You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
#### Why should you have minimum scope for variables?
136
+
129
137
#### Why should you understand performance of String Concatenation?
138
+
130
139
#### What are the best practices with Exception Handling?
140
+
- Do not ignore exceptions
141
+
- When coding, think what will the guy debugging a problem here would need?
142
+
- Microservices - Centralized logging & correlation id
143
+
131
144
#### When is it recommended to prefer Unchecked Exceptions?
132
145
- Spring Framework
146
+
133
147
#### When do you use a Marker Interface?
148
+
134
149
#### Why are ENUMS important for Readable Code?
150
+
151
+
#### Avoid String when other types are appropriate
152
+
135
153
#### Why should you minimize mutability?
154
+
136
155
#### What is functional programming?
156
+
137
157
#### Why should you prefer Builder Pattern to build complex objects?
158
+
138
159
#### Why should you avoid floats for Calculations?
160
+
139
161
#### Why should you build the riskiest high priority features first?
140
162
141
163
### Code Quality
164
+
142
165
#### Code Quality Overview
143
166

144
167
- An overview : https://github.com/in28minutes/java-best-practices/blob/master/pdf/CodeQuality.pdf
@@ -196,7 +219,7 @@ I've been Programming, Designing and Architecting Java applications for 15 years
196
219
197
220
#### Why is performance of Unit Tests important?
198
221
199
-
#### Do not be fooled by Code Coverage?
222
+
#### Do not be fooled by Code Coverage!
200
223
201
224
#### Why should a good programmer understand Mocking?
202
225
@@ -247,6 +270,11 @@ I've been Programming, Designing and Architecting Java applications for 15 years
247
270
- Minimize Duplication
248
271
- Maximize Clarity
249
272
- Keep it Small
273
+
-- Code - Method, Class, jar etc
274
+
-- Component
275
+
-- Cycle Time (Short cycles)
276
+
-- Team Size
277
+
250
278
- Object Oriented Programming. Have good object, which have well-defined responsibilities. Following are the important concepts you need to have a good overview of. These are covered in various parts in the video https://www.youtube.com/watch?v=0xcgzUdTO5M. Also, look up the specific videos for each topic.
@@ -313,21 +341,162 @@ Listed below are some of the important considerations
313
341
- Do we need to expose external web services?
314
342
315
343
### Web Services
316
-
317
-
### SOAP Web Services
318
344

319
-
320
-
### REST Web Services
345
+
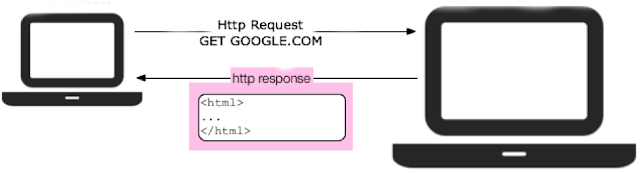
346
+
- Service Provider : Google.com is the service provider. Handles the request and sends a response back.
347
+
- Service Consumer : Browser is the service consumer. Creates Request. Invokes Service. Processes the Response.
348
+
- Data Exchange Format : In this example, Data Exchange is done over HTTP protocol. Request is HTTP request and Response is HTTP Response. Data exchange format can be something else as well. SOAP (in case of SOAP web services) and JSON (most RESTful services).
349
+
350
+
####Advantages
351
+
- Re-use : Web services avoid the need to implement business logic repeatedly. If we expose a web service, other applications can re-use the functionality
352
+
- Modularity : For example, tax calculation can be implemented as a service and all the applications that need this feature can invoke the tax calculation web service. Leads to very modular application architecture.
353
+
- Language Neutral : Web services enable communication between systems using different programming languages and different architectures. For example, following systems can talk with each other : Java, .Net, Mainframes etc.
354
+
- Web Services are the fundamental blocks of implementing Service Oriented Architecture in an organization.
355
+
356
+
#### SOAP Web Services
357
+
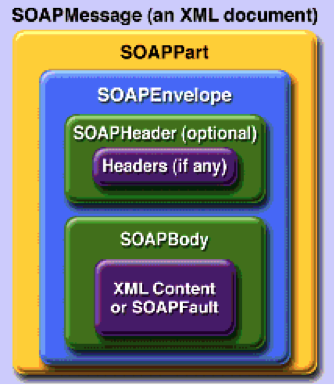
358
+
- In SOAP web services, data exchange (request and responses) happens using SOAP format. SOAP is based on XML.
359
+
- SOAP format defines a SOAP-Envelope which envelopes the entire document. SOAP-Header (optional) contains any information needed to identify the request. Also, part of the Header is authentication, authorization information (signatures, encrypted information etc). SOAP-Body contains the real xml content of request or response.
360
+
- All the SOAP web services use this format for exchanging requests and responses. In case of error response, server responds back with SOAP-Fault.
