnet-script
+ Exe
+ dotnet;cli;script;csx;csharp;roslyn
+ https://avatars.githubusercontent.com/u/113979420
+ https://github.com/dotnet-script/dotnet-script
+ MIT
+ git
+ https://github.com/dotnet-script/dotnet-script.git
+ false
+ false
+ false
+ false
+ true
+ latest
+ true
+ ../dotnet-script.snk
+
+
+
+
+
+
+
+
+
+
+
+
+ Always
+
+
+ Always
+
+
+
\ No newline at end of file
diff --git a/src/Dotnet.Script/LogHelper.cs b/src/Dotnet.Script/LogHelper.cs
index e523c09d..dd5de434 100644
--- a/src/Dotnet.Script/LogHelper.cs
+++ b/src/Dotnet.Script/LogHelper.cs
@@ -32,7 +32,7 @@ public static LogFactory CreateLogFactory(string verbosity)
internal class ConsoleOptionsMonitor : IOptionsMonitor
{
- private ConsoleLoggerOptions _consoleLoggerOptions;
+ private readonly ConsoleLoggerOptions _consoleLoggerOptions;
public ConsoleOptionsMonitor()
{
diff --git a/src/Dotnet.Script/Program.cs b/src/Dotnet.Script/Program.cs
index 54b2c196..06edc471 100644
--- a/src/Dotnet.Script/Program.cs
+++ b/src/Dotnet.Script/Program.cs
@@ -11,6 +11,7 @@
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
+using System.Runtime.Loader;
using System.Threading.Tasks;
namespace Dotnet.Script
@@ -54,8 +55,9 @@ public static Func CreateLogFactory
private static int Wain(string[] args)
{
- var app = new CommandLineApplication(throwOnUnexpectedArg: false)
+ var app = new CommandLineApplication()
{
+ UnrecognizedArgumentHandling = UnrecognizedArgumentHandling.CollectAndContinue,
ExtendedHelpText = "Starting without a path to a CSX file or a command, starts the REPL (interactive) mode."
};
@@ -66,10 +68,11 @@ private static int Wain(string[] args)
var debugMode = app.Option(DebugFlagShort + " | " + DebugFlagLong, "Enables debug output.", CommandOptionType.NoValue);
var verbosity = app.Option("--verbosity", " Set the verbosity level of the command. Allowed values are t[trace], d[ebug], i[nfo], w[arning], e[rror], and c[ritical].", CommandOptionType.SingleValue);
var nocache = app.Option("--no-cache", "Disable caching (Restore and Dll cache)", CommandOptionType.NoValue);
+ var isolatedLoadContext = app.Option("--isolated-load-context", "Use isolated assembly load context", CommandOptionType.NoValue);
var infoOption = app.Option("--info", "Displays environmental information", CommandOptionType.NoValue);
var argsBeforeDoubleHyphen = args.TakeWhile(a => a != "--").ToArray();
- var argsAfterDoubleHyphen = args.SkipWhile(a => a != "--").Skip(1).ToArray();
+ var argsAfterDoubleHyphen = args.SkipWhile(a => a != "--").Skip(1).ToArray();
const string helpOptionTemplate = "-? | -h | --help";
app.HelpOption(helpOptionTemplate);
@@ -81,7 +84,7 @@ private static int Wain(string[] args)
var code = c.Argument("code", "Code to execute.");
var cwd = c.Option("-cwd |--workingdirectory ", "Working directory for the code compiler. Defaults to current directory.", CommandOptionType.SingleValue);
c.HelpOption(helpOptionTemplate);
- c.OnExecute(async () =>
+ c.OnExecuteAsync(async (cancellationToken) =>
{
var source = code.Value;
if (string.IsNullOrWhiteSpace(source))
@@ -98,7 +101,7 @@ private static int Wain(string[] args)
}
var logFactory = CreateLogFactory(verbosity.Value(), debugMode.HasValue());
- var options = new ExecuteCodeCommandOptions(source, cwd.Value(), app.RemainingArguments.Concat(argsAfterDoubleHyphen).ToArray(),configuration.ValueEquals("release", StringComparison.OrdinalIgnoreCase) ? OptimizationLevel.Release : OptimizationLevel.Debug, nocache.HasValue(),packageSources.Values?.ToArray());
+ var options = new ExecuteCodeCommandOptions(source, cwd.Value(), app.RemainingArguments.Concat(argsAfterDoubleHyphen).ToArray(), configuration.ValueEquals("release", StringComparison.OrdinalIgnoreCase) ? OptimizationLevel.Release : OptimizationLevel.Debug, nocache.HasValue(), packageSources.Values?.ToArray());
return await new ExecuteCodeCommand(ScriptConsole.Default, logFactory).Execute(options);