361
+
362
+
##### WSDL
363
+
WSDL defines the format for a SOAP Message exchange between the Server (Service Provider) and the Client (Service Consumer).
364
+
365
+
A WSDL defines the following:
366
+
- What are the different services (operations) exposed by the server?
367
+
- How can a service (operation) be called? What url to use? (also called End Point).
368
+
- What should the structure of request xml?
369
+
- What should be the structure of response xml?
370
+
371
+
##### Marshalling and Unmarshalling
372
+
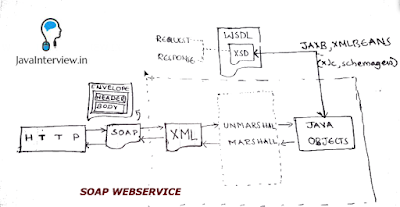
373
+
SOAP web services use SOAP based XML format for communication. Java applications work with beans i.e. java objects. For an application to expose or consume SOAP web services, we need two things
374
+
- Convert Java object to SOAP xml. This is called Marshalling.
375
+
- Convert SOAP xml to Java object. This is called Unmarshalling.
376
+
JAXB and XMLBeans are frameworks which enable use to do marshalling and unmarshalling easily.
377
+
378
+
##### Security
379
+
- At transport level, SSL is used to exchange certificates (HTTPS). This ensures that the server (service producer) and client (service consumer) are mutually authenticated. It is possible to use one way SSL authentication as well.
380
+
- At the application level, security is implemented by transferring encrypted information (digital signatures, for example) in the message header (SOAP Header). This helps the server to authenticate the client and be confident that the message has not been tampered with.
381
+
382
+
#### REST Web Services
321
383
- PDF TO UPDATE https://www.mindmup.com/#m:g10B8KENIDghuHAYmFzM0daOU80SDA
322
-
323
-
#### How should you document your REST Web Services?
324
-
- Swagger
384
+
- There are a set of architectural constraints (we will discuss them shortly) called Rest Style Constraints. Any service which satisfies these constraints is called RESTful Web Service.
385
+
- There are a lot of misconceptions about REST Web Services : They are over HTTP , based on JSON etc. Yes : More than 90% of RESTful Web Services are JSON over HTTP. But these are not necessary constraints. We can have RESTful Web Services which are not using JSON and which are not over HTTP.
386
+
387
+
##### Constraints
388
+
The five important constraints for RESTful Web Service are
389
+
- Client - Server : There should be a service producer and a service consumer.
390
+
- The interface (URL) is uniform and exposing resources. Interface uses nouns (not actions)
391
+
- The service is stateless. Even if the service is called 10 times, the result must be the same.
392
+
- The service result should be Cacheable. HTTP cache, for example.
393
+
- Service should assume a Layered architecture. Client should not assume direct connection to server - it might be getting info from a middle layer - cache.
394
+
395
+
##### Richardson Maturity Model
396
+
Richardson Maturity Model defines the maturity level of a Restful Web Service. Following are the different levels and their characteristics.
397
+
- Level 0 : Expose SOAP web services in REST style. Expose action based services (http://server/getPosts, http://server/deletePosts, http://server/doThis, http://server/doThat etc) using REST.
398
+
- Level 1 : Expose Resources with proper URI’s (using nouns). Ex: http://server/accounts, http://server/accounts/10. However, HTTP Methods are not used.
399
+
- Level 2 : Resources use proper URI's + HTTP Methods. For example, to update an account, you do a PUT to . The create an account, you do a POST to . Uri’s look like posts/1/comments/5 and accounts/1/friends/1.
400
+
- Level 3 : HATEOAS (Hypermedia as the engine of application state). You will tell not only about the information being requested but also about the next possible actions that the service consumer can do. When requesting information about a facebook user, a REST service can return user details along with information about how to get his recent posts, how to get his recent comments and how to retrieve his friend’s list.
401
+
402
+
##### RESTful API Best Practices
403
+
- Needs more work
404
+
- While designing any API, the most important thing is to think about the api consumer i.e. the client who is going to use the service. What are his needs? Does the service uri make sense to him? Does the request, response format make sense to him?
405
+
- URI’s should be hierarchical and as self descriptive as possible. Prefer plurals.
406
+
- Always use HTTP Methods. Best practices with respect to each HTTP method is described below:
407
+
-- GET : Should not update anything. Should be idempotent (same result in multiple calls). Possible Return Codes 200 (OK) + 404 (NOT FOUND) +400 (BAD REQUEST)
408
+
-- POST : Should create new resource. Ideally return JSON with link to newly created resource. Same return codes as get possible. In addition : Return code 201 (CREATED) is possible.
409
+
-- PUT : Update a known resource. ex: update client details. Possible Return Codes : 200(OK)
410
+
-- DELETE : Used to delete a resource.
411
+
412
+
##### JAX-RS
413
+
JAX-RS is the JEE Specification for Restful web services implemented by all JEE compliant web servers (and application servers).
414
+
Important Annotations
415
+
-@ApplicationPath("/"). @Path("users") : used on class and methods to define the url path.
416
+
-@GET@POST : Used to define the HTTP method that invokes the method.
417
+
-@Produces(MediaType.APPLICATION_JSON) : Defines the output format of Restful service.
418
+
-@Path("/{id}") on method (and) @PathParam("id") on method parameter : This helps in defining a dynamic parameter in Rest URL. @Path("{user_id}/followers/{follower_id}") is a more complicated example.
419
+
-@QueryParam("page") : To define a method parameter ex: /users?page=10.
420
+
421
+
Useful methods:
422
+
- Response.OK(jsonBuilder.build()).build() returns json response with status code.
423
+
- Json.createObjectBuilder(). add("id",user.getId()); creates a user object.
424
+
425
+
##### How should you document your REST Web Services?
- Challenges with Monolith Applications - Longer Release Cycles because of Large Size, Large Teams and difficulty in adopting Automation testing and modern development practices
432
+
- “Keep it Small”. Small deployable components.
433
+
- Flights
434
+
-- Points
435
+
-- Offers
436
+
-- Trips
437
+
- Customer
438
+
-- Product
439
+
-- Order
440
+
-- Recommendations
441
+
- Key question to ask : Can we make a change to a service and deploy it by itself without changing anything else?
442
+
443
+
#### Microservices Characteristics
444
+
- Small, Lightweight
445
+
- Loosely coupled service-oriented architectures with bounded contexts
446
+
- Bounded Scope, Limited Scope, Solve one problem well
447
+
- Interoperable with message based communication
448
+
- Independently deployable and testable services
449
+
- Building systems that are replaceable over being maintainable
450
+
- “Smart endpoints and dump pipes”
451
+
452
+
#### Advantages
453
+
- Faster Time To Market
454
+
- Complete Automation Possibility
455
+
- Experimentation
456
+
- Technology Evolution
457
+
- Speed of innovation
458
+
- Team Autonomy : Enables creation of independent teams with End-to-End ownership
459
+
- Deployment Simplicity
460
+
- Flexible Scaling
461
+
462
+
#### Challenges
463
+
- Visibility
464
+
- Standardization
465
+
- Operations Team
466
+
- Determining Boundaries
467
+
468
+
469
+
#### What is the difference between Microservices and SOA?
470
+
Microservices have similar goals from SOA : Create services around your business logic.
471
+
472
+
Key Differences
473
+
- Big vendors hijacked SOA to link SOA with products like Enterprise Services Bus (Websphere ESB, Oracle ESB, TIBCO Business Works) etc
474
+
- SOA was tied to XML and its formalities and complexities
475
+
- SOA had centralized governance whereas microservice architecture recommends a decentralized governance.
476
+
- SOA did not focus on the independent deployability of the components.
477
+
- ESB was brought in to enable loose coupling for SOA based systems. The complexity with the ESBs etc in SOA lead to coining the term “dumb pipes smart endpoints”
478
+
Example:
479
+

480
+
- Consider a banking application selling multiple products
481
+
- Along with Saving Account, a customer gets a Debit Card Free and a Insurance
482
+
Saving Account and Debit Card are different products managed by different product systems
483
+
- In SOA Architecture, the ESB took the order request from the sales application and handled the communication with creating the appropriate products. ESB ends up having a lot of logic. Heavy weight ESB.
484
+
- Microservices use more of an event driven architecture!
327
485
328
486
#### What are Cloud Native Applications?
329
-
330
-
#### What are micro-services?
487
+
- 12 Factor App
488
+
- Codebase - One codebase tracked in revision control, many deploys
489
+
- Dependencies - Explicitly declare and isolate dependencies
490
+
- Config - Store config in the environment
491
+
- Backing services - Treat backing services as attached resources
492
+
- Build, release, run - Strictly separate build and run stages
493
+
- Processes - Execute the app as one or more stateless processes
494
+
- Port binding - Export services via port binding
495
+
- Concurrency - Scale out via the process model
496
+
- Disposability - Maximize robustness with fast startup and graceful shutdown
497
+
- Dev/prod parity - Keep development, staging, and production as similar as possible
498
+
- Logs - Treat logs as event streams
499
+
- Admin processes - Run admin/management tasks as one-off processes
331
500
332
501
#### Why is it important to have an API Standard?
333
502
- YARAS
@@ -411,6 +580,7 @@ Most important qualities I look for in an Architect are
411
580
- In critical parts - write unit tests for performance.
412
581
- Be conscious about create as few objects as possible. Especially in loops.
0 commit comments