});
});
@@ -185,7 +188,7 @@ private static int Wain(string[] args)
);
var logFactory = CreateLogFactory(verbosity.Value(), debugMode.HasValue());
- new PublishCommand(ScriptConsole.Default, logFactory).Execute(options);
+ new PublishCommand(ScriptConsole.Default, logFactory).Execute(options);
return 0;
});
});
@@ -196,7 +199,7 @@ private static int Wain(string[] args)
var dllPath = c.Argument("dll", "Path to DLL based script");
var commandDebugMode = c.Option(DebugFlagShort + " | " + DebugFlagLong, "Enables debug output.", CommandOptionType.NoValue);
c.HelpOption(helpOptionTemplate);
- c.OnExecute(async () =>
+ c.OnExecuteAsync(async (cancellationToken) =>
{
if (string.IsNullOrWhiteSpace(dllPath.Value))
{
@@ -215,7 +218,7 @@ private static int Wain(string[] args)
});
});
- app.OnExecute(async () =>
+ app.OnExecuteAsync(async (cancellationToken) =>
{
int exitCode = 0;
@@ -230,11 +233,15 @@ private static int Wain(string[] args)
return 0;
}
+ AssemblyLoadContext assemblyLoadContext = null;
+ if (isolatedLoadContext.HasValue())
+ assemblyLoadContext = new ScriptAssemblyLoadContext();
+
if (scriptFile.HasValue)
{
if (interactive.HasValue())
{
- return await RunInteractiveWithSeed(file.Value, logFactory, scriptArguments, packageSources.Values?.ToArray());
+ return await RunInteractiveWithSeed(file.Value, logFactory, scriptArguments, packageSources.Values?.ToArray(), assemblyLoadContext);
}
var fileCommandOptions = new ExecuteScriptCommandOptions
@@ -245,14 +252,21 @@ private static int Wain(string[] args)
packageSources.Values?.ToArray(),
interactive.HasValue(),
nocache.HasValue()
- );
+ )
+ {
+ AssemblyLoadContext = assemblyLoadContext
+ };
var fileCommand = new ExecuteScriptCommand(ScriptConsole.Default, logFactory);
- return await fileCommand.Run(fileCommandOptions);
- }
+ var result = await fileCommand.Run(fileCommandOptions);
+ if (Environment.ExitCode != 0) return Environment.ExitCode;
+
+ return result;
+
+ }
else
{
- await RunInteractive(!nocache.HasValue(), logFactory, packageSources.Values?.ToArray());
+ await RunInteractive(!nocache.HasValue(), logFactory, packageSources.Values?.ToArray(), assemblyLoadContext);
}
return exitCode;
});
@@ -260,16 +274,22 @@ private static int Wain(string[] args)
return app.Execute(argsBeforeDoubleHyphen);
}
- private static async Task RunInteractive(bool useRestoreCache, LogFactory logFactory, string[] packageSources)
+ private static async Task RunInteractive(bool useRestoreCache, LogFactory logFactory, string[] packageSources, AssemblyLoadContext assemblyLoadContext)
{
- var options = new ExecuteInteractiveCommandOptions(null, Array.Empty(), packageSources);
+ var options = new ExecuteInteractiveCommandOptions(null, Array.Empty(), packageSources)
+ {
+ AssemblyLoadContext = assemblyLoadContext
+ };
await new ExecuteInteractiveCommand(ScriptConsole.Default, logFactory).Execute(options);
return 0;
}
- private async static Task RunInteractiveWithSeed(string file, LogFactory logFactory, string[] arguments, string[] packageSources)
+ private async static Task RunInteractiveWithSeed(string file, LogFactory logFactory, string[] arguments, string[] packageSources, AssemblyLoadContext assemblyLoadContext)
{
- var options = new ExecuteInteractiveCommandOptions(new ScriptFile(file), arguments, packageSources);
+ var options = new ExecuteInteractiveCommandOptions(new ScriptFile(file), arguments, packageSources)
+ {
+ AssemblyLoadContext = assemblyLoadContext
+ };
await new ExecuteInteractiveCommand(ScriptConsole.Default, logFactory).Execute(options);
return 0;
}
diff --git a/src/omnisharp.json b/src/omnisharp.json
new file mode 100644
index 00000000..8d9f5671
--- /dev/null
+++ b/src/omnisharp.json
@@ -0,0 +1,9 @@
+{
+ "fileOptions": {
+ "systemExcludeSearchPatterns": [
+ "**/TestFixtures/**/*",
+ "**/ScriptPackages/**/*"
+ ],
+ "excludeSearchPatterns": []
+ }
+}
\ No newline at end of file